First, you will debug the Paint1 class so that no errors remain and all calculations work correctly by completing the following: o Find and fix the three errors in the given code. • Use the following sample input values to test your program. If you have found and fixed all the errors, your output should exactly match the sample. Input: 30 25 Output: Wall area: 750.0 square feet Paint needed: 2.142857142857143 gallons Tip: When you run your program in Eclipse, it will prompt you to enter input in the Console window. If you cannot find this window, go to Window, then Show View, then Console. Next, you will add loops to validate all user input and handle exceptions so that code passes all test cases by completing the following: • Review the code, looking for the two code blocks where user input is required. • Implement a do-while loop in both blocks of code to ensure that input is valid and any exceptions are handled. • Use the two following sample sets of input to test your program. Since these inputs are invalid, your program should respond to each by prompting the user to enter valid input. This should continue to loop until valid input is received. Input 1: Input 2: thirty 30 25 0
First, you will debug the Paint1 class so that no errors remain and all calculations work correctly by completing the following: o Find and fix the three errors in the given code. • Use the following sample input values to test your program. If you have found and fixed all the errors, your output should exactly match the sample. Input: 30 25 Output: Wall area: 750.0 square feet Paint needed: 2.142857142857143 gallons Tip: When you run your program in Eclipse, it will prompt you to enter input in the Console window. If you cannot find this window, go to Window, then Show View, then Console. Next, you will add loops to validate all user input and handle exceptions so that code passes all test cases by completing the following: • Review the code, looking for the two code blocks where user input is required. • Implement a do-while loop in both blocks of code to ensure that input is valid and any exceptions are handled. • Use the two following sample sets of input to test your program. Since these inputs are invalid, your program should respond to each by prompting the user to enter valid input. This should continue to loop until valid input is received. Input 1: Input 2: thirty 30 25 0
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter5: Control Structures Ii (repetition)
Section: Chapter Questions
Problem 19PE
Related questions
Question

Transcribed Image Text:First, you will debug the Paint1 class so that no errors remain and all calculations work correctly by completing the following:
o Find and fix the three errors in the given code.
• Use the following sample input values to test your program. If you have found and fixed all the errors, your output should exactly match
the sample.
Input:
30
25
Output:
Wall area: 750.0 square feet
Paint needed: 2.142857142857143 gallons
Tip: When you run your program in Eclipse, it will prompt you to enter input in the Console window. If you cannot find this window, go to
Window, then Show View, then Console.
Next, you will add loops to validate all user input and handle exceptions so that code passes all test cases by completing the following:
• Review the code, looking for the two code blocks where user input is required.
• Implement a do-while loop in both blocks of code to ensure that input is valid and any exceptions are handled.
• Use the two following sample sets of input to test your program. Since these inputs are invalid, your program should respond to each by
prompting the user to enter valid input. This should continue to loop until valid input is received.
Input 1: Input 2:
thirty 30
25
0
![import java.util.Scanner;
public class Paintl {
+
public static void main(String[] args) {
Scanner scnr = new Scanner (System.in);
double wallHeight = 0.0;
}
double wallWidth = 0.0;
double wallArea = 0.0;
double gallons PaintNeeded = 0.0;
final double square Feet PerGallons = 350.0;
// Implement a do-while loop to ensure input is valid
// Prompt user to input wall's height
System.out.println("Enter wall height (feet): ");
wallHeight = scnr.nextDouble();
// Calculate and output the amount of paint (in gallons)
needed to paint the wall
// Implement a do-while loop to ensure input is valid
// Prompt user to input wall's width
System.out.println("Enter wall width (feet): ");
wallHeight = scnr.nextDouble();
}
// Calculate and output wall area
wallArea = wallHeight * wallWidth;
System.out.println("Wall area: square feet");
gallons PaintNeeded = wallArea/square Feet PerGallons;
System.out.println("Paint needed: " + gallonspaintneeded
gallons");](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fc697cfa7-35be-400d-88d6-26bf90a0b862%2F4480a57c-221c-40fd-ab99-8c924fc92efb%2Fslebq6i_processed.png&w=3840&q=75)
Transcribed Image Text:import java.util.Scanner;
public class Paintl {
+
public static void main(String[] args) {
Scanner scnr = new Scanner (System.in);
double wallHeight = 0.0;
}
double wallWidth = 0.0;
double wallArea = 0.0;
double gallons PaintNeeded = 0.0;
final double square Feet PerGallons = 350.0;
// Implement a do-while loop to ensure input is valid
// Prompt user to input wall's height
System.out.println("Enter wall height (feet): ");
wallHeight = scnr.nextDouble();
// Calculate and output the amount of paint (in gallons)
needed to paint the wall
// Implement a do-while loop to ensure input is valid
// Prompt user to input wall's width
System.out.println("Enter wall width (feet): ");
wallHeight = scnr.nextDouble();
}
// Calculate and output wall area
wallArea = wallHeight * wallWidth;
System.out.println("Wall area: square feet");
gallons PaintNeeded = wallArea/square Feet PerGallons;
System.out.println("Paint needed: " + gallonspaintneeded
gallons");
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
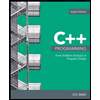
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
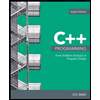
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning