Fix this program import java.util.Scanner; public class main { public static void WeShipItBad(String[] args) { int OVERNIGHT_CHARGE = 5; int TWO_DAY_CHARGE = 2; int ECONOMY_CHARGE = 1; Scanner console = new Scanner(System.in); // Scanner object to pass around. // Get item description. String itemDescription = getItemDescription(console); if (itemDescription.equals("")) { System.out.println("Invalid description."); } shipWeight = getShipWeight(scnr); if (shipWeight <= 0.0) { System.out.println(); System.out.println("Invalid weight."); } char shipMethod = getShipMethod(council); shipMethod = Character.toUpperCase(shipMethod); double shipCost = calculateShip(shipWeight, shipMethod); displayResults(itemDescription, shipCost, shipMethod, shipWeight); } public static void getItemDescription(Scanner console) { System.out.println("Enter item description:"); String description = keyboard.nextLine(); return description; } public static double getShipWeight(Scanner console) { System.out.print("Enter item weight (in lbs): "); int weight = consoal.nextInt(); return weight; } // Prompts the user for the shipping method and returns it. public static char getShipMethod(Scanner console) { char method; System.out.print("Enter ship method (O)vernight - (T)wo Days - (E)conomy: "); method = console.next().charAt(0); // I've been told to leave this line in here. // Will be explained in class. return method; } // Calculates and returns the shipping charge. public static double calculateShipping(char method, double weight) { double shipCharge = 0.0; if (method == 'o') { shipCharge = weight * 5; } else if (method == 'T') { shipCharge = weight * 3; } else shipCharge = weight * 2; return shipCharge; } // Print shipping charge invoice. public static void displayResults(String description, float weight, char method, double cost) { System.out.println("*** WE SHIP INVOICE ****"); System.out.println("Item Description: ", description); System.out.printf("Item Weight: %.3f\n", cost); System.out.println("Ship Method: " + method); System.out.printf("Total Cost: $%.1f\n" + cost); System.out.println(); System.out.print("Like this program? Hire me!! "); System.out.println("Email: nadhaSkolar@shouldHaveGoneToVanderbilt.edu"); } }
Fix this program
import java.util.Scanner;
public class main {
public static void WeShipItBad(String[] args) {
int OVERNIGHT_CHARGE = 5;
int TWO_DAY_CHARGE = 2;
int ECONOMY_CHARGE = 1;
Scanner console = new Scanner(System.in); // Scanner object to pass around.
// Get item description.
String itemDescription = getItemDescription(console);
if (itemDescription.equals("")) {
System.out.println("Invalid description.");
}
shipWeight = getShipWeight(scnr);
if (shipWeight <= 0.0) {
System.out.println();
System.out.println("Invalid weight.");
}
char shipMethod = getShipMethod(council);
shipMethod = Character.toUpperCase(shipMethod);
double shipCost = calculateShip(shipWeight, shipMethod);
displayResults(itemDescription, shipCost, shipMethod, shipWeight);
}
public static void getItemDescription(Scanner console) {
System.out.println("Enter item description:");
String description = keyboard.nextLine();
return description;
}
public static double getShipWeight(Scanner console) {
System.out.print("Enter item weight (in lbs): ");
int weight = consoal.nextInt();
return weight;
}
// Prompts the user for the shipping method and returns it.
public static char getShipMethod(Scanner console) {
char method;
System.out.print("Enter ship method (O)vernight - (T)wo Days - (E)conomy: ");
method = console.next().charAt(0); // I've been told to leave this line in here.
// Will be explained in class.
return method;
}
// Calculates and returns the shipping charge.
public static double calculateShipping(char method, double weight) {
double shipCharge = 0.0;
if (method == 'o') {
shipCharge = weight * 5;
} else if (method == 'T') {
shipCharge = weight * 3;
} else
shipCharge = weight * 2;
return shipCharge;
}
// Print shipping charge invoice.
public static void displayResults(String description, float weight, char method, double cost) {
System.out.println("*** WE SHIP INVOICE ****");
System.out.println("Item Description: ", description);
System.out.printf("Item Weight: %.3f\n", cost);
System.out.println("Ship Method: " + method);
System.out.printf("Total Cost: $%.1f\n" + cost);
System.out.println();
System.out.print("Like this program? Hire me!! ");
System.out.println("Email: nadhaSkolar@shouldHaveGoneToVanderbilt.edu");
}
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

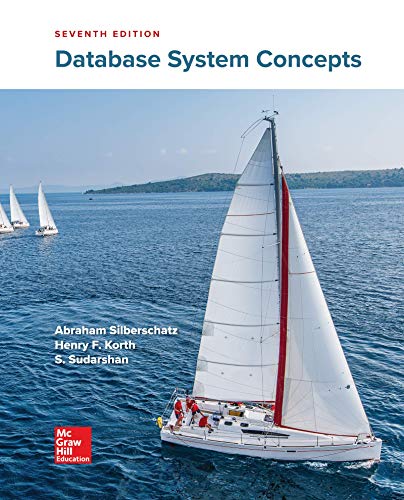
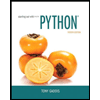
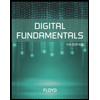
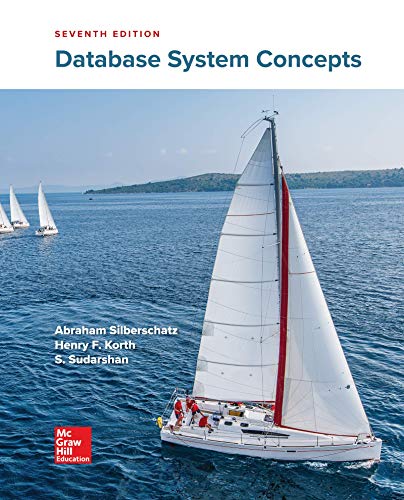
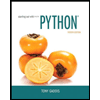
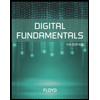
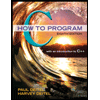
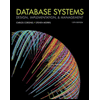
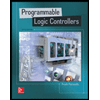