In this lab, you will make additions to a Java program that is provided. The program is a guessing game. A random number between 1 and 10 is generated in the program. The user enters a number between 1 and 10, trying to guess the correct number. If the user guesses correctly, the program congratulates the user, and then the loop that controls guessing numbers exits; otherwise, the program asks the user if he or she wants to guess again. If the user enters a "Y", he or she can guess again. If the user enters "N", the loop exits. You can see that the "Y" or an "N" is the sentinel value that controls the loop. Note that the entire program has been written for you. You need to add code that validates correct input, which is "Y" or "N", when the user is asked if he or she wants to guess a number, and a number in the range of 1 through 10 when the user is asked to guess a number. Instructions Ensure the file named GuessNumber.java is open. Write loops that validate input at all places in the code where the user is asked to provide input. Comments have been included in the code to help you identify where these loops should be written. Execute the program by clicking "Run Code." See if you can guess the randomly generated number. Execute the program several times to see if the random number changes. Also, test the program to verify that incorrect input is handled correctly. On your best attempt, how many guesses did you have to take to guess the correct number?
Types of Loop
Loops are the elements of programming in which a part of code is repeated a particular number of times. Loop executes the series of statements many times till the conditional statement becomes false.
Loops
Any task which is repeated more than one time is called a loop. Basically, loops can be divided into three types as while, do-while and for loop. There are so many programming languages like C, C++, JAVA, PYTHON, and many more where looping statements can be used for repetitive execution.
While Loop
Loop is a feature in the programming language. It helps us to execute a set of instructions regularly. The block of code executes until some conditions provided within that Loop are true.
Validating User Input
Summary
In this lab, you will make additions to a Java program that is provided. The program is a guessing game. A random number between 1 and 10 is generated in the program. The user enters a number between 1 and 10, trying to guess the correct number.
If the user guesses correctly, the program congratulates the user, and then the loop that controls guessing numbers exits; otherwise, the program asks the user if he or she wants to guess again. If the user enters a "Y", he or she can guess again. If the user enters "N", the loop exits. You can see that the "Y" or an "N" is the sentinel value that controls the loop.
Note that the entire program has been written for you. You need to add code that validates correct input, which is "Y" or "N", when the user is asked if he or she wants to guess a number, and a number in the range of 1 through 10 when the user is asked to guess a number.
Instructions
-
Ensure the file named GuessNumber.java is open.
-
Write loops that validate input at all places in the code where the user is asked to provide input. Comments have been included in the code to help you identify where these loops should be written.
-
Execute the program by clicking "Run Code." See if you can guess the randomly generated number. Execute the program several times to see if the random number changes. Also, test the program to verify that incorrect input is handled correctly. On your best attempt, how many guesses did you have to take to guess the correct number?



Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

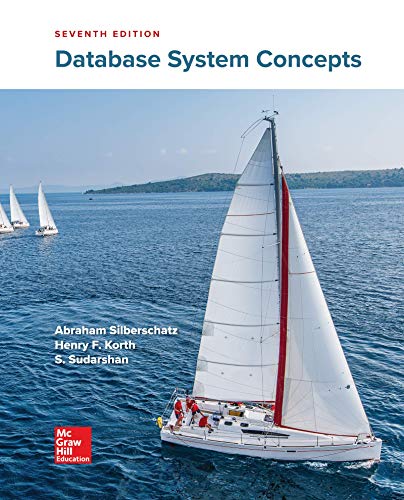
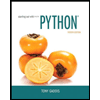
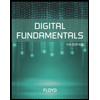
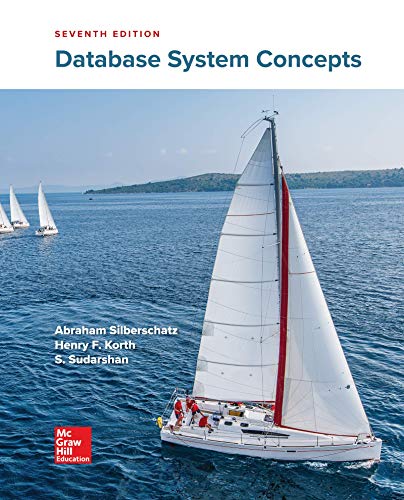
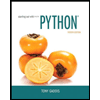
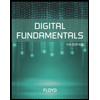
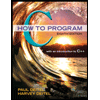
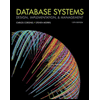
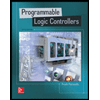