Please follow the comments and please do exactly according to the comments. import java.util.Scanner; public class SortTemps { public static final int MAX_TEMPS = 1000; // As specified in the directions. public static void main(String[] args) { // Temporary arrays to store all the incoming data. int[] temperature = new int[MAX_TEMPS]; String[] city = new String[MAX_TEMPS]; int longestCityLength = 0; // Needed to make temperatures line up int count = 0; // Counts actual number of pairs Scanner inScanner = new Scanner(System.in); System.out.println("Enter temperature & city pairs:"); while (inScanner.hasNextInt()) { temperature[count] = inScanner.nextInt(); city[count] = inScanner.nextLine().trim(); if (city[count].length() > longestCityLength) { longestCityLength = city[count].length(); } count += 1; } // RIGHT AFTER THIS COMMENT declare an instantiate cityTempPairArray // to be an array of length count, holding objects that store the // city/temperature pairs. // HINT: you will have to create a class declaration (in another // .java file) for a new type of class that holds a city // and temperature pair. for (int i = 0; i < count; i += 1) { // REPLACE the following two lines with code that // constructs a new object holding city[i] and temperature[i], // and store that new object in cityTempPairArray[i]. String padding = " ".repeat(longestCityLength - city[i].length() + 1); System.out.println(city[i] + padding + temperature[i]); } // Insert code to bubble sort cityTempPairArray, putting the // object with the highest temperature at slot 0. System.out.println("The data sorted by temperature--highest first:"); // Insert code to print the contents of cityTempPairArray to output. // Use the strategy I used in the first for loop above // to line the temperatures up in columns. }
Please follow the comments and please do exactly according to the comments.
import java.util.Scanner;
public class SortTemps
{
public static final int MAX_TEMPS = 1000;
// As specified in the directions.
public static void main(String[] args)
{
// Temporary arrays to store all the incoming data.
int[] temperature = new int[MAX_TEMPS];
String[] city = new String[MAX_TEMPS];
int longestCityLength = 0; // Needed to make temperatures line up
int count = 0; // Counts actual number of pairs
Scanner inScanner = new Scanner(System.in);
System.out.println("Enter temperature & city pairs:");
while (inScanner.hasNextInt())
{
temperature[count] = inScanner.nextInt();
city[count] = inScanner.nextLine().trim();
if (city[count].length() > longestCityLength)
{
longestCityLength = city[count].length();
}
count += 1;
}
// RIGHT AFTER THIS COMMENT declare an instantiate cityTempPairArray
// to be an array of length count, holding objects that store the
// city/temperature pairs.
// HINT: you will have to create a class declaration (in another
// .java file) for a new type of class that holds a city
// and temperature pair.
for (int i = 0; i < count; i += 1)
{
// REPLACE the following two lines with code that
// constructs a new object holding city[i] and temperature[i],
// and store that new object in cityTempPairArray[i].
String padding = " ".repeat(longestCityLength - city[i].length() + 1);
System.out.println(city[i] + padding + temperature[i]);
}
// Insert code to bubble sort cityTempPairArray, putting the
// object with the highest temperature at slot 0.
System.out.println("The data sorted by temperature--highest first:");
// Insert code to print the contents of cityTempPairArray to output.
// Use the strategy I used in the first for loop above
// to line the temperatures up in columns.
}
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

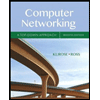
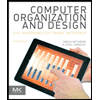
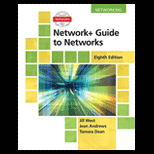
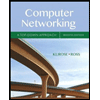
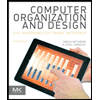
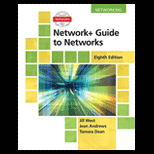
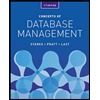
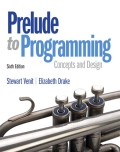
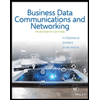