MUST BE IN PYTHON PROGRAMMING CODE!!!!! NO C++ or Java!! Directions: Design a program with a loop that lets the user enter a series of names (in no particular order). After the final person’s name has been entered, the program should display the name that is first alphabetically and the name that is last alphabetically. For example, if the user enters the names Kristin, Joel, Adam, Beth, Zeb, and Chris, the program would display Adam and Zeb. Additional requirements: Note that the possible set of input values can include negative, 0, or positive values, but not the sentinel value of -99. DESIGN HINT: • When determining the largest (or smallest) value in a set of numbers, remember to use variables to keep track of the current largest (or smallest) number found thus far. These variables can then be used as a comparison to each subsequent input number to see if the new number is larger (or smaller) than the current largest (or smallest) number and updated appropriately. At the end of all input, these variables will then contain the largest (or smallest) of all values input. Be careful on variable initialization—you will need to use a value that is in the set of possible input values. The IPO chart for this program is given below: Input: Series of numbers (-99 indicates end of input) Processing: Read user input numbers while input number does not equal -99. Keep track of largest and smallest numbers input by comparing each user input number with current smallest and largest numbers. Reset largest and smallest values based upon user input: If inputNum < smallest Then Set smallest = inputNum Else If inputNum > largest Then Set largest = inputNum End If Output: Largest and smallest number entered Additional Problem Requirements: • Create a main module as your program start module. Include comparisons to determine highest/lowest input values in the main module. • Only use simple data types, not arrays, in your implementation (i.e. do not use Python lists or min/max functions). • Include a separate module that uses pass by value parameters to descriptively display the passed largest and smallest numbers. Call this module from your main module with appropriate arguments. • Do not use global variables.
MUST BE IN PYTHON PROGRAMMING CODE!!!!! NO C++ or Java!!
Directions: Design a program with a loop that lets the user enter a series of names (in no particular order). After the final person’s name has been entered, the program should display the name that is first alphabetically and the name that is last alphabetically. For example, if the user enters the names Kristin, Joel, Adam, Beth, Zeb, and Chris, the program would display Adam and Zeb.
Additional requirements: Note that the possible set of input values can include negative, 0, or positive values, but not the sentinel value of -99.
DESIGN HINT: • When determining the largest (or smallest) value in a set of numbers, remember to use variables to keep track of the current largest (or smallest) number found thus far. These variables can then be used as a comparison to each subsequent input number to see if the new number is larger (or smaller) than the current largest (or smallest) number and updated appropriately. At the end of all input, these variables will then contain the largest (or smallest) of all values input. Be careful on variable initialization—you will need to use a value that is in the set of possible input values. The IPO chart for this program is given below:
Input:
Series of numbers (-99 indicates end of input) |
Processing:
Read user input numbers while input number does not equal -99. Keep track of largest and smallest numbers input by comparing each user input number with current smallest and largest numbers. Reset largest and smallest values based upon user input: If inputNum < smallest Then Set smallest = inputNum Else If inputNum > largest Then Set largest = inputNum End If |
Output:
Largest and smallest number entered |
Additional Problem Requirements:
• Create a main module as your program start module. Include comparisons to determine highest/lowest input values in the main module.
• Only use simple data types, not arrays, in your implementation (i.e. do not use Python lists or min/max functions).
• Include a separate module that uses pass by value parameters to descriptively display the passed largest and smallest numbers. Call this module from your main module with appropriate arguments.
• Do not use global variables.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

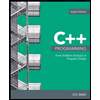
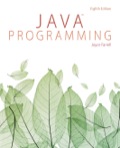
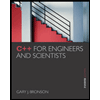
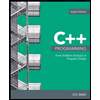
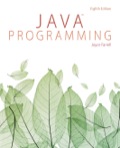
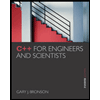
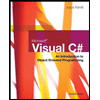
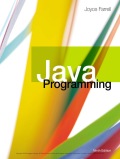