Average grades bar chart Write a python program grades.py that reads a file of assignment grades and draws a bar chart of the average grades for each assignment. Save the figure as grades.png in the current working directory. The format of a line in the file is: assignment_type grade Note that there is a single space between the assignment type and the grade. E.g. exam 92.80 Your program must contain the following functions: Function name Function description Function input(s) Function return value(s) get_grades() This function takes a file name as input and populates a dictionary containing the assignment types and grades. Filename string Dictionary of grades main() This function prompts for the grades file name. Next, it calls the functions get_grades(). Next, it draws the bar chart of the average grade for each assignment. Hint: You can use the mean function of the statistics module to calculate the average of a list. None None Use the starter code here import matplotlib.pyplot as drawing import statistics def get_grades(filename): """ This function takes a file name as input and populates a dictionary containing the assignment types and grades. @param filename: @return: dictionary of grades """ gradebook = dict() # todo return gradebook def main(): """ This function prompts for the grades file name. Next, it calls the functions get_grades(). Next, it draws the bar chart of the average grade for each assignment. @return: """ filename = input("Enter the grade file name: ") gradebook = get_grades(filename) print(f'The dictionary of grades is: {gradebook}') # todo if __name__ == '__main__': main() Some sample score files are provided (these are examples only-you do not need to assume these will be the same numbers) quiz 98 quiz 60.25 exam 92.80 quiz 100 exam 50 lab 82.76 quiz 75 quiz 76.23 quiz 82 lab 89 lab 100 exam 95.1 lab 76.88 Ex 2 exam 79 reading 25 reading 90 reading 93.08 quiz 40 exam 68.32 exam 72 quiz 46 quiz 80.19 lab 100 lab 80 reading 71 lab 78 Sample output Enter the grade file name: grades1.txt The dictionary of grades is: {'quiz': [98.0, 60.25, 100.0, 75.0, 76.23, 82.0], 'exam': [92.8, 50.0, 95.1], 'lab': [82.76, 89.0, 100.0, 76.88]} Expected bar chart below Sample output Enter the grade file name: data/grades2.txt The dictionary of grades is: {'exam': [79.0, 68.32, 72.0], 'reading': [25.0, 90.0, 93.08, 71.0], 'quiz': [40.0, 46.0, 80.19], 'lab': [100.0, 80.0, 78.0]} Expected bar chart below
Average grades bar chart
Write a python program grades.py that reads a file of assignment grades and draws a bar chart of the average grades for each assignment.
Save the figure as grades.png in the current working directory.
The format of a line in the file is: assignment_type grade
Note that there is a single space between the assignment type and the grade. E.g. exam 92.80
Your program must contain the following functions:
Function name |
Function description |
Function input(s) |
Function return value(s) |
---|---|---|---|
get_grades() |
This function takes a file name as input and populates a dictionary containing the assignment types and grades. |
Filename string |
Dictionary of grades |
main() |
This function prompts for the grades file name. Next, it calls the functions get_grades(). Next, it draws the bar chart of the average grade for each assignment. Hint: You can use the mean function of the statistics module to calculate the average of a list. |
None |
None |
Use the starter code here
import matplotlib.pyplot as drawing
import statistics
def get_grades(filename):
""" This function takes a file name as input and populates a dictionary containing the assignment types and grades.
@param filename:
@return: dictionary of grades """
gradebook = dict()
# todo
return gradebook
def main():
""" This function prompts for the grades file name. Next, it calls the functions get_grades(). Next, it draws the bar chart of the average grade for each assignment.
@return: """
filename = input("Enter the grade file name: ")
gradebook = get_grades(filename)
print(f'The dictionary of grades is: {gradebook}')
# todo
if __name__ == '__main__':
main()
Some sample score files are provided (these are examples only-you do not need to assume these will be the same numbers)
quiz 98
quiz 60.25
exam 92.80
quiz 100
exam 50
lab 82.76
quiz 75
quiz 76.23
quiz 82
lab 89
lab 100
exam 95.1
lab 76.88
Ex 2
exam 79
reading 25
reading 90
reading 93.08
quiz 40
exam 68.32
exam 72
quiz 46
quiz 80.19
lab 100
lab 80
reading 71
lab 78
Sample output
Enter the grade file name: grades1.txt The dictionary of grades is: {'quiz': [98.0, 60.25, 100.0, 75.0, 76.23, 82.0], 'exam': [92.8, 50.0, 95.1], 'lab': [82.76, 89.0, 100.0, 76.88]} |
Expected bar chart below
Sample output
Enter the grade file name: data/grades2.txt The dictionary of grades is: {'exam': [79.0, 68.32, 72.0], 'reading': [25.0, 90.0, 93.08, 71.0], 'quiz': [40.0, 46.0, 80.19], 'lab': [100.0, 80.0, 78.0]} |
Expected bar chart below



Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

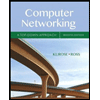
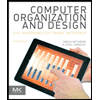
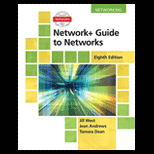
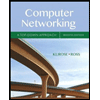
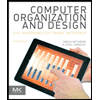
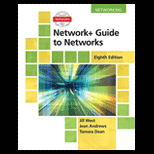
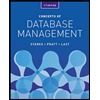
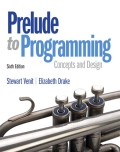
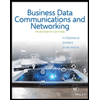