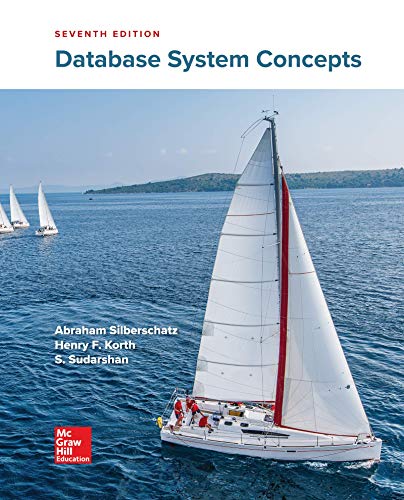
Public class DLL{
public DLLNode first;
public DLLNode last;
public DLL()
{this.first=Null;
this.last=Null;}
Public void Display()
{if (first==Null)
{s.o.p(“ the list is empty”);}
else
{for (DLLNode curr=first; curr!=Null; curr=curr.succ)
{System.out.println(“the element is”+ curr.element);
}}
Public void AddBegining(DLLNode Node)
{Node.succ=first;
First.prev=Node
First=Node;
Node.prev=Null;
if(first ==null && last==null)
Last=node;
}
Public void Append(DLLNode Node)
{Node.succ=Null;
if(first==Null)
{first=Node;
Node.prev=Null}
Public void insetAfter(int obj, DLLNode Node)
{DLLNode curr=first;
while((curr!=Null)&&( curr.element!=obj))
{ curr=curr.succ; }
If(curr.element==obj)
{Node.succ=curr.succ;
Curr.succ.prev=Node;
Curr.succ=Node;
Node.prev=curr;}
Else
System.out.println(“the element doesn’t exist”);
Public void delete(DLLNode del)
{
if(first==Null)
{System.out.println(“the list is empty”); }
Else if(del==first)
{first==del.succ;}
Else if(del.succ==Null)
first=Null;
last=Null;
Else
{DLLNode ptr= first;
while(ptr.succ!=del)
{Ptr=ptr.succ;}
Public class DLLNode{
int element;
DLLNode prev;
DLLNode succ;
Public DLLNode(int E)
{this. Element=E;
This. prev=Null;
This.succ=Null}
Public static void main(String args[])
{DLL list = new DLL();
Scanner s = new Scanner(System.in);
Int X,Y,Size;
DLLNode Node1= new DLLNode(5);
DLLNode Node2= new DLLNode(6);
DLLNode Node3= new DLLNode(7);
DLLNode Node4= new DLLNode(9);
list.AddBegining(Node1);
list.Append(Node2);
list.Append(Node3);
list.Append(Node4);
list.Display();
1. Count the number of nodes
2. Insert a new node before the value 7 of Double Linked List
3. Search an existing element in a Double linked list (the element of search
is given by the user)
Suppose List contained the following Test Data: Input the number of nodes : 4
Input data for node 1 : 5
Input data for node 2 : 6
Input data for node 3 : 7 Input data for node 4: 9

Step by stepSolved in 4 steps with 5 images

- A class object can encapsulate more than one [answer].arrow_forwardpublic class LLNode{ private Name name; private LLNode next; public LLNode(){ name=null; next=null; } public LLNode(Name name, LLNode next){ setName(name); setNext(next); } public Name getName(){ return name; } public LLNode getNext(){ return next; } public void setName(Name name){ this.name = name; } public void setNext(LLNode next){ this.next = next; } } ******************************************************************public class Name implements Comparable<Name>{ private String firstName; private String lastName; public Name(String firstName, String lastName){ setFirstName(firstName); setLastName(lastName); } public String getFirstName(){ return firstName; } public String getLastName(){ return lastName; } public void setFirstName(String firstName){ this.firstName = firstName; } public void setLastName(String lastName){ this.lastName = lastName;…arrow_forwardCreate a fee invoice application for students attending Valence College, a college in the State of Florida. There are two types of students: graduate and undergraduate. Out-of-state undergraduate students pay twice the tuition of Florida-resident undergraduate students (all students pay the same health and id fees). A graduate student is either a PhD or a MS student. PhD students don't take any courses, but each has an advisor and a research subject. Each Ms and Phd student must be a teaching assistant for one undergraduate course. MS students can only take graduate courses. A course with a Course Number (crn) less than 5000 is considered an undergraduate course, and courses with a 5000 crn or higher are graduate courses. CRN Course Credit Hours. 4587 MAT 236 4 4599 COP 220 3 8997 GOL 124 1 9696 COP 100 3 4580 MAT 136 1 2599 COP 260 3 1997 CAP 424 1 5696 KOL 110 2 7587 MAT 936 5 2599 COP 111 3 6997 GOL 109 1 2696 COP 101 3 5580 MAT 636 2 2099 COP 268 3 4997 CAP 427 1 3696 KOL 910 2…arrow_forward
- public class Plant { protected String plantName; protected String plantCost; public void setPlantName(String userPlantName) { plantName = userPlantName; } public String getPlantName() { return plantName; } public void setPlantCost(String userPlantCost) { plantCost = userPlantCost; } public String getPlantCost() { return plantCost; } public void printInfo() { System.out.println(" Plant name: " + plantName); System.out.println(" Cost: " + plantCost); }} public class Flower extends Plant { private boolean isAnnual; private String colorOfFlowers; public void setPlantType(boolean userIsAnnual) { isAnnual = userIsAnnual; } public boolean getPlantType(){ return isAnnual; } public void setColorOfFlowers(String userColorOfFlowers) { colorOfFlowers = userColorOfFlowers; } public String getColorOfFlowers(){ return colorOfFlowers; } @Override public void printInfo(){…arrow_forwardQuestion 13 What is outpout? public class Vehicle { public void drive(){ System.out.println("Driving vehicle"); } } public class Plane extends Vehicle { @Override public void drive(){ System.out.println("Flying plane"); } public static void main(String args[]) { Vehicle myVehicle= = new Plane(); myVehicle.drive(); } Driving vehicle syntax error Flying plane Driving vehicle Flying plane }arrow_forwardCreate a fee invoice application for students attending Valence College, a college in the State of Florida. There are two types of students: graduate and undergraduate. Out-of-state undergraduate students pay twice the tuition of Florida-resident undergraduate students (all students pay the same health and id fees). A graduate student is either a PhD or a MS student. PhD students don't take any courses, but each has an advisor and a research subject. Each Ms and Phd student must be a teaching assistant for one undergraduate course. MS students can only take graduate courses. A course with a Course Number (crn) less than 5000 is considered an undergraduate course, and courses with a 5000 crn or higher are graduate courses. CRN Course Credit Hours. 4587 MAT 236 4 4599 COP 220 3 8997 GOL 124 1 9696 COP 100 3 4580 MAT 136 1 2599 COP 260 3 1997 CAP 424 1 5696 KOL 110 2 7587 MAT 936 5 2599 COP 111 3 6997 GOL 109 1 2696 COP 101 3 5580 MAT 636 2 2099 COP 268 3 4997 CAP 427 1 3696 KOL 910 2…arrow_forward
- interface StudentsADT{void admissions();void discharge();void transfers(); }public class Course{String cname;int cno;int credits;public Course(){System.out.println("\nDEFAULT constructor called");}public Course(String c){System.out.println("\noverloaded constructor called");cname=c;}public Course(Course ch){System.out.println("\nCopy constructor called");cname=ch;}void setCourseName(String ch){cname=ch;System.out.println("\n"+cname);}void setSelectionNumber(int cno1){cno=cno1;System.out.println("\n"+cno);}void setNumberOfCredits(int cdit){credits=cdit;System.out.println("\n"+credits);}void setLink(){System.out.println("\nset link");}String getCourseName(){System.out.println("\n"+cname);}int getSelectionNumber(){System.out.println("\n"+cno);}int getNumberOfCredits(){System.out.println("\n"+credits); }void getLink(){System.out.println("\ninside get link");}} public class Students{String sname;int cno;int credits;int maxno;public Students(){System.out.println("\nDEFAULT constructor…arrow_forwardQuestion 6 Consider: class Bike { } private String color; public Bike() { } public Bike(String newColor) { color = newColor; } public Bike (Bike bike) { color = } bike.color; public String getColor() { } } return color; public void setColor(String newColor) { color = newColor; Bike bike1= new Bike(); = Bike bike2 new Bike("blue"); Bike bike3 = new Bike(bike2); bike2.setColor("green"); Bike bike4 = new Bike(bike2); Which of the following are correct? bike4.getColor() returns null bike4.getColor() return "blue" bike3.getColor() return "green" bike2.getColor() returns "blue" bike4.getColor() return "green" bike1.getColor() returns bike3.getColor() return null bike3.getColor() returns "blue" bike2.getColor() returns "green" bike1.getColor() returns nullarrow_forward// This class discounts prices by 10% public class DebugFour4 { public static void main(String args[]) { final double DISCOUNT_RATE = 0.90; int price = 100; double price2 = 100.00; tenPercentOff(price DISCOUNT_RATE); tenPercentOff(price2 DISCOUNT_RATE); } public static void tenPercentOff(int p, final double DISCOUNT_RATE) { double newPrice = p * DISCOUNT_RATE; System.out.println("Ten percent off " + p); System.out.println(" New price is " + newPrice); } public static void tenPercentOff(double p, final double DISCOUNT_RATE) { double newPrice = p * DISCOUNT_RATE; System.out.println"Ten percent off " + p); System.out.println(" New price is " + newprice); } }arrow_forward
- public class Cat extends Pet { private String breed; public void setBreed(String userBreed) { breed = userBreed; } public String getBreed() { return breed; }} public class Pet { protected String name; protected int age; public void setName(String userName) { name = userName; } public String getName() { return name; } public void setAge(int userAge) { age = userAge; } public int getAge() { return age; } public void printInfo() { System.out.println("Pet Information: "); System.out.println(" Name: " + name); System.out.println(" Age: " + age); } } import java.util.Scanner; public class PetInformation { public static void main(String[] args) { Scanner scnr = new Scanner(System.in); // create a generic Pet and a Cat Pet myPet = new Pet(); Cat myCat = new Cat(); // declare variables for pet and cat info String petName, catName, catBreed; int petAge,…arrow_forwardclass IndexItem { public: virtual int count() = 0; virtual void display()= 0; };class Book : public IndexItem { private: string title; string author; public: Book(string title, string author): title(title), author(author){} virtual int count(){ return 1; } virtual void display(){ /* YOU DO NOT NEED TO IMPLEMENT THIS FUNCTION */ } };class Category: public IndexItem { private: /* fill in the private member variables for the Category class below */ ? int count; public: Category(string name, string code): name(name), code(code){} /* Implement the count function below. Consider the use of the function as depicted in main() */ ? /* Implement the add function which fills the category with contents below. Consider the use of the function as depicted in main() */ ? virtualvoiddisplay(){ /* YOU DO NOT NEED TO IMPLEMENT THIS FUNCTION */ } };arrow_forwardthis is my retail item code: //Import statements public class RetailItem { private String description; private int units; private double price; public RetailItem() { } public RetailItem(String x, int y, double z) { description = x; units = y; price = z; } public RetailItem(RetailItem i) { } public void setDescription(String x) { description = x; } public void setPrice(double z) { price = z; } void setUnits(int y) { units = y; } public int getUnits() { return units; } public String getDescription() { return description; } public double getPrice() { return price; } // Main class public static void main(String[] args) { String str = "Shirt"; RetailItem r1 = new RetailItem("Jacket", 12,…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
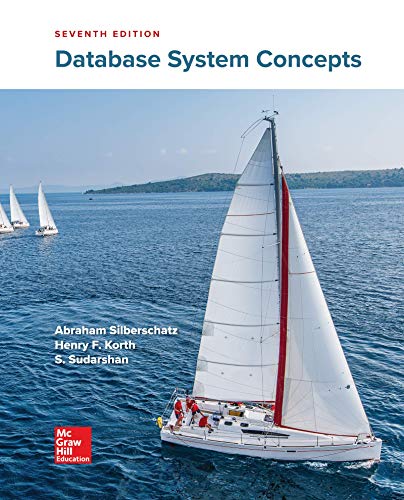
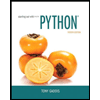
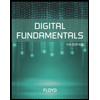
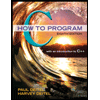
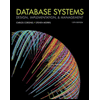
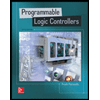