java programming A lot of people have been in the real estate market this year and 2 measurements are used to measure land, acres and hectares. You are writing a program to convert acres to hectares for lots of land given in acres. You begin by asking the user how many conversions that they want to make for lots of land. Once you have a response, a for loop is entered for processing the lots of land. You will input the lot# and the acres for the lot and this information will be stored in parallel arrays. Use a value returning method to validate the value of the acres to make sure that it is not <1 acre. Print the lot#, and the acres within a 2nd for loop. Find the lot with the smallest acreage. Use a void method convertHectares that converts the sum of the acres of land processed by a factor of 2.471052, and then displays the Hectares. The following methods are required in this program: A value returning method to validate the acres to make sure that it is at least 1. A void method to convert the sum of the acres to hectares and display the result. Below is the output of the program with some test data. Remember your program must run for any number of years and bushels of tomatoes and not just these years and bushels. Other reminders: Use camelCase correctly Indent correctly Comment at the top of each program and within each program Use print formatting to show 1 decimal place in the output for the total Bushels of Tomatoes and total Cubic Feet. Use the test data given for testing. Do not use object oriented classes and methods in this program that you may find on the Internet. This program must use a procedural approach. Here is how the program will run. Test data input is italicized and bolded. Let's analyze lot acreage for several lots. Please enter the number of lots: 3 Please enter the lot#. Abc123 Please enter the acreage for lot Abc123. 1 Please enter the lot#. Bcd234 Please enter the acreage for lot Bcd234. 2 Please enter the lot#. Cde345 Please enter the acreage for lot Cde345. 0 Invalid. Please enter a value that is at least 1. 7 ------Acreage Conversions------ Abc123 1 Bcd234 2 Cde345 7 The lot with the smallest acreage is Abc123 with 1 acre. The total hectares converted from acres is 24.71052.
java programming
A lot of people have been in the real estate market this year and 2 measurements are used to measure land, acres and hectares.
You are writing a program to convert acres to hectares for lots of land given in acres. You begin by asking the user how many conversions that they want to make for lots of land. Once you have a response, a for loop is entered for processing the lots of land.
You will input the lot# and the acres for the lot and this information will be stored in parallel arrays. Use a value returning method to validate the value of the acres to make sure that it is not <1 acre. Print the lot#, and the acres within a 2nd for loop. Find the lot with the smallest acreage. Use a void method convertHectares that converts the sum of the acres of land processed by a factor of 2.471052, and then displays the Hectares.
The following methods are required in this program:
A value returning method to validate the acres to make sure that it is at least 1.
A void method to convert the sum of the acres to hectares and display the result.
Below is the output of the program with some test data. Remember your program must run for any number of years and bushels of tomatoes and not just these years and bushels.
Other reminders:
Use camelCase correctly
Indent correctly
Comment at the top of each program and within each program
Use print formatting to show 1 decimal place in the output for the total Bushels of Tomatoes and total Cubic Feet.
Use the test data given for testing.
Do not use object oriented classes and methods in this program that you may find on the Internet. This program must use a procedural approach.
Here is how the program will run. Test data input is italicized and bolded.
Let's analyze lot acreage for several lots.
Please enter the number of lots:
3
Please enter the lot#.
Abc123
Please enter the acreage for lot Abc123.
1
Please enter the lot#.
Bcd234
Please enter the acreage for lot Bcd234.
2
Please enter the lot#.
Cde345
Please enter the acreage for lot Cde345.
0
Invalid. Please enter a value that is at least 1.
7
------Acreage Conversions------
Abc123 1
Bcd234 2
Cde345 7
The lot with the smallest acreage is Abc123 with 1 acre.
The total hectares converted from acres is 24.71052.

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

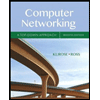
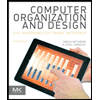
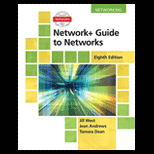
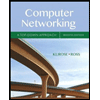
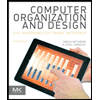
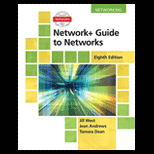
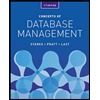
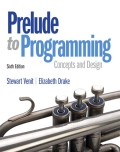
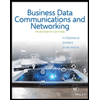