Find and replace problem You will implement a replica of find and replace option available in the word document !!! (attached below) Input: An initialized one dimensional character array of size 100 and the strings that would be found and replaced with. Output: The input character array after being replaced by the requested string. Sample Input: a:"A quick brown fox jumps over the lazy dog." Find: "fox", Replace: "tiger" Sample Output: "A quick brown tiger jumps over the lazy dog." Define a separate function that accepts three arguments as input string, find and replace string and implement the find and replace inside that function. You can use the strcmp() function for the string comparison along with other necessary libraries. You need to call that function from the main function and print the result in the main function. (e.g. use the pointer concept)
Find and replace problem
You will implement a replica of find and replace option available in the word document !!! (attached below)
Input: An initialized one dimensional character array of size 100 and the strings that would be found and replaced with.
Output: The input character array after being replaced by the requested string.
Sample Input: a:"A quick brown fox jumps over the lazy dog." Find: "fox", Replace: "tiger"
Sample Output: "A quick brown tiger jumps over the lazy dog."
Define a separate function that accepts three arguments as input string, find and replace string
and implement the find and replace inside that function. You can use the strcmp() function for the
string comparison along with other necessary libraries. You need to call that function
from the main function and print the result in the main function. (e.g. use the pointer concept)


#include<stdio.h>
#include<string.h>
void replaceSubstring(char string[],char find[],char new[])
{
int stringLen, findLen, newLen;
int i=0, j, k;
int flag=0, start, end;
stringLen = strlen(string);
findLen = strlen(find);
newLen = strlen(new);
for(i=0; i<stringLen; i++)
{
flag = 0;
start = i;
for(j=0; string[i] == find[j]; j++,i++)
if(j == findLen-1)
flag = 1;
end = i;
if(flag == 0)
i -= j;
else
{
for(j=start; j<end; j++)
{
for(k=start; k<stringLen; k++)
string[k] = string[k+1];
stringLen--;
i--;
}
for(j=start; j<start+newLen; j++)
{
for(k=stringLen; k>=j; k--)
string[k+1] = string[k];
string[j] = new[j-start];
stringLen++;
i++;
}
}
}
}
int main()
{
char string[100], find[100], new[100];
printf("Enter a string: ");
scanf("%[^\n]%*c", string);
printf("Enter the string to replace: ");
scanf("%[^\n]%*c", find);
printf("Enter the string to replace with: ");
scanf("%[^\n]%*c", new);
replaceSubstring(string, find, new);
printf("The string after replacing : %s\n",string);
return 0;
}
Step by step
Solved in 2 steps with 1 images

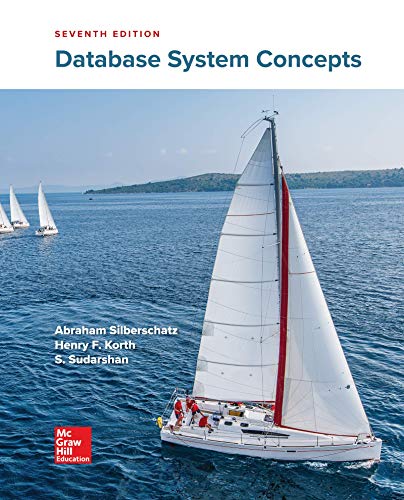
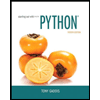
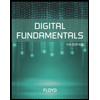
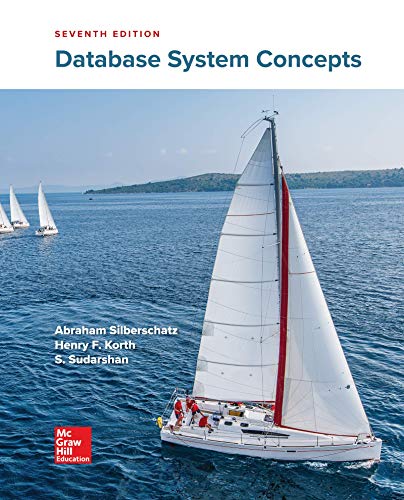
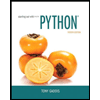
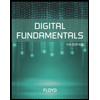
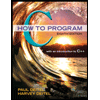
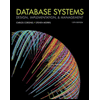
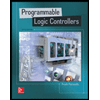