How do I change the code so if numMinutes is zero then the number cast members is also zero? Code: import java.util.*; import java.util.Arrays; public class Movie { private String movieName; private int numMinutes; private boolean isKidFriendly; private int numCastMembers; private String[] castMembers; // default constructor public Movie() { this.movieName = "Flick"; this.numMinutes = 0; this.isKidFriendly = false; this.numCastMembers = 0; this.castMembers = new String[10]; } // overloaded parameterized constructor public Movie(String movieName, int numMinutes, boolean isKidFriendly, String[] castMembers) { this.movieName = movieName; this.numMinutes = numMinutes; this.isKidFriendly = isKidFriendly; this.numCastMembers = castMembers.length; this.castMembers = new String[numCastMembers]; for (int i = 0; i < castMembers.length; i++) { this.castMembers[i] = castMembers[i]; } } // set the number of minutes public void setNumMinutes(int numMinutes) { this.numMinutes = numMinutes; } // set the movie name public void setMovieName(String movieName) { this.movieName = movieName; } // set if the movie is kid friendly or not public void setIsKidFriendly(boolean isKidFriendly) { this.isKidFriendly = isKidFriendly; } // return the movie name public String getMovieName() { return this.movieName; } // return the number of minutes public int getNumMinutes() { return this.numMinutes; } // return true if movie is kid friendly else false public boolean isKidFriendly() { return this.isKidFriendly; } // return the array of cast members public String[] getCastMembers() { // create a deep copy of the array String[] copyCastMembers = new String[this.castMembers.length]; // copy the strings from the array to the copy for (int i = 0; i < this.castMembers.length; i++) { copyCastMembers[i] = this.castMembers[i]; } return copyCastMembers; } // return the number of cast members public int getNumCastMembers() { return this.numCastMembers; } // method that allows the name of a castMember at an index in the castMembers // array to be changed public boolean replaceCastMember(int index, String castMemberName) { if (index < 0 || index >= numCastMembers) return false; castMembers[index] = castMemberName; return true; } // method that determines the equality of two String arrays and returns a // boolean, by comparing the value at each index location. // Return true if all elements of both arrays match, return false if there is // any mismatch. public boolean doArraysMatch(String[] arr1, String[] arr2) { // both arrays are null if (arr1 == null && arr2 == null) return true; else if (arr1 == null || arr2 == null) // one of the array is null return false; else if (arr1.length != arr2.length) // length of arrays do not match return false; // length of both arrays are same // loop over the arrays for (int i = 0; i < arr1.length; i++) { // if ith element are not equal, return false if (!arr1[i].equalsIgnoreCase(arr2[i])) return false; } return true; // length of arrays are same and each element at respective location are same } // method that gets the contents of the array of castMembers as a comma // separated String. public String getCastMemberNamesAsString() { if (numCastMembers == 0) { return "none"; } String names = castMembers[0]; for (int i = 1; i < numCastMembers; i++) { names += ", " + castMembers[i]; } return names; } // return String representation of the movie public String toString() { // format the numMinutes to 3 digits String movie = "Movie: [ Minutes "+String.format("%03d", numMinutes)+" | Movie Name: %21s |"; if (isKidFriendly) movie +=" is kid friendly |"; else movie +=" not kid friendly |"; movie +=" Number of Cast Members: "+ numCastMembers+ " | Cast Members: "+getCastMemberNamesAsString()+" ]"; return String.format(movie,movieName); } // returns true if this movie is equal to o public boolean equals(Object o) { // check if o is an instance of Movie if (o instanceof Movie) { Movie other = (Movie) o; // returns true if all the fields are equal return ((movieName.equalsIgnoreCase(other.movieName)) && (isKidFriendly == other.isKidFriendly) && (numMinutes == other.numMinutes) && (numCastMembers == other.numCastMembers) && (doArraysMatch(castMembers, other.castMembers))); } return false; // movies are not equal or o is not an object of Movie } public static void main(String args[]) { //This is the added main method } { //change the code according to your requiredment } }
How do I change the code so if numMinutes is zero then the number cast members is also zero?
Code:
import java.util.*;
import java.util.Arrays;
public class Movie
{
private String movieName;
private int numMinutes;
private boolean isKidFriendly;
private int numCastMembers;
private String[] castMembers;
// default constructor
public Movie()
{
this.movieName = "Flick";
this.numMinutes = 0;
this.isKidFriendly = false;
this.numCastMembers = 0;
this.castMembers = new String[10];
}
// overloaded parameterized constructor
public Movie(String movieName, int numMinutes, boolean isKidFriendly, String[] castMembers)
{
this.movieName = movieName;
this.numMinutes = numMinutes;
this.isKidFriendly = isKidFriendly;
this.numCastMembers = castMembers.length;
this.castMembers = new String[numCastMembers];
for (int i = 0; i < castMembers.length; i++)
{
this.castMembers[i] = castMembers[i];
}
}
// set the number of minutes
public void setNumMinutes(int numMinutes)
{
this.numMinutes = numMinutes;
}
// set the movie name
public void setMovieName(String movieName)
{
this.movieName = movieName;
}
// set if the movie is kid friendly or not
public void setIsKidFriendly(boolean isKidFriendly)
{
this.isKidFriendly = isKidFriendly;
}
// return the movie name
public String getMovieName()
{
return this.movieName;
}
// return the number of minutes
public int getNumMinutes()
{
return this.numMinutes;
}
// return true if movie is kid friendly else false
public boolean isKidFriendly()
{
return this.isKidFriendly;
}
// return the array of cast members
public String[] getCastMembers()
{
// create a deep copy of the array
String[] copyCastMembers = new String[this.castMembers.length];
// copy the strings from the array to the copy
for (int i = 0; i < this.castMembers.length; i++)
{
copyCastMembers[i] = this.castMembers[i];
}
return copyCastMembers;
}
// return the number of cast members
public int getNumCastMembers()
{
return this.numCastMembers;
}
// method that allows the name of a castMember at an index in the castMembers
// array to be changed
public boolean replaceCastMember(int index, String castMemberName)
{
if (index < 0 || index >= numCastMembers)
return false;
castMembers[index] = castMemberName;
return true;
}
// method that determines the equality of two String arrays and returns a
// boolean, by comparing the value at each index location.
// Return true if all elements of both arrays match, return false if there is
// any mismatch.
public boolean doArraysMatch(String[] arr1, String[] arr2)
{
// both arrays are null
if (arr1 == null && arr2 == null)
return true;
else if (arr1 == null || arr2 == null) // one of the array is null
return false;
else if (arr1.length != arr2.length) // length of arrays do not match
return false;
// length of both arrays are same
// loop over the arrays
for (int i = 0; i < arr1.length; i++)
{
// if ith element are not equal, return false
if (!arr1[i].equalsIgnoreCase(arr2[i]))
return false;
}
return true; // length of arrays are same and each element at respective location are same
}
// method that gets the contents of the array of castMembers as a comma
// separated String.
public String getCastMemberNamesAsString()
{
if (numCastMembers == 0)
{
return "none";
}
String names = castMembers[0];
for (int i = 1; i < numCastMembers; i++)
{
names += ", " + castMembers[i];
}
return names;
}
// return String representation of the movie
public String toString() {
// format the numMinutes to 3 digits
String movie = "Movie: [ Minutes "+String.format("%03d", numMinutes)+" | Movie Name: %21s |";
if (isKidFriendly)
movie +=" is kid friendly |";
else
movie +=" not kid friendly |";
movie +=" Number of Cast Members: "+ numCastMembers+ " | Cast Members: "+getCastMemberNamesAsString()+" ]";
return String.format(movie,movieName);
}
// returns true if this movie is equal to o
public boolean equals(Object o)
{
// check if o is an instance of Movie
if (o instanceof Movie)
{
Movie other = (Movie) o;
// returns true if all the fields are equal
return ((movieName.equalsIgnoreCase(other.movieName)) && (isKidFriendly == other.isKidFriendly)
&&
(numMinutes == other.numMinutes) && (numCastMembers == other.numCastMembers)
&&
(doArraysMatch(castMembers, other.castMembers)));
}
return false; // movies are not equal or o is not an object of Movie
}
public static void main(String args[]) { //This is the added main method
}
{
//change the code according to your requiredment
}
}

Step by step
Solved in 3 steps with 6 images

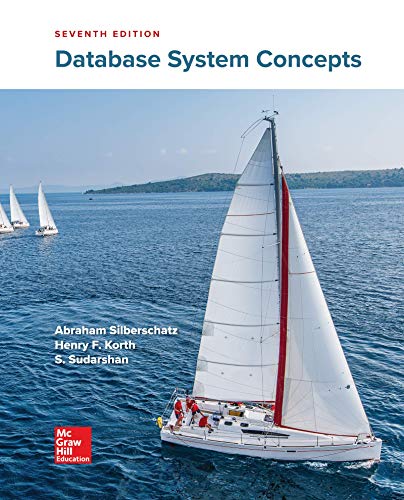
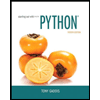
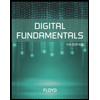
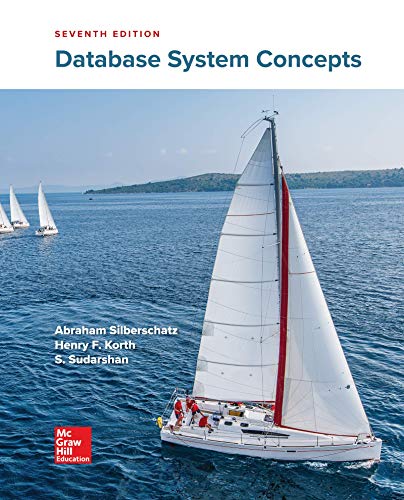
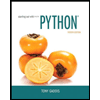
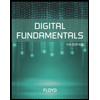
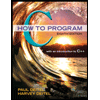
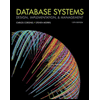
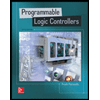