s the unparsed raw data (use vector here to get the unparsed raw data) Parse: A external function to Parse the data. The function accepts the raw data and returns the data parsed by the function. This type of function is called a "Call-Back Function". A Call-Back is necessary for each file as each file requires different regular expressions to parse. With binary files, need to use RegEx to do the parse(). [My code doesn't have this part] Please refer to the Callback.cpp example on how to setup a Call-Back. CallBack.cpp:
C++
I cannot run the code. Please help me fix it.
Below is the requirement of the code.
Design and write a C++ class that reads text, binary and csv files. The class functions:
Size: Returns the file size
Name: Returns the file name
Raw: Returns the unparsed raw data (use
Parse: A external function to Parse the data. The function accepts the raw data and returns the data parsed by the function. This type of function is called a "Call-Back Function". A Call-Back is necessary for each file as each file requires different regular expressions to parse.
With binary files, need to use RegEx to do the parse(). [My code doesn't have this part]
Please refer to the Callback.cpp example on how to setup a Call-Back.
CallBack.cpp:
#include <string>
#include <functional>
#include <iostream>
#include <vector>
using namespace std;
string ToLower(string s)
{
string temp;
for (char c : s)
temp.push_back(tolower(c));
temp.push_back(NULL);
return temp;
}
string ToUpper(string s)
{
string temp;
for (char c : s)
temp.push_back(toupper(c));
temp.push_back(NULL);
return temp;
}
struct Append
{
string operator()(string a, string b) // function object
{
return a + b;
}
};
class CallBack
{
function<string(string)> callback;
public:
CallBack(function<string(string)> cb) { callback = cb; }
string useCallBack(string s) { return callback(s); }
};
void embedd(string s)
{
CallBack cback(ToUpper);
cout << cback.useCallBack(s) << endl;
}
void functional()
{
string cat = "cat";
string dog = "DOG";
function<string(string)> fn1 = ToUpper; // ToLower; // function
function<string(string)> fn2 = &ToUpper; // function pointer
function<string(string, string)> fn3 = Append(); // function object
function<int(string)> fn4 = [](string s) { return s.length(); }; // lambda expression
function<int(int, int)> fn5 = plus<int>(); // standard function object
cout << fn1(dog) << endl;
cout << fn2(cat) << endl;
cout << fn3(cat, dog) << endl;
cout << fn4(dog) << endl;
cout << fn5(123, 456) << endl;
}
int main()
{
functional();
embedd("dog");
}
myCode.cpp
#include <iostream>
#include <fstream>
#include <string>
#include <vector>
#include <functional>
using namespace std;
class FileReader {
private:
string fileName;
vector<char> rawData;
int fileSize;
function<string(string)> callback;
public:
FileReader(string name, function<string(string)> cb) {
fileName = name;
callback = cb;
}
int size() {
return fileSize;
}
string name() {
return fileName;
}
vector<char> raw() {
return rawData;
}
void parse() {
// Read the file into the raw data vector
ifstream file(fileName, ios::binary | ios::ate);
fileSize = file.tellg();
file.seekg(0, ios::beg);
rawData.resize(fileSize);
file.read(rawData.data(), fileSize);
// Convert the raw data vector to a string and call the callback function to parse the data
string rawDataStr(rawData.begin(), rawData.end());
rawDataStr = callback(rawDataStr);
vector<char> newRawData(rawDataStr.begin(), rawDataStr.end());
rawData = newRawData;
}
};

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

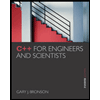
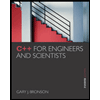