my code is # importing pandas for csv file operations import pandas as pd # filename to store the filename, change it to your filename fileName = 'survey.csv' # Creating a dataframe by opening the given file df = pd.read_csv(fileName) # converting the date string to datetime pd.to_datetime(df['Timestamp']) Question - Python code to drop the data values from your data set where the age is less than 1 or greater than 99 BY USING DROP FUNCTION. DO NOT CHANGE ORIGINAL DATA FRAME Example of function required is df_data.drop(df_data[df_data.salary<0].index,inplace=True) • Print the shape of your new data frame using the shape property. • Has the number of columns changed? • Has the number of rows changed? • Explain why each has or has not changed.
my code is
# importing pandas for csv file operations
import pandas as pd
# filename to store the filename, change it to your filename
fileName = 'survey.csv'
# Creating a dataframe by opening the given file
df = pd.read_csv(fileName)
# converting the date string to datetime
pd.to_datetime(df['Timestamp'])
Question - Python code to drop the data values from your data set where the age is less than 1 or greater than 99 BY USING DROP FUNCTION.
DO NOT CHANGE ORIGINAL DATA FRAME
Example of function required is df_data.drop(df_data[df_data.salary<0].index,inplace=True)
• Print the shape of your new data frame using the shape property.
• Has the number of columns changed?
• Has the number of rows changed?
• Explain why each has or has not changed.
![Timestamp Age Gender
37 Female
Country state self_employed \
2014-08-27 11:29:31
United States
IL
NaN
2014-08-27 11:29:37
44
M
United States
IN
NaN
Male
Canada
Male United Kingdom
United States
2
2014-08-27 11:29:44
32
NaN
NaN
2014-08-27 11:29:46
2014-08-27 11:30:22
3
31
NaN
NaN
4
31
Male
TX
NaN
...
...
...
...
...
male United Kingdom
Male
1254
2015-09-12 11:17:21
26
NaN
No
1255
2015-09-26 01:07:35
32
United States
IL
No
1256
2015-11-07 12:36:58
34
male
United States
CA
No
1257
2015-11-30 21:25:06
46
f
United States
NC
No
1258
2016-02-01 23:04:31
25
Male
United States
IL
No
family history treatment work interfere
Often
no_employees
...
No
Yes
6-25
Rarely More than 1000
Rarely
Often
1
No
No
2
No
No
6-25
3
Yes
Yes
26-100
4
No
No
Never
100-500
...
...
...
...
...
1254
No
Yes
NaN
26-100
1255
Yes
Yes
Often
26-100
1256
Yes
Yes
Sometimes More than 1000
1257
No
No
NaN
100-500
1258
Yes
Yes
Sometimes
26-100
leave mental_health_consequence phys_health_consequence \
Somewhat easy
Don't know
No
No
Maybe
No
2
Somewhat difficult
No
No
3
Somewhat difficult
Yes
Yes
4
Don't know
No
No
...
...
...
...
1254
Somewhat easy
No
No
1255 Somewhat difficult
No
No
1256 Somewhat difficult
Yes
Yes
1257
Don't know
Yes
No
1258
Don't know
Maybe
No
coworkers
supervisor mental_health_interview \
Some of them
Yes
No
1
No
No
No
Yes
Yes
Yes
Some of them
Some of them
3
No
Maybe
4
Yes
Yes
...
...
...
...
1254 Some of them Some of them
No
1255
Some of them
Yes
No
1256
No
No
No
1257
No
No
No
1258 Some of them
No
No
phys_health_interview mental_vs_physical obs_consequence comments
Maybe
Yes
No
NaN
1
No
Don't know
No
NaN
2
Yes
No
No
NaN
3
Maybe
No
Yes
NaN
4
Yes
Don't know
No
NaN
...
...
...
...
1254
No
Don't know
No
NaN
1255
No
Yes
No
NaN
1256
No
No
No
NaN
1257
No
No
No
NaN
1258
No
Don't know
No
NaN
[1259 rows x 27 columns]](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F4eafb156-293f-4083-8e25-3c3058268846%2Ff130d33e-7d8d-4ad6-8e91-ec37dcdd1dc6%2Fjknv8y_processed.png&w=3840&q=75)

Step by step
Solved in 3 steps

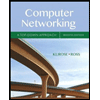
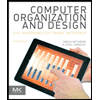
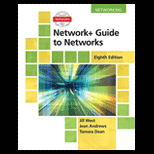
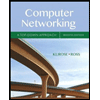
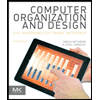
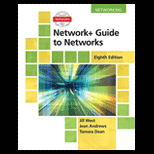
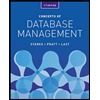
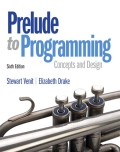
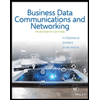