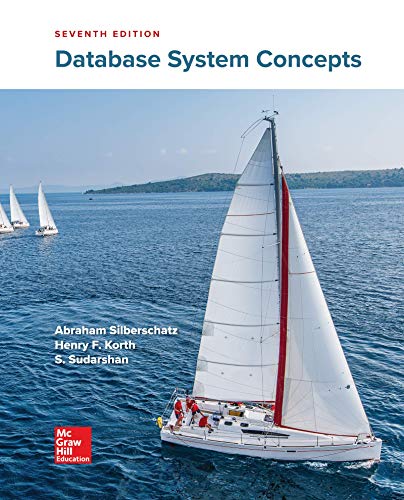
Concept explainers
import java.util.Scanner;
public class ThreeTestScore {
int size = 10;
//create arrays for 10 student information
String[] fname = new String[size];
String[] mname = new String[size];
String[] lname = new String[size];
int[] score1 = new int[size];
int[] score2 = new int[size];
int[] score3 = new int[size];
//read student informtion
public void readStudentInfo(Scanner sc) {
for (int i = 0; i < size; i++) {
System.out.print("Enter First Name: ");
String fname = sc.next();
System.out.print("Enter Middle Initial: ");
String mname = sc.next();
System.out.print("Enter Last Name: ");
String lname = sc.next();
int score1 = -1, score2 = -1, score3 = -1;
do {
System.out.print("Enter Score 1(between 0 and 100): ");
score1 = sc.nextInt();
} while (score1 < 0 || score1 > 100);
do {
System.out.print("Enter Score 2(between 0 and 100): ");
score2 = sc.nextInt();
} while (score2 < 0 || score2 > 100);
do {
System.out.print("Enter Score 3(between 0 and 100): ");
score3 = sc.nextInt();
} while (score3 < 0 || score3 > 100);
this.fname[i] = fname;
this.mname[i] = mname;
this.lname[i] = lname;
this.score1[i] = score1;
this.score2[i] = score2;
this.score3[i] = score3;
System.out.println();
}
}
public double getAverage(double score1, double score2, double score3) {
return (score1 + score2 + score3) / 3;
}
public double getClassAverage(double value) {
return value / size;
}
public void displayInfo() {
System.out.println("========================================================================");
System.out.println("First Name\tMI\tLast Name\tTest 1\tTest 2\tTest 3\t Average");
System.out.println("----------\t----\t---------\t-----\t-----\t------\t--------\t");
double class_avg = 0;
for (int i = 0; i < size; i++) {
class_avg += getAverage(score1[i], score2[i], score3[i]);
System.out.printf("%-10s\t%-2s\t%-10s\t%d\t%d\t%d\t%.2f\n", fname[i], mname[i], lname[i], score1[i], score2[i], score3[i], getAverage(score1[i], score2[i], score3[i]));
}
System.out.println("");
System.out.printf("class average: %.2f\n\n", getClassAverage(class_avg));
}
public static void main(String[] args) {
String option = "Yes";
Scanner sc = new Scanner(System.in);
do {
ThreeTestScore test = new ThreeTestScore();
test.readStudentInfo(sc);
test.displayInfo();
System.out.print("Do you want to restart the program?(Y/N): ");
option = sc.next().toLowerCase();
} while (!option.equals("n"));
}
}
in Arrays and method

Step by stepSolved in 3 steps with 2 images

- In java, the Arrays class contains a static binarySearch() method that returns …… if element is found. false true (-k–1) where k is the position before which the element should be inserted The index of the elementarrow_forwardconvert this code to JAVA location = [] size = [] rover = 0 def displayInitialList(location, size): global rover print("FSB# location Size") for i in range(len(location)): print(i," ",location[i]," ",size[i]) if rover<len(size)-1: print("Rover is at ",location[rover+1]) else: print("Rover is at ",location[rover]) def allocateMemory(location,size,blockSize): global rover if rover<len(size): while size[rover]<blockSize: rover+=1 if i==len(size): return False location[rover] += blockSize size[rover] -= blockSize rover+=1 return True else: return False def deallocateMemory(location,size,delLocation,delSize): i=0 while delLocation>location[i]: i+=1 location[i]-=delSize size[i]+=delSize while True: print("1. Define Initital memory\n2. Display initial FSB list\n3. Allocate memory\n4. Deallocate memory\n5. Exit") print("Enter choice: ",end="") choice = int(input()) if…arrow_forward*JAVA* complete method Delete the largest valueremoveMax(); Delete the smallest valueremoveMin(); class BinarHeap<T> { int root; static int[] arr; static int size; public BinarHeap() { arr = new int[50]; size = 0; } public void insert(int val) { arr[++size] = val; bubbleUP(size); } public void bubbleUP(int i) { int parent = (i) / 2; while (i > 1 && arr[parent] > arr[i]) { int temp = arr[parent]; arr[parent] = arr[i]; arr[i] = temp; i = parent; } } public int retMin() { return arr[1]; } public void removeMin() { } public void removeMax() { } public void print() { for (int i = 0; i <= size; i++) { System.out.print( arr[i] + " "); } }} public class BinarH { public static void main(String[] args) { BinarHeap Heap1 = new BinarHeap();…arrow_forward
- For any element in keysList with a value greater than 50, print the corresponding value in itemsList, followed by a comma (no spaces). Ex: If the input is: 32 105 101 35 10 20 30 40 the output is: 20,30, 1 #include 2 3 int main(void) { const int SIZE_LIST = 4; int keysList[SIZE_LIST]; int itemsList[SIZE_LIST]; int i; 4 6 7 8 scanf("%d", &keysList[0]); scanf ("%d", &keysList[1]); scanf("%d", &keysList[2]); scanf("%d", &keysList[3]); 10 11 12 13 scanf ("%d", &itemsList[0]); scanf ("%d", &itemsList[1]); scanf("%d", &itemsList[2]); scanf ("%d", &itemsList[3]); 14 15 16 17 18 19 /* Your code goes here */ 20 21 printf("\n"); 22 23 return 0; 24 }arrow_forwardpublic int binarySearch(int[]array, int num) {int low = 0;//low rangeint high = array.length -1; //high range int mid; //mid rangewhile () //while low is less than or equal to high{mid = ; //set middle range to be (low + high) /2if () { //if the array in the middle range = input number//return mid range }elseif () { //if the array in the middle range > input number//set the high value to be the mid value minus 1 }else{//set low value to be mid value plus one } }//return -1 here because that would mean that the number is not found in the loop}arrow_forwardMethod Details getCountInRange public static int getCountInRange(int[] array, int lower, int upper) Returns the number of values in the range defined by lower (inclusive) and upper (inclusive) Parameters: array - integer array lower - lower limit upper - upper limit Returns: Number of values found Throws: java.lang.IllegalArgumentException - if array is null or lower is greater than upper Any error message is fine (e.g., "Invalid parameters(s)")arrow_forward
- public class arrayOutput ( public static void main (String [] args) { final int NUM ELEMENTS = 3; int[] userVals = new int [NUM_ELEMENTS]; int i; } Type the program's output userVals [0] = 2; userVals [1] = 6; userVals [2] = 8; for (i = userVals.length - 1; i >= 0; −−1) { System.out.println(userVals [1]); } C.C. ? ? ??arrow_forwardpackage lab06;;public class gradereport { public static void main(String[] args) { Scanner in = new Scanner(System.in);double[] Scores = new double[10]; for(int i=0;i<10;i++){ System.out.println("Enter score " + (i+1));scores[i]=in.nextdouble(); } for(int i=0;i<10;i++){ if (scores[i] >=80) System.out.println("Score " + (i+1) + " receives a grade of HD"); else if (scores[i]>=70) System.out.println("Score " + (i+1) + " receives a grade of D"); else if (scores[i] >=60) System.out.println("Score "+ (i+1) + " receives a grade of C"); else if (scores[i] >=50) System.out.println("Score " + (i+1) + " receives a grade of P"); else if (scores[i] >=40) System.out.println("Score " + (i+1) + " receives a grade of MF"); else if (scores[i] >=0) System.out.println("Score " +…arrow_forwardfor the class ArrayAverage write a code that calculates the average of the listed numbers with a double result for the class ArrayAverageTester write a for each loop and print out the resultarrow_forward
- class Container {private int val1=1;private void printValue(){final int val2=2;class Containee{public void f(){System.out.println(String.format("Values are: %d and %d."),val1);}}}public static void main(String[] args) {(new Container()).printValue();}} a.The above does not compile because the method f() requires an instance of class Container b.The above does not compile because the class definition in done within the body of the function c.The above code complies with no errors, but does not produce any ouput d.The above code does not compile because the methodf() cannot access the variable val1arrow_forwardJavaTimer.java: import java.util.Arrays;import java.util.Random; public class JavaTimer { // Please expand method main() to meet the requirements.// You have the following sorting methods available:// insertionSort(int[] a);// selectionSort(int[] a);// mergeSort(int[] a);// quickSort(int[] a);// The array will be in sorted order after the routines are called!// Be sure to re-randomize the array after each sort.public static void main(String[] args) {// Create and initialize arraysint[] a = {1, 3, 5}, b, c, d;// Check the time to sort array along startTime = System.nanoTime();quickSort(a);long endTime = System.nanoTime();long duration = (endTime - startTime) / 1000l;// Output resultsSystem.out.println("Working on an array of length " + a.length + ".");System.out.println("Quick sort: " + duration + "us.");}// Thanks to https://www.javatpoint.com/insertion-sort-in-javapublic static void insertionSort(int array[]) {int n = array.length;for (int j = 1; j < n; j++) {int key = array[j];int…arrow_forwardimport java.awt.*; public class TestRectangle {public static void main(String[] args) {Rectangle r1 = new Rectangle(5, 4, 10, 17);Rectangle r2 = new Rectangle(10, 10, 20, 3);Rectangle r3 = new Rectangle(0, 1, 12, 15);Rectangle r4 = new Rectangle(10, 10, 20, 3);System.out.println("r1 = " + r1);System.out.println("r2 = " + r2);System.out.println("r3 = " + r3);System.out.println("r2 equals r1? " + r2.equals(r1));System.out.println("r2 equals r2? " + r2.equals(r2));System.out.println("r2 equals r3? " + r2.equals(r3));System.out.println("r2 equals r4? " + r2.equals(r4)); System.out.println("r1 contains (6, 8)? = " + r1.contains(6, 8));System.out.println("r2 contains (6, 8)? = " + r2.contains(6, 8));System.out.println("r3 contains (6, 8)? = " + r3.contains(6, 8));System.out.println("r2 contains (30, 13)? = " + r2.contains(30, 13)); r1.intersect(r3);r2.intersect(r4);System.out.println("r1 intersect r3 = " + r1);System.out.println("r2 intersect r4 = " + r2);…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
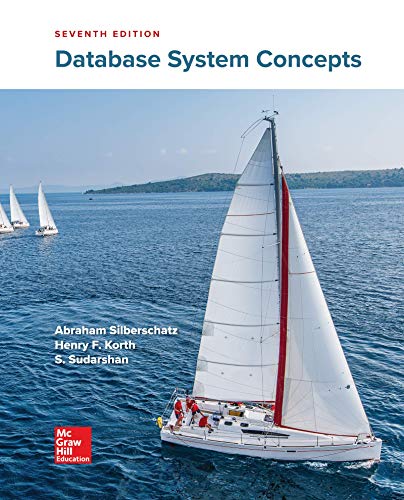
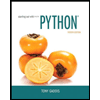
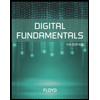
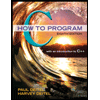
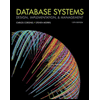
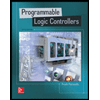