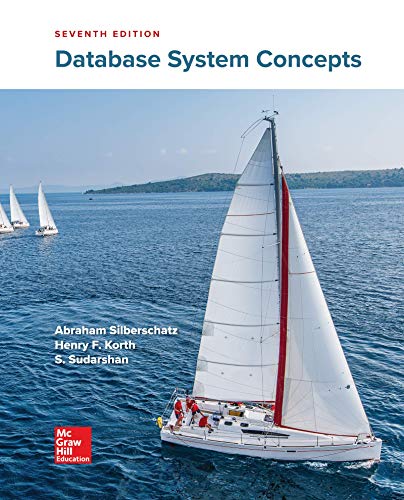
Concept explainers
JAVA Language
Caesar Shift
Question:
import java.util.*;
public class TestCipher {
public static void main(String[] args) {
int shift = 7;
Caesar caesar = new Caesar();
String text = "hello world";
String encryptTxt = caesar.encrypt(text);
System.out.println(text + " encrypted with shift " + shift + " is " + encryptTxt);
}
}
abstract class Cipher {
public String encrypt(String s) {
StringBuffer result = new StringBuffer(""); // Use a StringBuffer
StringTokenizer words = new StringTokenizer(s); // Break s into its words
while (words.hasMoreTokens()) { // For each word in s
result.append(encode(words.nextToken()) + " "); // Encode it
}
return result.toString(); // Return the result
} // encrypt()
public String decrypt(String s) {
StringBuffer result = new StringBuffer(""); // Use a StringBuffer
StringTokenizer words = new StringTokenizer(s); // Break s into words
while (words.hasMoreTokens()) { // For each word in s
result.append(decode(words.nextToken()) + " "); // Decode it
}
return result.toString(); // Return the decryption
} // decrypt()
public abstract String encode(String word); // Abstract methods
public abstract String decode(String word);
} // Cipher
class Caesar extends Cipher {
public String encode(String word) {
StringBuffer result = new StringBuffer(); // Initialize a string buffer
for (int k = 0; k < word.length(); k++) { // For each character in word
char ch = word.charAt(k); // Get the character
ch = (char)('a' + (ch -'a'+ 3) % 26); // Perform caesar shift
result.append(ch); // Append it to new string
}
return result.toString(); // Return the result as a string
} // encode()
public String decode(String word) {
StringBuffer result = new StringBuffer(); // Initialize a string buffer
for (int k = 0; k < word.length(); k++) { // For each character in word
char ch = word.charAt(k); // Get the character
ch = (char)('a' + (ch - 'a' + 23) % 26); // Perform reverse shift
result.append(ch); // Append it to new string
}
return result.toString(); // Return the result as a string
} // decode()
} // Caesar

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 3 images

- See ERROR in Java file 2: Java file 1: import java.util.Random;import java.util.Scanner; public class Account {// Declare the variablesprivate String customerName;private String accountNumber;private double balance;private int type;private static int numObjects;// constructorpublic Account(String customerName, double balance, int type) { this.customerName = customerName;this.balance = balance;this.type = type;setaccountNumber(); // set account number functionnumObjects += 1;}private void setaccountNumber() // definition of set account number{Random rand = new Random();accountNumber = customerName.substring(0, 3).toUpperCase();accountNumber += type;accountNumber += "#";accountNumber += (rand.nextInt(100) + 100);}// function to makedepositvoid makeDeposit(double amount){if (amount > 0){balance += amount;}}// function for withdrawboolean makeWithdrawal(double amount) {if (amount < balance)balance -= amount;elsereturn false;return true;}public String toString(){return accountNumber +…arrow_forwardJAVA Practice Question for Midterm Write a class with a constructor that accepts a String object as its argument. The class should have a method that returns the number of vowels in the string, and another method that returns the number of consonants in the string. Demonstrate the class in a program by invoking the methods that return the number of vowels and consonants. Print the counts returned. Imagine you are developing a software package for an online shopping site that requires users to enter their own passwords. Your software requires that users’ passwords meet the following criteria: The password should be at least six characters long. The password should contain at least one uppercase and at least one lowercase letter. The password should have at least one digit. a program that asks the user to enter a password, then displays a message indicating whether it is valid or not.arrow_forward1. Insert the missing part of the code below to output the string. public class MyClass { public static void main(String[] args) { ("Hello, I am a Computer Engineer");arrow_forward
- Fibonacci A fibonacci sequence is a series of numbers in which each number is the sum of the two preceding numbers. For example: 0, 1, 1, 2, 3, 5, 8, 13, 21, 34, and so on… For this, you will implement a class that takes a positive integer (n) and returns the number in the nth position in the sequence. Examples When n is 1, the returned value will be 0. When n is 4, the returned value will be 2. When n is 9, the returned value will be 21. come up with the formula and base cases Implementation Create a class Fibonacci with a public static method getValue. Create a Main class to test and run your Fibonacci class.arrow_forwardAg 1- Random Prime Generator Add a new method to the Primes class called genRandPrime. It should take as input two int values: 1owerBound and upperBound. It should return a random prime number in between TowerBound (inclusive) and upperBound (exclusive) values. Test the functionality inside the main method. Implementation steps: a) Start by adding the method header. Remember to start with public static keywords, then the return type, method name, formal parameter list in parenthesis, and open brace. The return type will be int. We will have two formal parameters, so separate those by a comma. b) Now, add the method body code that generates a random prime number in the specified range. The simplest way to do this is to just keep trying different random numbers in the range, until we get one that is a prime. So, generate a random int using: int randNum = lowerBound + gen.nextInt(upperBound); Then enter put a while loop that will keep going while randNum is not a prime number - you can…arrow_forwardcorrect the codearrow_forward
- x = 3 + numbers[3]++; System.out.println(x); QUESTION 2: Class Rectangle has two data members, length (type of float) and width (type of float). The class Rectangle also has the following: //mutator methods void setlength (float len) } //B. length = len; H accessor methods float getLength() I return length; The following statement creates an array of Rectangle with size 2: Rectangle[] arrayRectangle= new Rectangle[2]; Is it correct if executing the following statements? What is the output? If it is not correct, write the correct one arrayRectangle. setLength (12.59); System.out.printin[rectangle Set.getLength()); arrayRectangle[1].setLength(4.15); System.out.println(arrayRectangle[1].getLength()): QUESTION 3: Use the following int array int[] numbers = { 42, 28, 36, 72, 17, 25, 81, 65, 23, 58} -Open file data.txt to write. to write the values of the array numbers to the file with the y numbers to get the valuesarrow_forwardcomplete the missing code. public class Exercise09_04Extra { public static void main(String[] args) { SimpleTime time = new SimpleTime(); time.hour = 2; time.minute = 3; time.second = 4; System.out.println("Hour: " + time.hour + " Minute: " + time.minute + " Second: " + time.second); } } class SimpleTime { // Complete the code to declare the data fields hour, // minute, and second of the int type }arrow_forwardDiscuss the challenges and best practices associated with managing a fleet of printers in a large organization.arrow_forward
- public class Animal { public static int population; private int age; } public Animal (int age) { this.age = age; population++; } + msg); public void say (String msg) { System.out.print("Saying: " System.out.print(" for "); System.out.print(getHuman Years()); System.out.println(" years."); } public int getHuman Years() { return age; } public class Mammal extends Animal { private String species; public Mammal(String species, int age) { super (age); this. species species; } } } = public int getHuman Years() { if (species.equals("dog")) 1 else return super.getHumanYears() * 7; return super.getHumanYears(); €arrow_forwardMath 130 Java programming Dear Bartleby, I am a student beginning in computer science. May I have some assistance on this lab assignment please? Thank you.arrow_forwardInstructions-Java Assignment is to define a class named Address. The Address class will have three private instance variables: an int named street_number a String named street_name and a String named state. Write three constructors for the Address class: an empty constructor (no input parameters) that initializes the three instance variables with default values of your choice, a constructor that takes the street values as input but defaults the state to "Arizona", and a constructor that takes all three pieces of information as input Next create a driver class named Main.java. Put public static void main here and test out your class by creating three instances of Address, one using each of the constructors. You can choose the particular address values that are used. I recommend you make them up and do not use actual addresses. Run your code to make sure it works. Next add the following public methods to the Address class and test them from main as you go: Write getters and…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
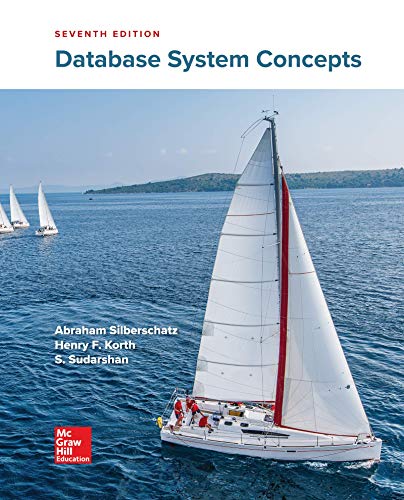
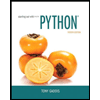
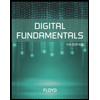
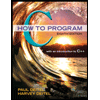
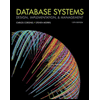
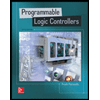