/* TestCarSensor.java - program to test the CarSensor class. */ public class TestCarSensor { public static void main (String[] args) { CarSensor generic = new CarSensor(); CarSensor tempCel = new CarSensor("temperature sensor", -50, +300, "C"); CarSensor speed = new CarSensor("speed sensor", 0, 200, "km/h"); CarSensor speed2 = new CarSensor("speed sensor 2", 0, 200, "m/h"); // 2. test changing desc and limits System.out.println (); System.out.println ( generic ); // display generic sensor (zero) generic.setDesc ("special sensor"); // change description generic.setLimits (-5,5,"units"); // change limits System.out.println ( generic ); // display generic sensor again // 3. test displaying object (calling .toString() ) System.out.println (); System.out.println ( tempCel ); System.out.println ( speed ); System.out.println ( speed2 ); // 4. test setlimits() rule System.out.println (); System.out.println ( generic ); generic.setLimits (10, -10, "blah"); System.out.println ( generic ); generic.setLimits (-10, 10, "blah"); System.out.println ( generic ); generic.setLimits (-5,5,"units"); System.out.println (); if ( speed.equalsLimits(speed2) ) { System.out.println ( speed + "\n = \n" + speed2 ); } else { System.out.println ( speed + "\n != \n" + speed2 ); } if ( tempCel.equalsLimits(generic) ) { System.out.println ( tempCel + "\n = \n" + generic ); } else { System.out.println ( tempCel + "\n != \n" + generic ); } System.out.println (); // 6. test changing current value System.out.println (); speed.setCurrentValue(22); // set current value System.out.println ( speed ); // is the current value 22? speed.setCurrentValue(2000); // set current value System.out.println ( speed ); // is the current value still 22? (unchanged) System.out.println (); // 7. test .equals() System.out.println (); System.out.println (" speed = speed " + speed.equals(speed) ); // should be true System.out.println (" speed = speed2 " + speed.equals(speed2) ); // should be false System.out.println (); // displaying error range System.out.println ( tempCel ); tempCel.setCurrentValue ( 25, 5 ); System.out.println ( tempCel ); System.out.println ( tempCel.getErrorDesc() ); System.out.println (); }// end of main() }// end of class ** below is the CarSensor class, or what could be rescued from the damaged .java file /* CarSensor.java - a class describing any sensor in the "smart" car project. public class CarSensor { private String desc="none"; // general description of sensor; simple text private int limitLow=0; // range of values for sensor; lower limit private int limitUp=0; // range of values for sensor; upper limit // rule: limitLow <= limitUp (ensured by setLimits() below) private String scale = "noscale"; // the "scale" of the sensor (C, F, km, km/h, kg/m, etc.) private int currVal=0; // current value of sensor private error=0; // sensor error around current value: +/- error // rule: limitLow <= currVal <= limitUp //---------------------- // default constructor - set default values; nothing to do public CarSensor () { } // constructor - set attributes to parameter values private CarSensor (String desc, int low, int upper, String scale) { setDesc(desc); setLimits (low, upper, scale); // set limits and scale, using rule } // setDesc() - set the description public void setDesc(String desc) { desc = desc; } // setLimits() - set the low and upper limit and scale attributes, but only if rule is true public void setLimits (int low, int upper, String scale) { if (low <= upper) // if rule is true, set lower & upper limits, and scale { limitLow = low; limitUp = upper; this.scale = scale; } // otherwise leave as-is } // setCurrentValue() - sets the current value, but only if rule is true public void setCurrentValue (int current) { setCurrentValue (current, error); // set current, using existing error value } // setCurrentValue() - sets the current value and error, but only if rule is true public void setCurrentValue (double current, double err) { if (limitLow <= current && current <= limitUp) // if rule is true, set current value { currVal = current; error = err; } } // getCurrentValue() - return the current value public double getCurrentValue () { return currVal; } // equals() - check equality with this sensor and another sensor; only check currVal public boolean equals (CarSensor other) { return ( this.currVal == other.currVal ); } // equalsLimits() - check equality with this sensor and another sensor; only check limits public boolean equalsLimits (CarSensor other) { return ( this.limitLow == == other.limitUp ); } // getErrorDesc() - return a String of the error description; ex: "Error range: mm to nn" getErrorDesc () { return String.format ("error range: %d to %d", (currVal-error), (currVal+error) ); } // toString() - return a formatted version of the sensor attributes // format: " desc limits: limitLow to limitUp scale, current value:, error range: " public String toString () { String ret = ""; ret += desc; ret += String.format (" limits: %d to %d %s,", limitLow, limitUp, scale ); ret += String.format (" current value: %f, ", currVal ); ret = getErrorDesc; return ; } // end of class
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
/* TestCarSensor.java - program to test the CarSensor class.
*/
public class TestCarSensor
{
public static void main (String[] args)
{
CarSensor generic = new CarSensor();
CarSensor tempCel = new CarSensor("temperature sensor", -50, +300, "C");
CarSensor speed = new CarSensor("speed sensor", 0, 200, "km/h");
CarSensor speed2 = new CarSensor("speed sensor 2", 0, 200, "m/h");
// 2. test changing desc and limits
System.out.println ();
System.out.println ( generic ); // display generic sensor (zero)
generic.setDesc ("special sensor"); // change description
generic.setLimits (-5,5,"units"); // change limits
System.out.println ( generic ); // display generic sensor again
// 3. test displaying object (calling .toString() )
System.out.println ();
System.out.println ( tempCel );
System.out.println ( speed );
System.out.println ( speed2 );
// 4. test setlimits() rule
System.out.println ();
System.out.println ( generic );
generic.setLimits (10, -10, "blah");
System.out.println ( generic );
generic.setLimits (-10, 10, "blah");
System.out.println ( generic );
generic.setLimits (-5,5,"units");
System.out.println ();
if ( speed.equalsLimits(speed2) )
{
System.out.println ( speed + "\n = \n" + speed2 );
}
else
{
System.out.println ( speed + "\n != \n" + speed2 );
}
if ( tempCel.equalsLimits(generic) )
{
System.out.println ( tempCel + "\n = \n" + generic );
}
else
{
System.out.println ( tempCel + "\n != \n" + generic );
}
System.out.println ();
// 6. test changing current value
System.out.println ();
speed.setCurrentValue(22); // set current value
System.out.println ( speed ); // is the current value 22?
speed.setCurrentValue(2000); // set current value
System.out.println ( speed ); // is the current value still 22? (unchanged)
System.out.println ();
// 7. test .equals()
System.out.println ();
System.out.println (" speed = speed " + speed.equals(speed) ); // should be true
System.out.println (" speed = speed2 " + speed.equals(speed2) ); // should be false
System.out.println ();
// displaying error range
System.out.println ( tempCel );
tempCel.setCurrentValue ( 25, 5 );
System.out.println ( tempCel );
System.out.println ( tempCel.getErrorDesc() );
System.out.println ();
}// end of main()
}// end of class
** below is the CarSensor class, or what could be rescued from the damaged .java file
/* CarSensor.java - a class describing any sensor in the "smart" car project.
public class CarSensor
{
private String desc="none"; // general description of sensor; simple text
private int limitLow=0; // range of values for sensor; lower limit
private int limitUp=0; // range of values for sensor; upper limit
// rule: limitLow <= limitUp (ensured by setLimits() below)
private String scale = "noscale"; // the "scale" of the sensor (C, F, km, km/h, kg/m, etc.)
private int currVal=0; // current value of sensor
private error=0; // sensor error around current value: +/- error
// rule: limitLow <= currVal <= limitUp
//----------------------
// default constructor - set default values; nothing to do
public CarSensor ()
{
}
// constructor - set attributes to parameter values
private CarSensor (String desc, int low, int upper, String scale)
{
setDesc(desc);
setLimits (low, upper, scale); // set limits and scale, using rule
}
// setDesc() - set the description
public void setDesc(String desc)
{
desc = desc;
}
// setLimits() - set the low and upper limit and scale attributes, but only if rule is true
public void setLimits (int low, int upper, String scale)
{
if (low <= upper) // if rule is true, set lower & upper limits, and scale
{
limitLow = low;
limitUp = upper;
this.scale = scale;
} // otherwise leave as-is
}
// setCurrentValue() - sets the current value, but only if rule is true
public void setCurrentValue (int current)
{
setCurrentValue (current, error); // set current, using existing error value
}
// setCurrentValue() - sets the current value and error, but only if rule is true
public void setCurrentValue (double current, double err)
{
if (limitLow <= current && current <= limitUp) // if rule is true, set current value
{
currVal = current;
error = err;
}
}
// getCurrentValue() - return the current value
public double getCurrentValue ()
{
return currVal;
}
// equals() - check equality with this sensor and another sensor; only check currVal
public boolean equals (CarSensor other)
{
return ( this.currVal == other.currVal );
}
// equalsLimits() - check equality with this sensor and another sensor; only check limits
public boolean equalsLimits (CarSensor other)
{
return ( this.limitLow == == other.limitUp );
}
// getErrorDesc() - return a String of the error description; ex: "Error range: mm to nn"
getErrorDesc ()
{
return String.format ("error range: %d to %d", (currVal-error), (currVal+error) );
}
// toString() - return a formatted version of the sensor attributes
// format: " desc limits: limitLow to limitUp scale, current value:, error range: "
public String toString ()
{
String ret = "";
ret += desc;
ret += String.format (" limits: %d to %d %s,", limitLow, limitUp, scale );
ret += String.format (" current value: %f, ", currVal );
ret = getErrorDesc;
return ;
}
// end of class



Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

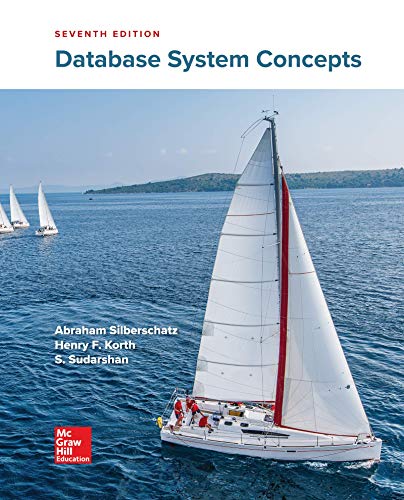
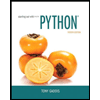
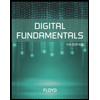
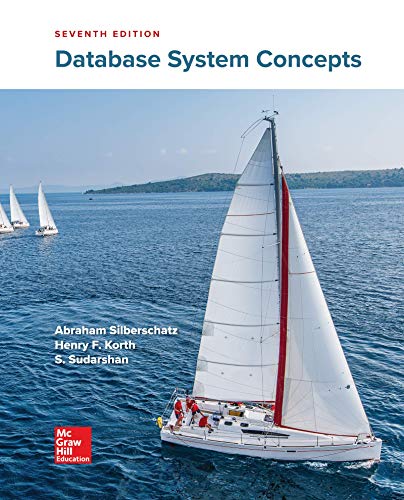
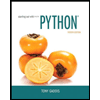
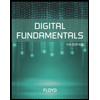
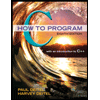
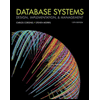
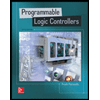