InheritanceDemo.java: // StudentName Today's Date public class InheritanceDemo { public static void main(String[] args) { KleenexBox kb1 = new KleenexBox(); KleenexBox kb2 = new KleenexBox(100); Box justABox = new Box(); System.out.println("justABox has area " + BoxArea(justABox)); System.out.println("kb1 has area " + BoxArea(kb1)); System.out.println("kb2 has this many square inches of tissue: " + KleenexArea(kb2)); } public static double BoxArea (Box b) { return b.width * b.length; } public static double KleenexArea (KleenexBox a) { return a.width * a.length * a.numTissues; } } Box.java: // Student Name Today's Date public class Box { public double width; public double length; public String label; } KleenexBox.java: // Student's Name Today's Date public class KleenexBox extends Box { public int numTissues; // A new Kleenex box public KleenexBox() { numTissues = 50; length = 8.0; width = 5.0; } // A new Kleenex box with a specific number of tissues public KleenexBox(int numberOfTissues) { numTissues = numberOfTissues; length = 8.0; width = 5.0; } public String toString() { return ("This box is " + length + " by " + width + " and has " + numTissues + " tissues."); } } Questions: (One points each) 5.1. In the relationship between Box and KleenexBox: which is the superclass? 5.2. In the relationship between Box and KleenexBox: which is the subclass? 5.3. Why can't BoxArea use b.numTissues? (Hint: if you try, Java will give you an error.) 5.4. Why is the programmer able to pass both justABox and kb1 as arguments to boxArea? (Hint: one word, starts with P.) 5.5. Why can't the programmer pass justABox as an argument to kleenexArea?
InheritanceDemo.java:
// StudentName Today's Date
public class InheritanceDemo {
public static void main(String[] args) {
KleenexBox kb1 = new KleenexBox();
KleenexBox kb2 = new KleenexBox(100);
Box justABox = new Box();
System.out.println("justABox has area " + BoxArea(justABox));
System.out.println("kb1 has area " + BoxArea(kb1));
System.out.println("kb2 has this many square inches of tissue: " + KleenexArea(kb2));
}
public static double BoxArea (Box b) {
return b.width * b.length;
}
public static double KleenexArea (KleenexBox a) {
return a.width * a.length * a.numTissues;
}
}
Box.java: // Student Name Today's Date
public class Box {
public double width;
public double length;
public String label;
}
KleenexBox.java: // Student's Name Today's Date public class KleenexBox extends Box {
public int numTissues;
// A new Kleenex box
public KleenexBox() {
numTissues = 50;
length = 8.0;
width = 5.0;
}
// A new Kleenex box with a specific number of tissues
public KleenexBox(int numberOfTissues) {
numTissues = numberOfTissues;
length = 8.0;
width = 5.0;
}
public String toString() {
return ("This box is " + length + " by " + width + " and has " + numTissues + " tissues.");
}
}
Questions: (One points each)
5.1. In the relationship between Box and KleenexBox: which is the superclass?
5.2. In the relationship between Box and KleenexBox: which is the subclass?
5.3. Why can't BoxArea use b.numTissues? (Hint: if you try, Java will give you an error.)
5.4. Why is the programmer able to pass both justABox and kb1 as arguments to boxArea? (Hint: one word, starts with P.)
5.5. Why can't the programmer pass justABox as an argument to kleenexArea?
Please paste a screenshot of a successful program run, and copy-and-paste the source code from your.java files, here:

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

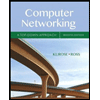
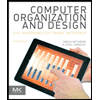
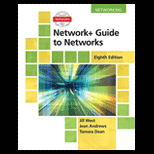
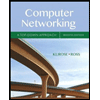
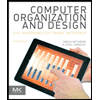
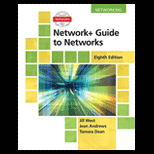
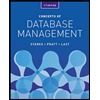
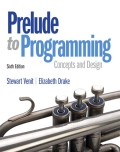
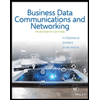