How do I code the C code for the main.c file to accomplish the following tasks outlined in the assignment (see images) to produce a similar palindromes output? Given Code: dynarray.c file code #include "dynarray.h" const int DEFAULT_DYNARRAY_CAPACITY = 2; void dynarray_init(dynarray_t *a) { /* allocate space for data */ a->array = (data_t*)malloc(DEFAULT_DYNARRAY_CAPACITY * sizeof(data_t)); if (a->array == NULL) { fprintf(stderr, "ERROR: Cannot allocate memory for dynamic array."); exit(-1); } /* initialize other metadata */ a->capacity = DEFAULT_DYNARRAY_CAPACITY; a->size = 0; a->front = 0; } void dynarray_expand(dynarray_t *a, size_t new_capacity) { size_t i; /* allocate new array */ data_t *new_array = (data_t*)malloc(new_capacity * sizeof(data_t)); if (!new_array) { fprintf(stderr, "ERROR: Cannot allocate memory for dynamic array."); exit(-1); } /* copy items to new array */ for (i = 0; i < a->size; i++) { new_array[i] = a->array[(a->front + i) % a->capacity]; } /* delete old array and clean up */ free(a->array); a->array = new_array; a->capacity = new_capacity; a->front = 0; } size_t dynarray_size(dynarray_t *a) { return a->size; } size_t dynarray_is_empty(dynarray_t *a) { return dynarray.h file code typedef int data_t; typedef struct { data_t *array; size_t capacity; size_t size; size_t front; } dynarray_t; /* dynamic array operations */ void dynarray_init(dynarray_t *a); void dynarray_expand(dynarray_t *a, size_t capacity); size_t dynarray_size(dynarray_t *a); size_t dynarray_is_empty(dynarray_t *a); void dynarray_free(dynarray_t *a); /* stack operations */ void dynarray_push(dynarray_t *a, data_t item); data_t dynarray_pop(dynarray_t *a); data_t dynarray_top(dynarray_t *a); /* queue operations */ void dynarray_enqueue(dynarray_t *a, data_t item); data_t dynarray_dequeue(dynarray_t *a); data_t dynarray_front(dynarray_t *a); #endif lab5.c file code #include "lab5.h" slnode_t* malloc_slnode(data_t data) { slnode_t *node = (slnode_t*)malloc(sizeof(slnode_t)); if (node == NULL) { fprintf(stderr, "ERROR: Cannot allocate memory for link list node."); exit(EXIT_FAILURE); } node->data = data; node->next = NULL; return node; } void sllist_init(sllist_t *list) { list->head = NULL; list->size = 0; } void sllist_addfirst(sllist_t *list, data_t item) { slnode_t *new_node = malloc_slnode(item); if (list->head != NULL) { new_node->next = list->head; } list->head = new_node; list->size++; } bool sllist_contains (sllist_t *list, data_t item) { slnode_t *n; // iterate over the linked list, looking for 'item' for (n = list->head; n != NULL; n = n->next) { if (n->data == item) { return true; } } return false; } size_t sllist_size(sllist_t *list) { return list->size; } bool is_palindrome(char *text) { return false; } size_t sllist_count (sllist_t *list, data_t item) { return 0; } int sllist_find (sllist_t *list, data_t item) { return -1; } void sllist_free (sllist_t *list) { } void sllist_addlast (sllist_t *list, data_t item) { } bool sllist_is_equal (sllist_t *list1, sllist_t *list2) { return false; } void sllist_insert (sllist_t *list, data_t item, size_t index) { } void sllist_remove (sllist_t *list, data_t item) {} lab5.h file code: typedef struct slnode { data_t data; struct slnode *next; } slnode_t; /* * Singly-linked list definition. typedef struct { slnode_t *head; size_t size; } sllist_t; PROVIDED FUNCTIONS //Allocate a new singly-linked list node with the given data value. slnode_t* malloc_slnode (data_t data); //Initialize a singly-linked list void sllist_init (sllist_t *list); //Add an item at the head of the list. void sllist_addfirst (sllist_t *list, data_t item); //Returns true if there is an item equal to the given one in the list. bool sllist_contains (sllist_t *list, data_t item); //Returns the number of items currently in the list. size_t sllist_size (sllist_t *list); //Return true if the given string is a palindrome; false otherwise. bool is_palindrome(char *text); //Returns the number of items equal to the given one in the list. size_t sllist_count (sllist_t *list, data_t item); // Returns the index of the first item equal to the given item. If no such item is found, returns -1. int sllist_find (sllist_t *list, data_t item); //Clean up the list, freeing all of the list nodes. void sllist_free (sllist_t *list); //Add an item at the tail of the list. void sllist_addlast (sllist_t *list, data_t item); //Returns true if the two lists have the same number of items, and each pair of corresponding items are also equal; false otherwise. bool sllist_is_equal (sllist_t *list1, sllist_t *list2); //Adds an item at the given index in the list. void sllist_insert (sllist_t *list, data_t item, size_t index); //Removed the first item in the list that is equal to the given item. If no such item is found, no changes are made. void sllist_remove (sllist_t *list, data_t item); #endif
How do I code the C code for the main.c file to accomplish the following tasks outlined in the assignment (see images) to produce a similar palindromes output?
Given Code:
dynarray.c file code
#include "dynarray.h"
const int DEFAULT_DYNARRAY_CAPACITY = 2;
void dynarray_init(dynarray_t *a) {
/* allocate space for data */
a->array = (data_t*)malloc(DEFAULT_DYNARRAY_CAPACITY * sizeof(data_t));
if (a->array == NULL) {
fprintf(stderr, "ERROR: Cannot allocate memory for dynamic array.");
exit(-1);
}
/* initialize other metadata */
a->capacity = DEFAULT_DYNARRAY_CAPACITY;
a->size = 0;
a->front = 0;
}
void dynarray_expand(dynarray_t *a, size_t new_capacity) {
size_t i;
/* allocate new array */
data_t *new_array = (data_t*)malloc(new_capacity * sizeof(data_t));
if (!new_array) {
fprintf(stderr, "ERROR: Cannot allocate memory for dynamic array.");
exit(-1);
}
/* copy items to new array */
for (i = 0; i < a->size; i++) {
new_array[i] = a->array[(a->front + i) % a->capacity];
}
/* delete old array and clean up */
free(a->array);
a->array = new_array;
a->capacity = new_capacity;
a->front = 0;
}
size_t dynarray_size(dynarray_t *a) {
return a->size;
}
size_t dynarray_is_empty(dynarray_t *a) {
return
dynarray.h file code
typedef int data_t;
typedef struct {
data_t *array;
size_t capacity;
size_t size;
size_t front;
} dynarray_t;
/* dynamic array operations */
void dynarray_init(dynarray_t *a);
void dynarray_expand(dynarray_t *a, size_t capacity);
size_t dynarray_size(dynarray_t *a);
size_t dynarray_is_empty(dynarray_t *a);
void dynarray_free(dynarray_t *a);
/* stack operations */
void dynarray_push(dynarray_t *a, data_t item);
data_t dynarray_pop(dynarray_t *a);
data_t dynarray_top(dynarray_t *a);
/* queue operations */
void dynarray_enqueue(dynarray_t *a, data_t item);
data_t dynarray_dequeue(dynarray_t *a);
data_t dynarray_front(dynarray_t *a);
#endif
lab5.c file code
#include "lab5.h"
slnode_t* malloc_slnode(data_t data) {
slnode_t *node = (slnode_t*)malloc(sizeof(slnode_t));
if (node == NULL) {
fprintf(stderr, "ERROR: Cannot allocate memory for link list node.");
exit(EXIT_FAILURE);
}
node->data = data;
node->next = NULL;
return node;
}
void sllist_init(sllist_t *list) {
list->head = NULL;
list->size = 0;
}
void sllist_addfirst(sllist_t *list, data_t item) {
slnode_t *new_node = malloc_slnode(item);
if (list->head != NULL) {
new_node->next = list->head;
}
list->head = new_node;
list->size++;
}
bool sllist_contains (sllist_t *list, data_t item) {
slnode_t *n;
// iterate over the linked list, looking for 'item'
for (n = list->head; n != NULL; n = n->next) {
if (n->data == item) {
return true;
}
}
return false;
}
size_t sllist_size(sllist_t *list) {
return list->size;
}
bool is_palindrome(char *text) {
return false;
}
size_t sllist_count (sllist_t *list, data_t item) {
return 0;
}
int sllist_find (sllist_t *list, data_t item) {
return -1;
}
void sllist_free (sllist_t *list) {
}
void sllist_addlast (sllist_t *list, data_t item) {
}
bool sllist_is_equal (sllist_t *list1, sllist_t *list2) {
return false;
}
void sllist_insert (sllist_t *list, data_t item, size_t index) {
}
void sllist_remove (sllist_t *list, data_t item) {}
lab5.h file code:
typedef struct slnode {
data_t data;
struct slnode *next;
} slnode_t;
/* * Singly-linked list definition.
typedef struct {
slnode_t *head;
size_t size;
} sllist_t;
PROVIDED FUNCTIONS
//Allocate a new singly-linked list node with the given data value.
slnode_t* malloc_slnode (data_t data);
//Initialize a singly-linked list
void sllist_init (sllist_t *list);
//Add an item at the head of the list.
void sllist_addfirst (sllist_t *list, data_t item);
//Returns true if there is an item equal to the given one in the list.
bool sllist_contains (sllist_t *list, data_t item);
//Returns the number of items currently in the list.
size_t sllist_size (sllist_t *list);
//Return true if the given string is a palindrome; false otherwise.
bool is_palindrome(char *text);
//Returns the number of items equal to the given one in the list.
size_t sllist_count (sllist_t *list, data_t item);
// Returns the index of the first item equal to the given item. If no such item is found, returns -1.
int sllist_find (sllist_t *list, data_t item);
//Clean up the list, freeing all of the list nodes.
void sllist_free (sllist_t *list);
//Add an item at the tail of the list.
void sllist_addlast (sllist_t *list, data_t item);
//Returns true if the two lists have the same number of items, and each pair of corresponding items are also equal; false otherwise.
bool sllist_is_equal (sllist_t *list1, sllist_t *list2);
//Adds an item at the given index in the list.
void sllist_insert (sllist_t *list, data_t item, size_t index);
//Removed the first item in the list that is equal to the given item. If no such item is found, no changes are made.
void sllist_remove (sllist_t *list, data_t item);
#endif



Trending now
This is a popular solution!
Step by step
Solved in 2 steps

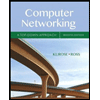
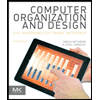
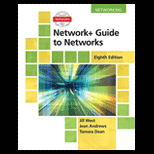
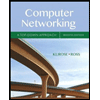
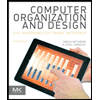
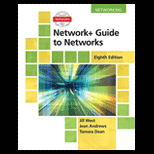
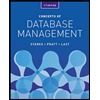
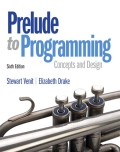
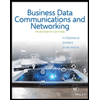