import java.io.*; import java.util.stream.*; public class Solution { static class ListCell { public T datum; // Data for this cell public ListCell next; // Next cell public ListCell(T datum, ListCell next) { this.datum = datum; this.next = next; } } static class LinkedList { private static final String STRING = " "; Solution.ListCell head; // head (first cell) of the List public LinkedList() { head = null; } public void insert(T element) { head = new ListCell(element, head); } public void delete(T element) { delete(element, head); } private ListCell delete(T element, ListCell cell) { if (cell == null) return null; if (cell.datum.equals(element)) return cell.next; cell.next = delete(element, cell.next); return cell; } public int size() { return size(head); } private int size(ListCell cell) { if (cell == null) return 0; return size(cell.next) + 1; } public String toString() { return toString(head); } private String toString(ListCell cell) { if (cell == null) return ""; return cell.datum.toString() + STRING + toString(cell.next); } } // Complete the mergeSort function below. // !!! Leave the code as is except for the below function, !!! // !!! though writing helper function(s) are allowed. !!! private static void sort(Solution.LinkedList llist) { } public static void main(String[] args) throws IOException { BufferedWriter bufferedWriter = new BufferedWriter(new OutputStreamWriter(System.out)); BufferedReader br = new BufferedReader(new InputStreamReader(System.in)); int llistCount = Integer.parseInt(br.readLine().trim()); LinkedList llist = new LinkedList<>(); IntStream.range(0, llistCount).forEach(i -> { try { Integer llistItem = Integer.parseInt(br.readLine().trim()); llist.insert(llistItem); } catch (IOException ex) { throw new RuntimeException(ex); } }); sort(llist); bufferedWriter.write(llist.toString().trim()); bufferedWriter.close(); br.close(); } }
import java.io.*;
import java.util.stream.*;
public class Solution {
static class ListCell<T> {
public T datum; // Data for this cell
public ListCell<T> next; // Next cell
public ListCell(T datum, ListCell<T> next) {
this.datum = datum;
this.next = next;
}
}
static class LinkedList<T> {
private static final String STRING = " ";
Solution.ListCell<T> head; // head (first cell) of the List
public LinkedList() {
head = null;
}
public void insert(T element) {
head = new ListCell<T>(element, head);
}
public void delete(T element) {
delete(element, head);
}
private ListCell<T> delete(T element, ListCell<T> cell) {
if (cell == null)
return null;
if (cell.datum.equals(element))
return cell.next;
cell.next = delete(element, cell.next);
return cell;
}
public int size() {
return size(head);
}
private int size(ListCell<T> cell) {
if (cell == null)
return 0;
return size(cell.next) + 1;
}
public String toString() {
return toString(head);
}
private String toString(ListCell<T> cell) {
if (cell == null)
return "";
return cell.datum.toString() + STRING + toString(cell.next);
}
}
// Complete the mergeSort function below.
// !!! Leave the code as is except for the below function, !!!
// !!! though writing helper function(s) are allowed. !!!
private static void sort(Solution.LinkedList<Integer> llist) {
}
public static void main(String[] args) throws IOException {
BufferedWriter bufferedWriter = new BufferedWriter(new OutputStreamWriter(System.out));
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
int llistCount = Integer.parseInt(br.readLine().trim());
LinkedList<Integer> llist = new LinkedList<>();
IntStream.range(0, llistCount).forEach(i -> {
try {
Integer llistItem = Integer.parseInt(br.readLine().trim());
llist.insert(llistItem);
} catch (IOException ex) {
throw new RuntimeException(ex);
}
});
sort(llist);
bufferedWriter.write(llist.toString().trim());
bufferedWriter.close();
br.close();
}
}



Trending now
This is a popular solution!
Step by step
Solved in 2 steps

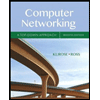
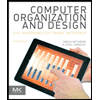
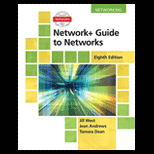
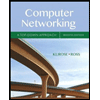
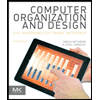
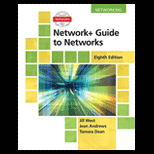
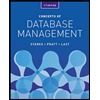
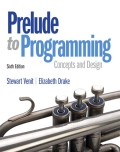
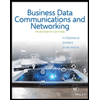