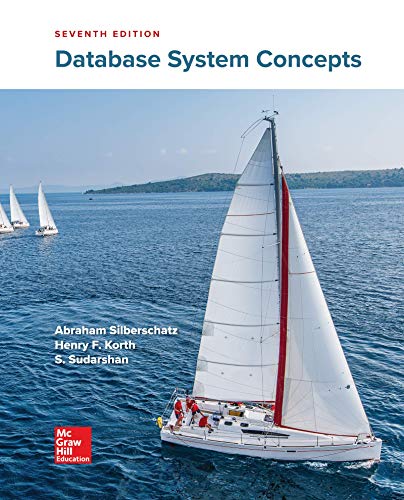
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Please help, I only need help where it is marked "TODO**", because I don't understand what to fill in
// PURPOSE: Removes vertex pointed to by V
// PARAM: V and its parent pointer P
// Case 1: it is a leaf, delete it
// Case 2: it has just one child, bypass it
// Case 3: it has two children, replace it with the max of the left subtree
void BST::remove(Vertex *V, Vertex *P)
{
if (V->left == NULL && V->right == NULL) //TODO (if)
{ // if V is a leaf (case 1)
cout << "removing a leaf" << endl;
if (P->left == V)
{ // TODO if V is a left child of P
P->left = NULL;
delete V;
// TODO call here from P to adjust height and BF
}
else
{ // TODO V is a right child of the Parent
P->right = NULL;
delete V; // TODO call from P to adjust height and BF
}
}
else if (V->left != NULL && V->right == NULL) //TODO (if)
{ // if V has just the left child so bypass V (case 2)
Vertex *C = V->left; // C is the left child
cout << "removing a vertex with just the left child" << endl;
// TODO You need if then else to determine Parent's left or right
// should point to C;
// TODO Make C point UP to the parent;
if (P->left == V)
P->left = C;
else
P->right = C;
C->up = P;
cout << C->elem << " points up to " << C->up->elem << endl;
// TODO Be sure to delete V
delete V;
// TODO** call from P to adjust height and BF
}
else if (V->left == NULL && V->right != NULL)
{ // if V has just the right child so bypass V (case 2)
Vertex *C = V->right; // C is the right child
cout << "removing a vertex with just the right child" << endl;
if (P->left == V)
P->left = C;
else
P->right = C;
C->up = P;
cout << C->elem << " points up to " << C->up->elem << endl;
delete V;
// TODO** call from P to adjust height and BF
}
else
{ // V has two children (case 3)
cout << "removing an internal vertex with children" << endl;
cout << "..find the MAX of its left sub-tree" << endl;
el_t Melem;
// find MAX element in the left sub-tree of V
Melem = findMax(V);
cout << "..replacing " << V->elem << " with " << Melem << endl;
V->elem = Melem; // TODO Replace V's element with Melem here
}
} // end of remove
SAVE
AI-Generated Solution
info
AI-generated content may present inaccurate or offensive content that does not represent bartleby’s views.
Unlock instant AI solutions
Tap the button
to generate a solution
to generate a solution
Click the button to generate
a solution
a solution
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Help in C++ please: Write a program (in main.cpp) that: Prompts the user for a filename containing node data. Outputs the minimal spanning tree for a given graph. You will need to implement the createSpanningGraph method in minimalSpanTreeType.h to create the graph and the weight matrix. There are a few tabs: main.cpp, graphType.h, linkedList.h, linkedQueue.h, queueADT.h, minimalSpanTreeType.h, and then two data files labeled: CH20_Ex21Data.txt, CH20Ex4Data.txtarrow_forwardclass Graph: def __init__(self, num_nodes): self.num_nodes = num_nodes # Initialize a 2D matrix with zeros self.adj_matrix = [[0] * num_nodes for _ in range(num_nodes)] def add_edge(self, node1, node2): # Add an undirected edge between node1 and node2 self.adj_matrix[node1][node2] = 1 self.adj_matrix[node2][node1] = 1 def remove_edge(self, node1, node2): # Remove the edge between node1 and node2 self.adj_matrix[node1][node2] = 0 self.adj_matrix[node2][node1] = 0 def num_edges(self): num_edges = 1 for i in range(self.num_nodes): for j in range(i+1, self.num_nodes): if self.adj_matrix[i][j] == 1: num_edges += 1 return num_edges def num_edges_in_set(self, node, subset): # count the number of edges joining the given node and nodes in subset. # example input: 1, {0, 2} num_edges = 0 for s in subset: if…arrow_forwardCan you help me with a C++ programming task I am trying to complete for myself please: Write a program (in main.cpp) that: Prompts the user for a filename containing node data. Outputs the minimal spanning tree for a given graph. You will need to implement the createSpanningGraph method in minimalSpanTreeType.h to create the graph and the weight matrix. There are a few tabs: main.cpp, graphType.h, linkedList.h, linkedQueue.h, queueADT.h, minimalSpanTreeType.h, and then two data files labeled: CH20_Ex21Data.txt, CH20Ex4Data.txtarrow_forward
- Graphs: Depth First Traversal Starting with the same graph program as last assignment, implement a depth first traversal method. Test iy on nodes 1, 2, and 3 as start nodes. Graph program: #include <iostream>#include <vector>#include <string>using namespace std; class Edge;//-------------------------------------------------------------////class Node{public:Node(string iname){name = iname;}string name;int in_count = 0;bool visited = false; vector<Edge *> out_edge_list;};//-------------------------------------------------------------////class Edge{public:Edge(string iname, double iweight, Node *ifrom, Node *ito){name = iname;weight = iweight;from = ifrom;to = ito;} string name;double weight;Node *from;Node *to;bool visited = false;}; //-------------------------------------------------------------////class Graph{public:vector<Node *> node_list;vector<Edge *> edge_list; //----------------------------------------------------------//Node*…arrow_forwardIn a doubly linked list the first class is Node which we can create a new node with a given element. Its constructor also includes previous node reference prev and next node reference next. make a node object for the new element. Check if the index >= 0.If index is 0, make new node as head; else, make a temp node and iterate to the node previous to the index.If the previous node is not null, adjust the prev and next references. Print a message when the previous node is null. implement these 3 methods: insert_at_index(), delete_at_end(), display(). use these three functions above use this to name the items 1. insert_to_empty_list()2. insert_to_end()3. insert_at_index() 4. delete_at_start() 5. delete_at_end() . 6. display() let the output be OUTPUT: The list is emptyElement is: 3Element is: 10Element is: 20Element is: 30Element is: 35Element is: 38Element is: 40Element is: 50Element is: 60 Element is: 10Element is: 20Element is: 30Element is: 35Element is: 38Element is: 40Element…arrow_forwardGiven the following definition for a LinkedList: // LinkedList.h class LinkedList { public: LinkedList(); // TODO: Implement me void printEveryOther() const; private: struct Node { int data; Node* next; }; Node * head; }; // LinkedList.cpp #include "LinkedList.h" LinkedList::LinkedList() { head = nullptr; } Implement the function printEveryOther, which prints every other data value (i.e. those at the odd indices assuming 0-based indexing).arrow_forward
- not sure how to do this problem output doesnt matterarrow_forwardTranposeGraph import java.io.*; import java.util.*; // This class represents a directed graph using adjacency list // representation class ALGraph{ private int vertices; // No. of vertices // Array of lists for Adjacency List Representation private LinkedList adj[]; // Constructor ALGraph(int vertices) { this.vertices = vertices; adj = new LinkedList[this.vertices]; for (int i=0; i getAdjacentList(int v) { return adj[v]; } //Function to add an edge into the graph void addEdge(int v, int w) { adj[v].add(w); // Add w to v's list. } // TODO Transpose graph // If the graph includes zero vertices, return null // Create a new ALGraph // For every vertex, retrieve its adjacent list, make a pass over the list and rewrite each edge (u, v) to be (v, u) and add the u into the adjacent list of v public ALGraph transpose(){…arrow_forwardComplete the //comments and TODOs in c++ #include "LinkedList.h" using namespace std; // Add a new node to the list void LinkedList::insert(Node* prev, int newKey){ //Check if head is Null i.e list is empty if(head == NULL){ head = newNode; head->key = newKey; head->next = NULL; } // if list is not empty, look for prev and append our node there elseif(prev == NULL) { Node* newNode = newNode; newNode->key = newKey; newNode->next = head; head = newNode; } else{ Node* newNode = newNode; newNode->key = newKey; newNode->next = prev->next; prev->next = newNode; } } // TODO: SILVER PROBLEM // Delete node at a particular index bool LinkedList::deleteAtIndex(int n) { boolisDeleted = false; if(head == NULL){ cout<<"List is already empty"<<endl; returnisDeleted; } // Special case to delete the head if (n == 0) { //TODO } Node *pres = head; Node *prev = NULL; // TODO returnisDeleted; } // TODO: GOLD PROBLEM // Swap the first and last nodes (don't…arrow_forward
- Send me your own work not AI generated response or plagiarised response. If I see these things, I'll give multiple downvotes and will definitely report.arrow_forwardI could use help, me please: Write a program (in main.cpp) that: Prompts the user for a filename containing node data. Outputs the minimal spanning tree for a given graph. You will need to implement the createSpanningGraph method in minimalSpanTreeType.h to create the graph and the weight matrix. Note: Files Ch20_Ex21Data.txt and Ch20_Ex4Data.txt contain node data that you may test your program with. minimalSpanTreeType.h: #ifndef H_queueADT #define H_queueADT template <class Type> class queueADT { public: virtual bool isEmptyQueue() const = 0; //Function to determine whether the queue is empty. //Postcondition: Returns true if the queue is empty, // otherwise returns false. virtual bool isFullQueue() const = 0; //Function to determine whether the queue is full. //Postcondition: Returns true if the queue is full, // otherwise returns false. virtual void initializeQueue() = 0; //Function…arrow_forwardJava language the top half is the class that contains the linked list parts the bottom half is the method i need help setting up should take two parameters "index" and "element" should be an actual method not just LinkedList.set(index, element); please and thank you!arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
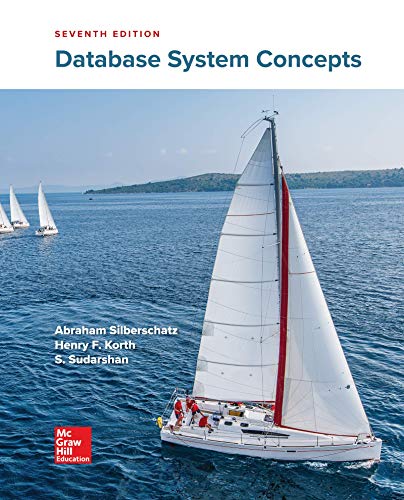
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
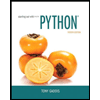
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
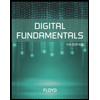
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
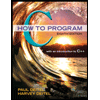
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
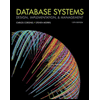
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
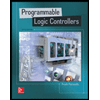
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education