import java.util.Scanner; public class Shady RestRoom 2 { // Modify the code below public static void main (String args[]) { int selection; int price; String result; final int QUEEN = 1, KING = 2, SUITE = 3; final int QPRICE = 125, KPRICE = 139, SPRICE = 165; final String QSTRING = "Queen bed", KSTRING = "King bed"; SSTRING = "Suite with a king bed and pull-out couch"; INVALIDSTRING = "an invalid option"; Scanner in = new Scanner(System.in); System.out.println("\t\n\nMenu\n"); System.out.println("(" + QUEEN + ") " + QSTRING); System.out.println("(" + KING + ") " + KSTRING); System.out.println("(" + SUITE + ") " + SSTRING); System.out.print("Enter Selection (1, 2, or 3) >> "); selection = in.nextInt(); if(selection == QUEEN) { result = QSTRING; price = QPRICE; } else if(selection == KING) { result = KSTRING; price = KPRICE; } else if (selection == SUITE) { result = SSTRING; price = SPRICE; } else { result = INVALIDSTRING; price = 0; } System.out.println("You selected " + result + " $" + price); } }
import java.util.Scanner;
public class Shady RestRoom 2
{ // Modify the code below public static void main (String args[])
{ int selection; int price; String result; final int QUEEN = 1, KING = 2, SUITE = 3; final int QPRICE = 125, KPRICE = 139, SPRICE = 165; final String QSTRING = "Queen bed", KSTRING = "King bed"; SSTRING = "Suite with a king bed and pull-out couch"; INVALIDSTRING = "an invalid option"; Scanner in = new Scanner(System.in); System.out.println("\t\n\nMenu\n"); System.out.println("(" + QUEEN + ") " + QSTRING); System.out.println("(" + KING + ") " + KSTRING); System.out.println("(" + SUITE + ") " + SSTRING); System.out.print("Enter Selection (1, 2, or 3) >> "); selection = in.nextInt(); if(selection == QUEEN) { result = QSTRING; price = QPRICE; } else if(selection == KING) { result = KSTRING; price = KPRICE; } else if (selection == SUITE) { result = SSTRING; price = SPRICE; } else { result = INVALIDSTRING; price = 0; } System.out.println("You selected " + result + " $" + price); } }

Step by step
Solved in 2 steps with 11 images

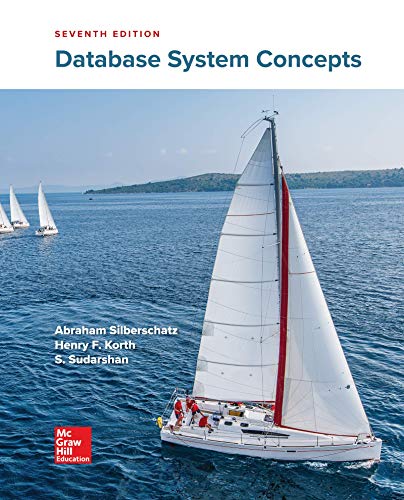
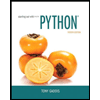
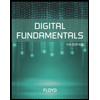
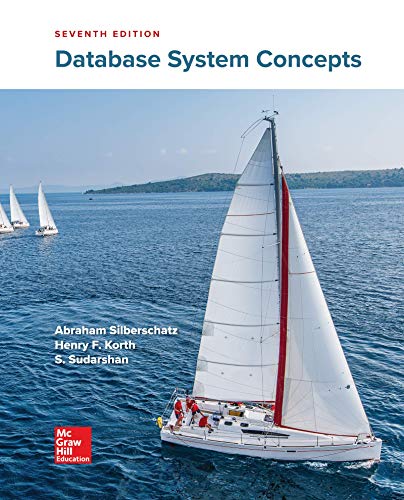
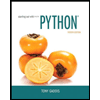
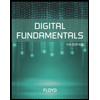
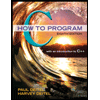
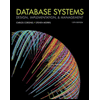
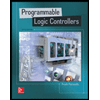