What is a linked list?
A sequence of data elements connected through links is called a linked list (LL). The elements of a linked list are nodes containing data and a reference to the next node in the list. In a linked list, the elements are stored in a non-contiguous manner and the linear order in maintained by means of a pointer associated with each node in the list which is used to point to the subsequent node in the list.
Each node in the linked list consists of two components namely data which represents the value stored and a reference to the next node containing the next element of the list.
Types of linked list
- Singly linked list (holds two parts).
- Doubly linked list (holds three parts).
- Circular linked list.
- Doubly circular linked list.
Singly linked list
This is a widely used linked list. Each node consists of two parts, the data part and the address part. Address part stores the address of the next node. Consider four elements 4, 6, 8 and 2 which are stored at locations 100, 200, 300 and 400 respectively. The primary/first node stores the address of the second node (200), the second node stores the address of the third node (300), the third node stores the address of the last node. The last node stores the null value because it does not point to any node. The pointer that stores the address of the first node is termed as the head pointer.
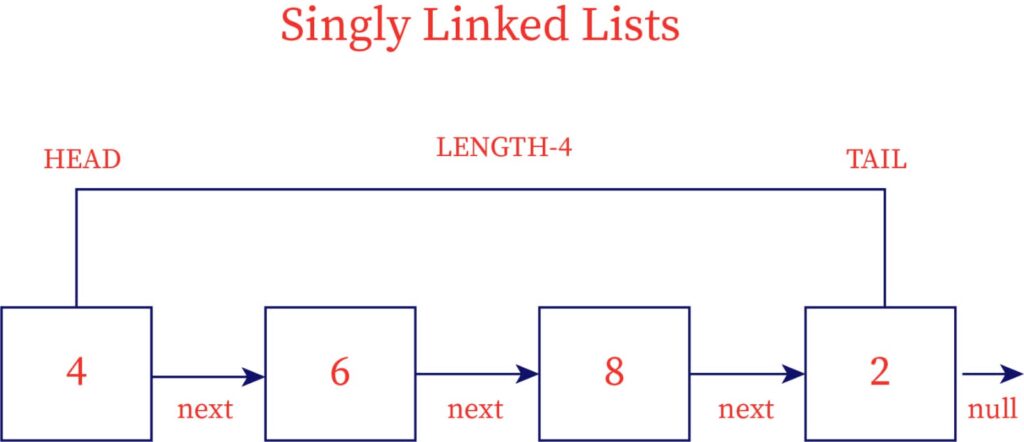
Declaration of node in a singly linked list
struct new node //user-defined structure
{
int data; //integer data value
struct node *next; //initiating successor node
}
The above code declares a user-defined structure node that stores data of type integer and a pointer to the next node.
Doubly linked list
A doubly linked list is similar to singly linked list except that each node has two pointers associated with it. One points to the previous node in the list and the other one points to the next node in the list. The first node's prev pointer equals null and the last node's next pointer equals null.
Declaration of a node in a doubly linked list
struct node //user-defined structure
{
int data; //integer data value
struct node *next;
struct node *prev;
}
The above code declares a user-defined structure node with three variables, an integer value (data), and two pointers (next and prev with node type). The pointer prev stores the previous node’s address and the next pointer stores the address of the succeeding/next node.
Circular linked list
Circular linked list is a variant of singly linked list. The sole distinction between a singly linked list and a circular linked list is that the next pointer of the last node points back to the first node. There will be no ending and starting node within the circular linked list.
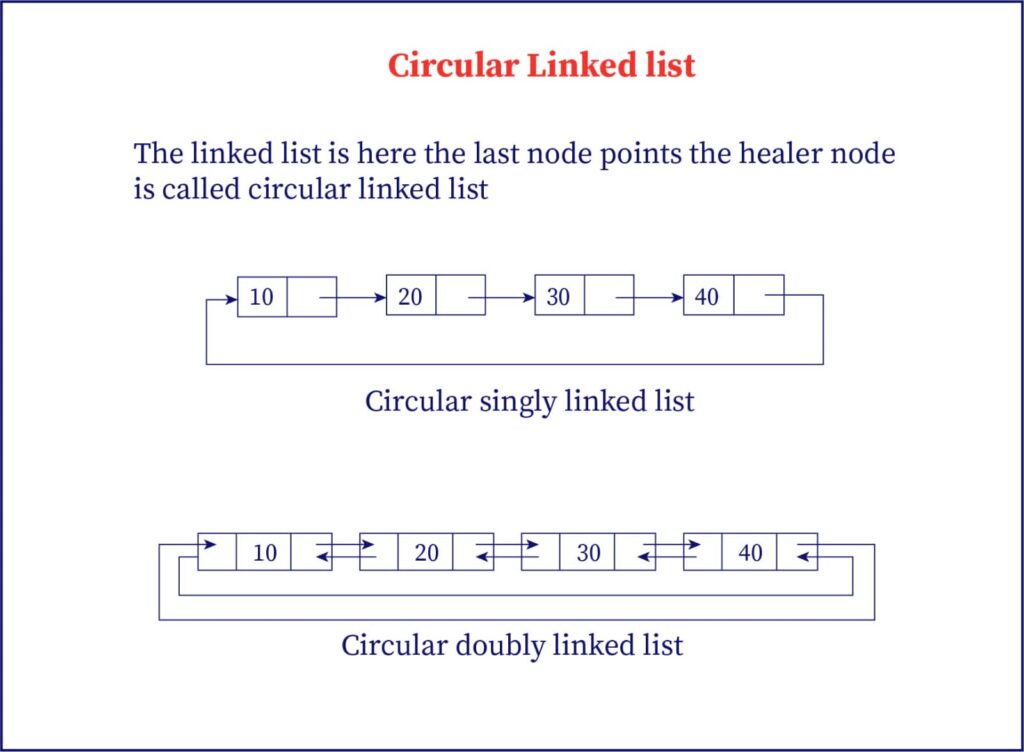
Doubly circular linked list
It is a combination of doubly linked list and circular linked list. It is similar to circular linked list since the last node's next pointer references the first node. It is a doubly linked list in which each node has two pointers which point to the preceding and succeeding nodes respectively. Therefore, the first node's previous pointer points to the last node and the last node's next pointer points to the first node. The list can be traversed in both forward and backward directions easily.
Basic operations
- Traversal- visiting the nodes sequentially.
- Insert- adding a new node at any position.
- Delete- removing a node from any position.
- Search- finding a node by value.
- Update- changing the data value of a node.
- Sort- arranging the nodes in a particular order.
- Merge- combining two linked lists into one.
Advantages
- Dynamic data structure.
- Memory is utilized very efficiently due to non-contiguous storage.
- Deletion and insertion operations are simple to perform.
Disadvantages
- Requires extra memory to store both the data and references.
- Difficulty in traversing the nodes.
- Does not allow random/direct access to a node.
Context and Applications
This topic is important for postgraduate and undergraduate courses, particularly for,
- Bachelors in computer science engineering.
- Associate of science in computer science.
Practice problems
Question 1: What is the name of a linear collection of data pieces which are stored in non-contiguous manner but still linear order is maintained by means of a pointer?
- linked list
- list of nodes
- list of primitives
- None of these
Answer: Option 1 is correct.
Explanation: A linked list allows list elements to be stored non-contiguously but linear order is maintained by means of pointers.
Question 2: ____ is the space complexity to delete an element from the linked list.
- O(n^2)
- O(1)
- O(logn)
- O(n)
Answer: Option 2 is correct.
Explanation: It takes constant time to delete an element from the list.
Question 3: Pointer pointing to the first element of the list is called________
- top
- first
- head
- None of the above
Answer: Option 3 is correct.
Explanation: Head pointer stores the address of the first element of the list.
Question 4: What is value of next pointer of last element in a circular linked list?
- Address of previous node.
- Address of first node.
- Address of itself.
- null.
Answer: Option 2 is correct.
Explanation: In a circular linked list, last node stores the address of first node.
Question 5: Elements in a linked list are stored in a contiguous manner.
- True
- False
Answer: Option 2 is correct.
Explanation: Elements are stored non-contiguously. However, linear ordering is maintained by means of pointers.
Want more help with your computer science homework?
*Response times may vary by subject and question complexity. Median response time is 34 minutes for paid subscribers and may be longer for promotional offers.
Search. Solve. Succeed!
Study smarter access to millions of step-by step textbook solutions, our Q&A library, and AI powered Math Solver. Plus, you get 30 questions to ask an expert each month.
Types of Linked List Homework Questions from Fellow Students
Browse our recently answered Types of Linked List homework questions.
Search. Solve. Succeed!
Study smarter access to millions of step-by step textbook solutions, our Q&A library, and AI powered Math Solver. Plus, you get 30 questions to ask an expert each month.