Define a class named LinkedList that represents a singly linked list. The class should have the following member functions: Linked List: A constructor that initializes an empty linked list. void insertAtEnd(int data): Inserts a new node with the given data at the end of the linked list. void deleteNode(int data): Deletes the first occurrence of the node with the specified data from the linked list. void display(): Displays the elements of the linked list. Additionally, implement a function int countNodes() outside the class that returns the total number of nodes in the linked list. Create a program to test the functionality of the Linked List class. Use the following code as a starting point: #include using namespace std; // Define the Linked List class here int countNodes(const LinkedList& list); int main() { Linked List myList; // Create an empty linked list // Test the insertAtEnd function myList.insertAtEnd(10); myList.insertAtEnd(20); myList.insertAtEnd(30); } cout << "Initial Linked List: "; myList.display(); cout <<< endl; // Test the deleteNode function myList.deleteNode(20); cout << "Linked List after deleting 20: "; myList.display(); cout << endl; // Test the countNodes function cout << "Number of nodes in the linked list: " << countNodes(myList) << endl; return 0;
Define a class named LinkedList that represents a singly linked list. The class should have the following member functions: Linked List: A constructor that initializes an empty linked list. void insertAtEnd(int data): Inserts a new node with the given data at the end of the linked list. void deleteNode(int data): Deletes the first occurrence of the node with the specified data from the linked list. void display(): Displays the elements of the linked list. Additionally, implement a function int countNodes() outside the class that returns the total number of nodes in the linked list. Create a program to test the functionality of the Linked List class. Use the following code as a starting point: #include using namespace std; // Define the Linked List class here int countNodes(const LinkedList& list); int main() { Linked List myList; // Create an empty linked list // Test the insertAtEnd function myList.insertAtEnd(10); myList.insertAtEnd(20); myList.insertAtEnd(30); } cout << "Initial Linked List: "; myList.display(); cout <<< endl; // Test the deleteNode function myList.deleteNode(20); cout << "Linked List after deleting 20: "; myList.display(); cout << endl; // Test the countNodes function cout << "Number of nodes in the linked list: " << countNodes(myList) << endl; return 0;
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Send me your own work not AI generated response or plagiarised response. If I see these things, I'll give multiple downvotes and will definitely report.

Transcribed Image Text:Define a class named LinkedList that represents a singly linked list. The class should
have the following member functions:
Linked List: A constructor that initializes an empty linked list.
void insertAtEnd(int data): Inserts a new node with the given data at the end of the
linked list.
void deleteNode(int data): Deletes the first occurrence of the node with the specified
data from the linked list.
void display(): Displays the elements of the linked list.
Additionally, implement a function int countNodes() outside the class that returns the
total number of nodes in the linked list.
Create a program to test the functionality of the Linked List class. Use the following
code as a starting point:
#include <iostream>
using namespace std;
// Define the Linked List class here
int countNodes(const Linked List& list);
int main() {
Linked List myList; // Create an empty linked list
}
// Test the insertAtEnd function
myList.insertAtEnd(10);
myList.insertAtEnd(20);
myList.insertAtEnd(30);
cout<< "Initial Linked List: ";
myList.display();
cout << endl;
// Test the deleteNode function
myList.deleteNode(20);
cout << "Linked List after deleting 20: ";
myList.display();
cout << endl;
// Test the countNodes function
cout << "Number of nodes in the linked list: " << countNodes(myList) << endl;
return 0;
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 5 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
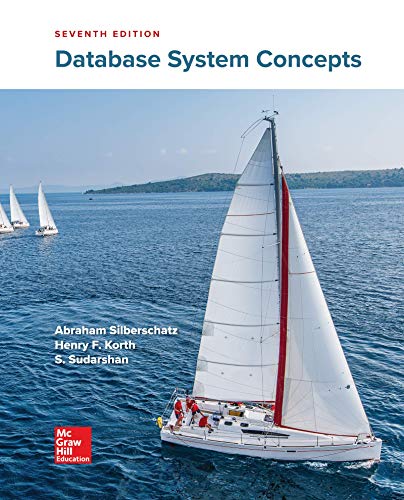
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
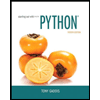
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
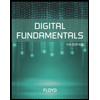
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
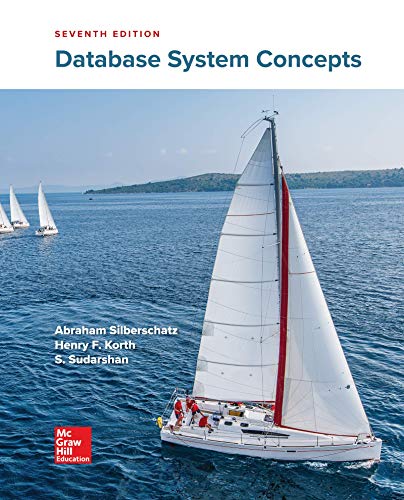
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
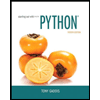
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
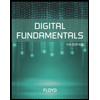
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
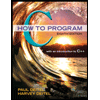
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
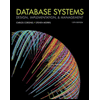
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
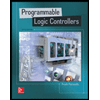
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education