Make this separate JacaScript code into one consolidated file. (Code already given, you just need to combine all the codes in file) 1. Write a Javascript function that would accept any number and return the square of that number. (Make code visible) example: var a = square(4); a = should have a value of 16 Square 2. Write a JavaScript function that would accept a string and return the reverse of that string. example: reverse("john doe"); - this returns "eod nhoj" // arrow function to reverse to the string const reverse = (str) => { const arr = []; // to store the characterin reverse order const len = str.length; //finding the length of the string // iterating over the string for (let i = len; i >= 0; i--) arr.push(str[i]); //adding characters of string to array return arr.join(''); //returning the array } //dummy string for testing the function stringTest = "john doe"; // calling the function and storing the response reverseString = reverse(stringTest); //printing the result console.log(`Reverse of the string "${stringTest}" is: "${reverseString}"`); 3. Write a JavaScript function that would accept a string and checks whether a passed string is palindrome? example: palindrome("010"); - this returns TRUE palindrome("1011"); - this returns FALSE function check_Palindrome(str_entry){ // Change the string into lower case and remove all non-alphanumeric characters var cstr = str_entry.toLowerCase().replace(/[^a-zA-Z0-9]+/g,''); var ccount = 0; // string is empty or not if(cstr==="") { console.log("string is not found"); return false; } // to check the string length is even or odd if ((cstr.length) % 2 === 0) { ccount = (cstr.length) / 2; } else { // If string length is 1 then it palindrome if (cstr.length === 1) { console.log("The string is a palindrome."); return true; } else { // If the string length is odd ignore the middle character ccount = (cstr.length - 1) / 2; } } // loop to check the first character to the last character and then move next for (var p = 0; p < ccount; p++) { // Compare characters if it doesn't match if (cstr[p] != cstr.slice(-1-p)[0]) { console.log("The string is not a palindrome."); return false; } } console.log("The string is a palindrome."); return true; } check_Palindrome('010'); check_Palindrome('1011'); 4. Write a Javascript function that would accept two numbers and return the swap of two numbers. example: var a = 2, b = 3; swap(a, b); a = should be 3 and b = should be 2 Swap Two Numbers 5.Write a JavaScript function that would accept a string and returns its letters in alphabetical order. alphabet("CSIT201") - returns "012CIST"
Make this separate JacaScript code into one consolidated file. (Code already given, you just need to combine all the codes in file)
1. Write a Javascript function that would accept any number and return the square of that number. (Make code visible)
example: var a = square(4); a = should have a value of 16
<html>
<head>
<title>Square</title>
</head>
<body>
<script type="text/javascript">
a=Number(prompt("Enter a Number"))
var res=1
res=a*a;
document.write("Square of "+a+" is:"+res)
</script>
</body>
</html>
2. Write a JavaScript function that would accept a string and return the reverse of that string.
example: reverse("john doe"); - this returns "eod nhoj"
// arrow function to reverse to the string
const reverse = (str) => {
const arr = []; // to store the characterin reverse order
const len = str.length; //finding the length of the string
// iterating over the string
for (let i = len; i >= 0; i--)
arr.push(str[i]); //adding characters of string to array
return arr.join(''); //returning the array
}
//dummy string for testing the function
stringTest = "john doe";
// calling the function and storing the response
reverseString = reverse(stringTest);
//printing the result
console.log(`Reverse of the string "${stringTest}" is: "${reverseString}"`);
3. Write a JavaScript function that would accept a string and checks whether a passed string is palindrome?
example: palindrome("010"); - this returns TRUE
palindrome("1011"); - this returns FALSE
function check_Palindrome(str_entry){
// Change the string into lower case and remove all non-alphanumeric characters
var cstr = str_entry.toLowerCase().replace(/[^a-zA-Z0-9]+/g,'');
var ccount = 0;
// string is empty or not
if(cstr==="") {
console.log("string is not found");
return false;
}
// to check the string length is even or odd
if ((cstr.length) % 2 === 0) {
ccount = (cstr.length) / 2;
} else {
// If string length is 1 then it palindrome
if (cstr.length === 1) {
console.log("The string is a palindrome.");
return true;
} else {
// If the string length is odd ignore the middle character
ccount = (cstr.length - 1) / 2;
}
}
// loop to check the first character to the last character and then move next
for (var p = 0; p < ccount; p++) {
// Compare characters if it doesn't match
if (cstr[p] != cstr.slice(-1-p)[0]) {
console.log("The string is not a palindrome.");
return false;
}
}
console.log("The string is a palindrome.");
return true;
}
check_Palindrome('010');
check_Palindrome('1011');
4. Write a Javascript function that would accept two numbers and return the swap of two numbers.
-
- example: var a = 2, b = 3; swap(a, b); a = should be 3 and b = should be 2
<html>
<head>
<title>Swap Two Numbers</title>
</head>
<body>
<script type="text/javascript">
let a = prompt('Enter the first variable: ')
let b = prompt('Enter the second variable: ')
document.write("The value of a before swapping = " ,a);
document.write("<br>");
document.write("\nThe value of b before swapping = " ,b);
document.write("<br>");
//creates temp variable
let temp;
//swap variables
temp = a;
a = b;
b = temp;
document.write("The value of a after swapping = " a);
document.write("<br>");
document.write("\nThe value of b after swapping = " b);
</script>
</body>
</html>
5.Write a JavaScript function that would accept a string and returns its letters in alphabetical order.
-
- alphabet("CSIT201") - returns "012CIST"
<html>
<body>
<script>
function alpha(str){
var arr = str.split(""); //splits the string
res = arr.sort().join(""); //sort the array and joins to form a string
return res; // returns the result
}
console.log(alpha("CSIT201"));
</script>

Step by step
Solved in 3 steps with 2 images

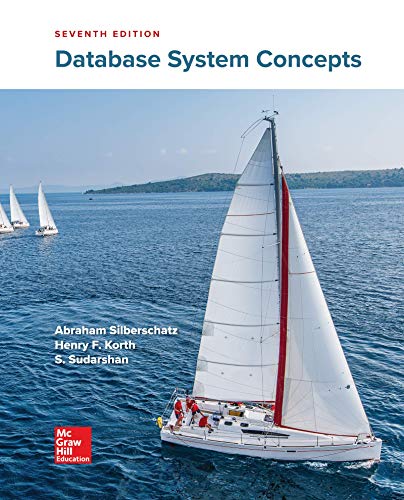
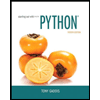
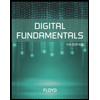
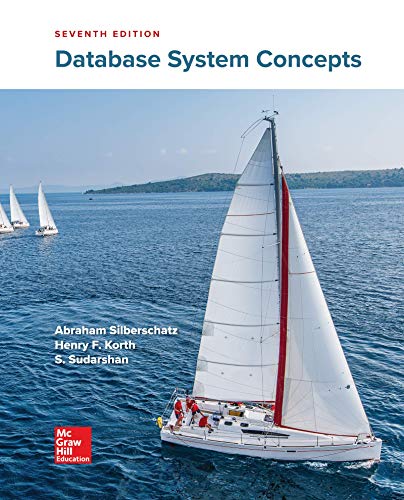
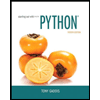
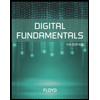
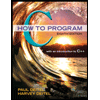
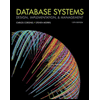
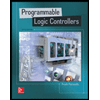