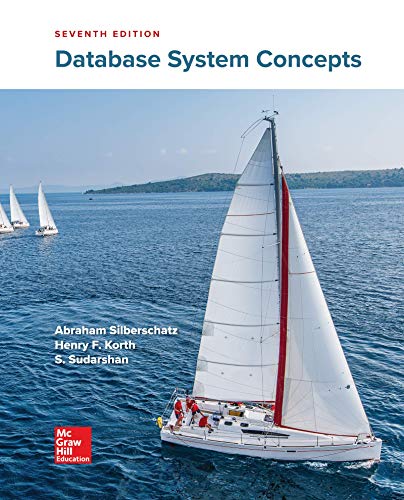
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
package citytech.calculator; public class Calculator { public static void main(String[]args) { System.out.println("Basic Calculator"); //declaring variable: //dataType variableName = value ; double num1 = 10.0; double num2 = 30.0; sum(num1, num2); multiply(num1, num2); } public static void sum(double n1, double n2) { double result = n1 + n2; System.out.println("Sum: "+result); } public static void multiply(double n1, double n2) { double result = n1 * n2; System.out.println("Multiply: "+result); } }
Question: Please Check the Calculator app and add subtract and division methods
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- using System; class Program { publicstaticvoid Main(string[] args) { int number = 1; while (number <= 88) { Console.WriteLine(number); number = number + 2; } int[] randNo = newint[88]; Random r = new Random(); int i=0; while (number <= 88) { randNo[i] = number; number+=1; i+=1; } for (i = 0; i < 3; i++) { Console.WriteLine("Random Numbers between 1 and 88 are " + randNo[r.Next(1, 88)]); } } } this code counts from 1-88 in odds and then selects three different random numbers. it keeps choosing 0 as a random number everytime. how can that be fixed?arrow_forwardclass temporary { public: void set(string, double, double); void print(); double manipulate(); void get(string&, double&, double&); void setDescription(string); void setFirst(double); void setSecond(double); string getDescription() const; double getFirst()const; double getSecond()const; temporary(string = "", double = 0.0, double = 0.0); private: string description; double first; double second; }; I need help writing the definition of the member function set so the instance varialbes are set according to the parameters. I also need help in writing the definition of the member function manipulation that returns a decimal with: the value of the description as "rectangle", returns first * second; if the value of description is "circle", it returns the area of the circle with radius first; if the value of the description is "cylinder", it returns the volume of the cylinder with radius first and height second; otherwise, it returns with the value -1.arrow_forwardComplete the isExact Reverse() method in Reverse.java as follows: The method takes two Strings x and y as parameters and returns a boolean. The method determines if the String x is the exact reverse of the String y. If x is the exact reverse of y, the method returns true, otherwise, the method returns false. Other than uncommenting the code, do not modify the main method in Reverse.java. Sample runs provided below. Argument String x "ba" "desserts" "apple" "regal" "war" "pal" Argument String y "stressed" "apple" "lager" "raw" "slap" Return Value false true false true true falsearrow_forward
- Fix all logic and syntax errors. Results Circle statistics area is 498.75924968391547 diameter is 25.2 // Some circle statistics public class DebugFour2 { public static void main(String args[]) { double radius = 12.6; System.out.println("Circle statistics"); double area = java.Math.PI * radius * radius; System.out.println("area is " + area); double diameter = 2 - radius; System.out.println("diameter is + diameter); } }arrow_forward1 public class Main { 1234567 7 8 9 10 11 ENERGET 12 13 14 15 16 17 18} public static void main(String[] args) { String present = ""; double r = Math.random(); // between 0 and 1 if (r > >>arrow_forwardpublic class Turn{public static void main(String[] args){// Different initial values will be used for testingint x = 1; int y = 1; // Turn the arrow by 90 degrees System.out.print("x:", y + 90);System.out.println(x);System.out.print("y: ", x - 1 );System.out.println(y); // Turn the arrow again System.out.print("x:", y - 1);System.out.println(x);System.out.print("y: ",x + 90);System.out.println(y);}}arrow_forward
- class Artist{StringsArtist(const std::string& name="",int age=0):name_(name),age_(age){}std::string name()const {return name_;}void set_name(const std::string& name){name_=name;}int age()const {return age_;}void set_age(int age){age_=age;}virtual std::string perform(){return std::string("");}private:int age_;std::string name_;};class Singer : public Artist{public:Singer():Artist(){};Singer(const std::string& name,int age,int hits);int hits() const{return hits_;}void set_hits(int hit);std::string perform();int operator+(const Singer& rhs);int changeme(std::string name,int age,int hits);Singer combine(const Singer& rhs);private:int hits_=0;};int FindOldestandMostSuccess(std::vector<Singer> list); Task: Implement the function int Singer::changeme(std::string name,int age,int hits) : This function should check if the values passed by name, age and hits are different than those stored. And if this is the case it should change the values. This should be done by…arrow_forwardint stop = 6; int num =6; int count=0; for(int i = stop; i >0; i-=2) { num += i; count++; } System.out.println("num = "+ num); System.out.println("count = "+ count); } }arrow_forwardJAVA CODE: check outputarrow_forward
- class Artist{StringsArtist(const std::string& name="",int age=0):name_(name),age_(age){}std::string name()const {return name_;}void set_name(const std::string& name){name_=name;}int age()const {return age_;}void set_age(int age){age_=age;}virtual std::string perform(){return std::string("");}private:int age_;std::string name_;};class Singer : public Artist{public:Singer():Artist(){};Singer(const std::string& name,int age,int hits);int hits() const{return hits_;}void set_hits(int hit);std::string perform();int operator+(const Singer& rhs);int changeme(std::string name,int age,int hits);Singer combine(const Singer& rhs);private:int hits_=0;};int FindOldestandMostSuccess(std::vector<Singer> list); Implement the function Singer Singer::combine(const Singer& rhs):It should create a new Singer object, by calling the constructor with the following values: For name it should pass a combination of be the name of the calling object followed by a '+' and then…arrow_forwardFix all errors to make the code compile and complete. //MainValidatorA3 public class MainA3 { public static void main(String[] args) { System.out.println("Welcome to the Validation Tester application"); // Int Test System.out.println("Int Test"); ValidatorNumeric intValidator = new ValidatorNumeric("Enter an integer between -100 and 100: ", -100, 100); int num = intValidator.getIntWithinRange(); System.out.println("You entered: " + num + "\n"); // Double Test System.out.println("Double Test"); ValidatorNumeric doubleValidator = new ValidatorNumeric("Enter a double value: "); double dbl = doubleValidator.getDoubleWithinRange(); System.out.println("You entered: " + dbl + "\n"); // Required String Test System.out.println("Required String Test:"); ValidatorString stringValidator = new ValidatorString("Enter a required string: "); String requiredString =…arrow_forwardpublic class Side Plot { * public static String PLOT_CHAR = "*"; public static void main(String[] args) { plotxSquared (-6,6); * plotNegXSquaredPlus20 (-5,5); plotAbsXplus1 (-4,4); plotSinWave (-9,9); public static void plotXSquared (int minX, int maxx) { System.out.println("Sideways Plot"); System.out.println("y = x*x where + minX + "= minX; row--) { for (int col = 0; col row * row; col++) { System.out.print (PLOT_CHAR); if (row== 0){ } System.out.print(" "); } for(int i = 0; i <= maxx* maxX; i++) { System.out.print("-"); } System.out.println(); Sideways PHOT y = x*x where -6<=x<=6 Sideways Plot y = x*x where -6<=x<=6 How do I fix my code to get a plot like 3rd picture?arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
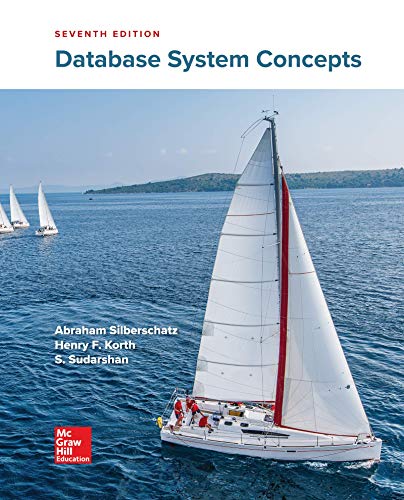
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
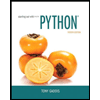
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
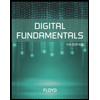
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
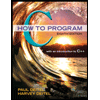
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
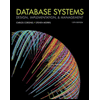
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
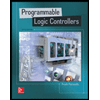
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education