Queue example – make this generic, instead of a Queue of Customer. public class Queue { private ArrayList line; public Queue() { line = null; } public void enqueue (Customer c) { Customer newc = new Customer (c); // copy constructor plateful.add(newc); } public Customer dequeue (Customer c) { Customer newc = line.get(0); plateful.remove(0); return newc; } } // end of class definition
Queue example – make this generic, instead of a Queue of Customer. public class Queue { private ArrayList line; public Queue() { line = null; } public void enqueue (Customer c) { Customer newc = new Customer (c); // copy constructor plateful.add(newc); } public Customer dequeue (Customer c) { Customer newc = line.get(0); plateful.remove(0); return newc; } } // end of class definition
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Queue example – make this generic, instead of a Queue of Customer.
public class Queue
{
private ArrayList<Customer> line;
public Queue()
{
line = null;
}
public void enqueue (Customer c)
{
Customer newc = new Customer (c); // copy constructor
plateful.add(newc);
}
public Customer dequeue (Customer c)
{
Customer newc = line.get(0);
plateful.remove(0);
return newc;
}
} // end of class definition
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
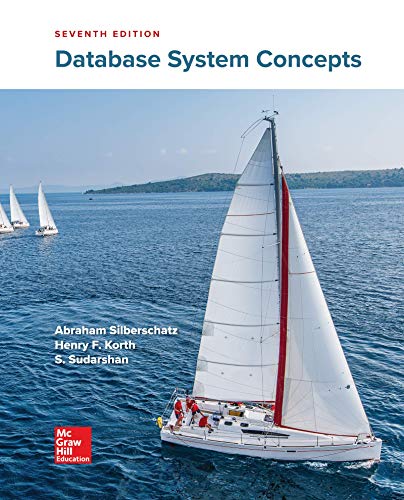
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
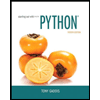
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
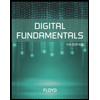
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
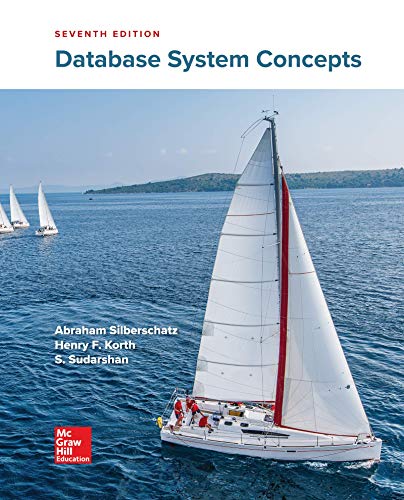
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
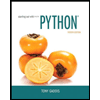
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
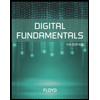
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
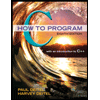
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
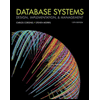
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
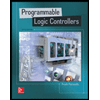
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education