Write a program (in main.cpp) that: Prompts the user for a filename containing node data. Outputs the minimal spanning tree for a given graph. You will need to implement the createSpanningGraph method in minimalSpanTreeType.h to create the graph and the weight matrix. Note: Files Ch20_Ex21Data.txt and Ch20_Ex4Data.txt contain node data that you may test your program with. minimalSpanTreeType.h: #ifndef H_queueADT #define H_queueADT template class queueADT { public: virtual bool isEmptyQueue() const = 0; //Function to determine whether the queue is empty. //Postcondition: Returns true if the queue is empty, // otherwise returns false. virtual bool isFullQueue() const = 0; //Function to determine whether the queue is full. //Postcondition: Returns true if the queue is full, // otherwise returns false. virtual void initializeQueue() = 0; //Function to initialize the queue to an empty state. //Postcondition: The queue is empty. virtual Type front() const = 0; //Function to return the first element of the queue. //Precondition: The queue exists and is not empty. //Postcondition: If the queue is empty, the program // terminates; otherwise, the first // element of the queue is returned. virtual Type back() const = 0; //Function to return the last element of the queue. //Precondition: The queue exists and is not empty. //Postcondition: If the queue is empty, the program // terminates; otherwise, the last // element of the queue is returned. virtual void addQueue(const Type& queueElement) = 0; //Function to add queueElement to the queue. //Precondition: The queue exists and is not full. //Postcondition: The queue is changed and queueElement // is added to the queue. virtual void deleteQueue() = 0; //Function to remove the first element of the queue. //Precondition: The queue exists and is not empty. //Postcondition: The queue is changed and the first // element is removed from the queue. }; #endif
Write a program (in main.cpp) that: Prompts the user for a filename containing node data. Outputs the minimal spanning tree for a given graph. You will need to implement the createSpanningGraph method in minimalSpanTreeType.h to create the graph and the weight matrix. Note: Files Ch20_Ex21Data.txt and Ch20_Ex4Data.txt contain node data that you may test your program with. minimalSpanTreeType.h: #ifndef H_queueADT #define H_queueADT template class queueADT { public: virtual bool isEmptyQueue() const = 0; //Function to determine whether the queue is empty. //Postcondition: Returns true if the queue is empty, // otherwise returns false. virtual bool isFullQueue() const = 0; //Function to determine whether the queue is full. //Postcondition: Returns true if the queue is full, // otherwise returns false. virtual void initializeQueue() = 0; //Function to initialize the queue to an empty state. //Postcondition: The queue is empty. virtual Type front() const = 0; //Function to return the first element of the queue. //Precondition: The queue exists and is not empty. //Postcondition: If the queue is empty, the program // terminates; otherwise, the first // element of the queue is returned. virtual Type back() const = 0; //Function to return the last element of the queue. //Precondition: The queue exists and is not empty. //Postcondition: If the queue is empty, the program // terminates; otherwise, the last // element of the queue is returned. virtual void addQueue(const Type& queueElement) = 0; //Function to add queueElement to the queue. //Precondition: The queue exists and is not full. //Postcondition: The queue is changed and queueElement // is added to the queue. virtual void deleteQueue() = 0; //Function to remove the first element of the queue. //Precondition: The queue exists and is not empty. //Postcondition: The queue is changed and the first // element is removed from the queue. }; #endif
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
I could use help, me please:
Write a
- Prompts the user for a filename containing node data.
- Outputs the minimal spanning tree for a given graph.
You will need to implement the createSpanningGraph method in minimalSpanTreeType.h to create the graph and the weight matrix.
Note: Files Ch20_Ex21Data.txt and Ch20_Ex4Data.txt contain node data that you may test your program with.
minimalSpanTreeType.h:
#ifndef H_queueADT
#define H_queueADT
template <class Type>
class queueADT
{
public:
virtual bool isEmptyQueue() const = 0;
//Function to determine whether the queue is empty.
//Postcondition: Returns true if the queue is empty,
// otherwise returns false.
virtual bool isFullQueue() const = 0;
//Function to determine whether the queue is full.
//Postcondition: Returns true if the queue is full,
// otherwise returns false.
virtual void initializeQueue() = 0;
//Function to initialize the queue to an empty state.
//Postcondition: The queue is empty.
virtual Type front() const = 0;
//Function to return the first element of the queue.
//Precondition: The queue exists and is not empty.
//Postcondition: If the queue is empty, the program
// terminates; otherwise, the first
// element of the queue is returned.
virtual Type back() const = 0;
//Function to return the last element of the queue.
//Precondition: The queue exists and is not empty.
//Postcondition: If the queue is empty, the program
// terminates; otherwise, the last
// element of the queue is returned.
virtual void addQueue(const Type& queueElement) = 0;
//Function to add queueElement to the queue.
//Precondition: The queue exists and is not full.
//Postcondition: The queue is changed and queueElement
// is added to the queue.
virtual void deleteQueue() = 0;
//Function to remove the first element of the queue.
//Precondition: The queue exists and is not empty.
//Postcondition: The queue is changed and the first
// element is removed from the queue.
};
#endif
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
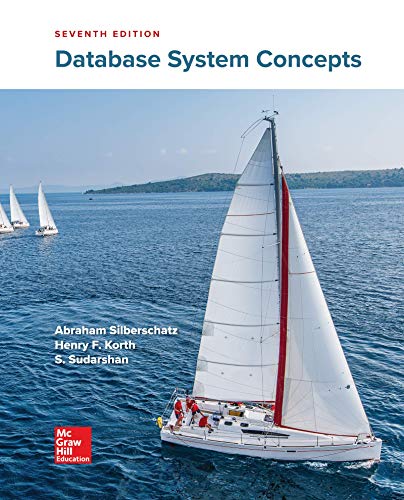
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
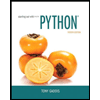
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
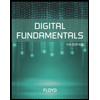
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
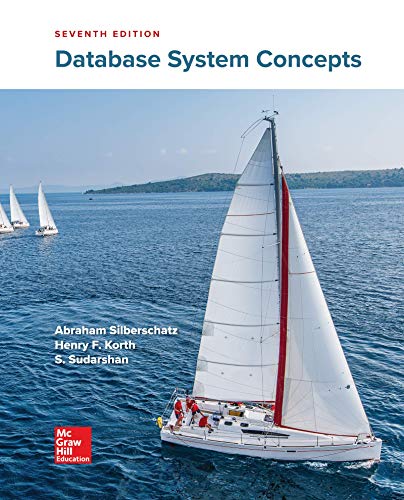
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
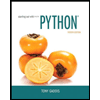
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
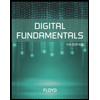
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
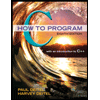
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
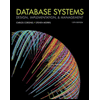
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
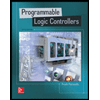
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education