import java.util.Scanner; public class WinningTeam { public static void main(String[] args) { Scanner scnr = new Scanner(System.in); Team team = new Team(); String name = scnr.next(); int wins = scnr.nextInt(); int losses = scnr.nextInt(); team.setName(name); team.setWins(wins); team.setLosses(losses); team.printStanding(); } } This is the second part of the program and not geting it to run public class Team { // TODO: Declare private fields - name, wins, losses private String name; private int wins; private int losses; public int getlosses(){ return losses; } // TODO: Define mutator methods - // setName(), setWins(), setLosses() public String setName(){//String name int wins int losses return name; } public int setWins(){ return wins; } public int setLosses(){ return losses; } // TODO: Define accessor methods - // getName(), getWins(), getLosses() public String getName(){ return name; } public int getWins(){ return wins; } public int getLosses(){ return losses; } // TODO: Define getWinPercentage() public double getWinPercentage(){ return ((double)wins)/(wins + losses); } // TODO: Define printStanding() public void printStanding(){ double winPercentage; winPercentage = getWinPercentage(); System.out.printf("Win percentage: %.2f\n", winPercentage); if (winPercentage>= 0.5) { System.out.println("Congratulations, Team " + name + " has a winning average!"); } else{ System.out.println("Team " + name + " has a losing average."); } } }
import java.util.Scanner;
public class WinningTeam {
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
Team team = new Team();
String name = scnr.next();
int wins = scnr.nextInt();
int losses = scnr.nextInt();
team.setName(name);
team.setWins(wins);
team.setLosses(losses);
team.printStanding();
}
}
This is the second part of the program and not geting it to run
public class Team {
// TODO: Declare private fields - name, wins, losses
private String name;
private int wins;
private int losses;
public int getlosses(){
return losses;
}
// TODO: Define mutator methods -
// setName(), setWins(), setLosses()
public String setName(){//String name int wins int losses
return name;
}
public int setWins(){
return wins;
}
public int setLosses(){
return losses;
}
// TODO: Define accessor methods -
// getName(), getWins(), getLosses()
public String getName(){
return name;
}
public int getWins(){
return wins;
}
public int getLosses(){
return losses;
}
// TODO: Define getWinPercentage()
public double getWinPercentage(){
return ((double)wins)/(wins + losses);
}
// TODO: Define printStanding()
public void printStanding(){
double winPercentage;
winPercentage = getWinPercentage();
System.out.printf("Win percentage: %.2f\n", winPercentage);
if (winPercentage>= 0.5) {
System.out.println("Congratulations, Team " + name + " has a winning average!");
}
else{
System.out.println("Team " + name + " has a losing average.");
}
}
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

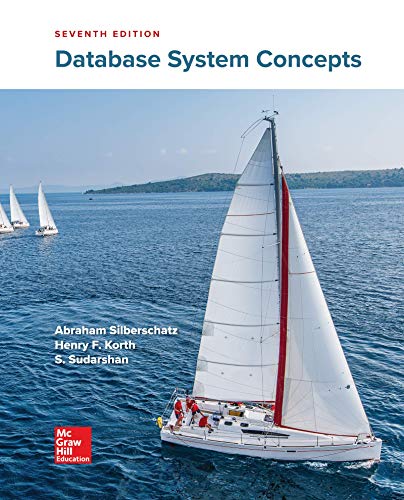
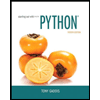
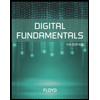
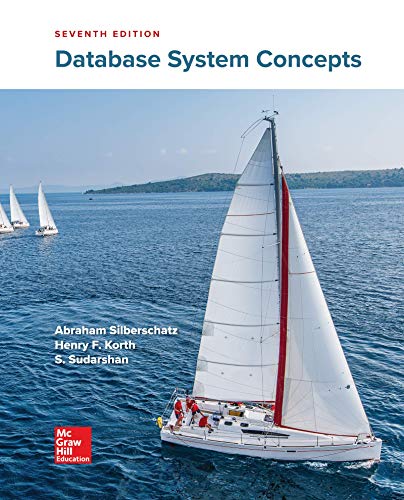
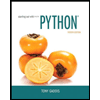
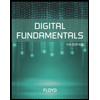
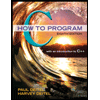
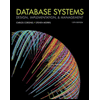
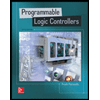