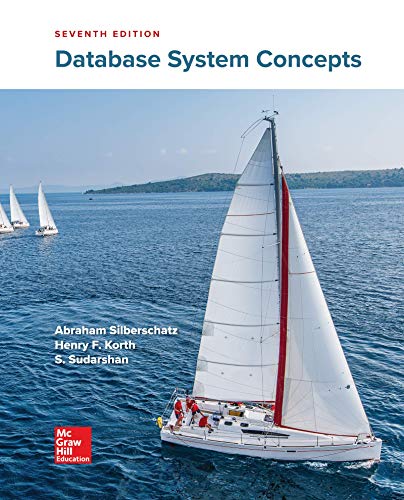
Concept explainers
I have to write an
#ifdef _WIN32
#include <Windows.h>
#else
#include <unistd.h>
#endif
#include <iostream>
#include <cstdlib>
using namespace std;
class GenrateShape {
public:
void SqaureShape(int length) {
for (int row = 1; row <= length; row++)
{
sleep (1);
for (int col = 1; col <= length; col++)
{
cout << "p"<< " ";
}
cout << endl;
}
}
void TriangleShape(int length) {
for (int i = 1; i <= length; i++)
{
sleep(1);
for (int j = length - i; j > 0; j--)
cout << " ";
for (int b = 1; b <= i; b++)
cout << "p" << " ";
cout << endl;
}
}
void pentagonShape(int length) {
TriangleShape(length);
SqaureShape(length);
}
};
int main()
{
int length = 13;
GenrateShape shape;
shape.pentagonShape(length);
return 0;
}
DESIGN (ALGORITHM):
On a piece of paper (or word processor), write down the algorithm, or sequence of steps, that you will
use to solve the problem. You may think of this as a “recipe” for someone else to follow. Continue to
refine your “recipe” until it is clear and deterministically solves the problem. Be sure to include the steps
for prompting for input, performing calculations, and displaying output.
You should attempt to solve the problem by hand first (using a calculator as needed) to work out what
the answer should be for a few sets of inputs.
Type these steps and calculations into a document (i.e., Word, text, or PDF) that will be submitted along
with your source code. Note that if you do any work by hand, images (such as pictures) may be used, but
they must be clear and easily readable. This document shall contain both the algorithm and any supporting
hand-calculations you used in verifying your results.

Introduction:
Algorithm is a sequential step of any program. In algorithm there is no specific language need. We can take simple language for explaining about step-by-step instructions. With algorithm we can easily understand the flow of program.
Step by stepSolved in 3 steps

- Java code 8. Given an existing ArrayList named friendList, find the first index of a friend named Sasha and store it in a new variable named index. 9. Given that an ArrayList of Integers named numberList has already been created and several values have already been inserted, write the statement necessary to insert the value 25 at the end of the list. 10. Declare an integer array named evens and fill it with even numbers from 2 through 10 in one statement. 11. Given an existing array named pets, find the size of the array and store it in a new variable named numPets. 12. Given an existing ArrayList named contactList, find the number of contacts in the ArrayList and store it in the existing variable named numContacts.arrow_forwardC++ ONLY PLEASE, and please read carefully, i have had people submit wrong answers Assignment 7 A: Rare Collection. We can make arrays of custom objects just like we’ve done with ints and strings. While it’s possible to make both 1D and 2D arrays of objects (and more), for this assignment we’ll start you out with just one dimensional arrays. Your parents have asked you to develop a program to help them organize the collection of rare CDs they currently have sitting in their car’s glove box. To do this, you will first create an AudioCD class. It should have the following private attributes. String cdTitle String[4] artists int releaseYear String genre float condition Your class should also have the following methods: Default Constructor: Initializes the five attributes to the following default values: ◦ cdTitle = “” ◦ artists = {“”, “”, “”, “”} ◦ releaseYear = 1980 ◦ genre = “” ◦ condition = 0.0 Overloaded Constructor: Initializes the five attributes based on values passed…arrow_forwardWhy my code given me error’s # Python code to draw snowflakes fractal. import turtle import random # setup the window with a background color wn = turtle.Screen() wn.bgcolor("cyan") # assign a name to your turtle elsa = turtle.Turtle() elsa.speed(15) # create a list of colours scolor = ["white", "blue", "purple", "grey", "magenta"] # create a function to create different size snowflakes def snowflake(size): # move the pen into starting position elsa.penup() elsa.forward(10*size) elsa.left(45) elsa.pendown() elsa.color(random.choice(sfcolor)) # draw branch 8 times to make a snowflake for i in range(8): branch(size) elsa.left(45) # create one branch of the snowflake def branch(size): for i in range(3): for i in range (3): elsa.forward(10.0*size/3) # draw branch 8 times to make a snowflake for i in range(8): branch(slice) elsa.left(45) # create one branch of the snowflake def branch (size): for…arrow_forward
- 个 O codestepbystep.com/problem/view/java/loops/ComputeSumOfDigits?problemsetid=4296 You are working on problem set: HW2- loops (Pause) ComputeSumOfDigits ♡ Language/Type: % Related Links: Java interactive programs cumulative algorithms fencepost while Scanner Write a console program in a class named ComputeSumOfDigits that prompts the user to type an integer and computes the sum of the digits of that integer. You may assume that the user types a non-negative integer. Match the following output format: Type an integer: 827184 Digit sum is 22 JE J @ C % 123 A 2 7 8 9 Class: Write a complete Java class. Need help? Stuck on an exercise? Contact your TA or instructor If something seems wrong with our site, please contact us. Submit t US Oct 13 9:00 Aarrow_forwardTime remaining: 00:09:10 Computer Science this file is named HW3.Java can someone run it through a GNU compiler and please show me the outputs import java.io.FileWriter;import java.io.IOException;public class HW3 {public static void main(String[] args) throws IOException {// 0th argument contains the name of algorithmString algo = args[0];// 1st argument contains the name of file// Make a new fileFileWriter fw = new FileWriter(args[1]);if (algo.equals("p1")) {// 2nd argument comes in the form of string.// convert it to intger and run a loopfor (int i = 1; i <= Integer.parseInt(args[2]); i++) {double[] x = new double[(int) Math.pow(10, i)];// use start and end for recording timelong start = System.currentTimeMillis();prefixAverage1(x);long end = System.currentTimeMillis();double totalTime = Math.log10(end - start);fw.write(String.valueOf(totalTime));String newLine = System.getProperty("line.separator");fw.write(newLine);}} else if (algo.equals("p2")) {for (int i = 1; i <=…arrow_forwardReferences Mailings Review View Help Picture Format EE AL T AaBbCcD AaBbCcDc AaBbC AaBbCcE AaB Find TI Normal TNo Spac. Heading 1 Heading 2 Replas Title A Select Paragraph Styles Editing Instructions BadsubscriptCaughtj. + 1 import java.util.*; 2 public class BadSubscriptcaught Write an application in which you 3 { declare an array of eight first 4 public static void main(String[] args) names. Write a try block in { which you prompt the user for an String[) names - {"Ariel", "Brad", "Clifford", "Denise", "Emily", integer and display the name in the requested position. Create a "Fred", "Gina", "Henry"}; Scanner keyhoard new Scanner(System.in); int number; catch block that catches the potential 10 ArrayIndexOutOfBoundsExceptio W your code here thrown when the user enters a number that is out of range. The catch block also should display the error message Subscript out of range. NFocus 144 & 6. 8. R T. Y F G J K LLarrow_forward
- BAGEL FILES import javax.swing.JFrame; public class Bagel{//-----------------------------------------------------------------// Creates and displays the controls for a bagel shop.//-----------------------------------------------------------------public static void main (String[] args){JFrame frame = new JFrame ("Bagel Shop");frame.setDefaultCloseOperation (JFrame.EXIT_ON_CLOSE); frame.getContentPane().add(new BagelControls()); frame.pack();frame.setVisible(true);}} import java.awt.*;import java.awt.event.*; import javax.swing.*; public class BagelControls extends JPanel{private JComboBox bagelCombo;private JButton calcButton;private JLabel cost;private double bagelCost;public BagelControls(){String[] types = {"Make A Selection...", "Plain","Asiago Cheese", "Cranberry"};bagelCombo = new JComboBox(types);calcButton = new JButton("Calc");cost = new JLabel("Cost = " + bagelCost);setPreferredSize (new Dimension (400,…arrow_forwardusing System;using System.Text.RegularExpressions;class chapter8 {static void Main() {string words = "08/14/57 46 02/25/59 45 06/05/85 18" +"03/12/88 16 09/09/90 13";string regExp1 = "(\\s\\d{2}\\s)";MatchCollection matchSet = Regex.Matches(words,regExp1);foreach (Match aMatch in matchSet)Console.WriteLine(aMatch.Groups[0].Captures[0]);}} Now let’s modify this program to search for dates instead of ages, and usea grouping construct to organize the dates. And write the c# code:arrow_forwardfor the class ArrayAverage write a code that calculates the average of the listed numbers with a double result for the class ArrayAverageTester write a for each loop and print out the resultarrow_forward
- /*** creates an array of 1000 integers where each element matches its index* * @return the array that is created*/public static int[] makeNumberArray() {arrow_forwardpython: numpy def coupons(my_list, on_sale, prices): ''' QUESTION 5 It's the time of week to go grocery shopping again! Given three arrays, one of what's on sale, one of your own list, and one of the prices, return an array of the items on your list that are on sale and more than five dollars. You have MORE coupons than you have groceries on your list- make sure to only use the coupons that correspond to your list. THIS MUST BE DONE IN ONE LINE Hint: use masking and slicing Args: my_list(np.array) on_sale(np.array) prices(np.array) Return: np.array >>> my_list = np.array(["eggs", "2% milk", "green onions", "whole wheat bread", "onions", "spinach", "peanut butter", "boxed spaghetti", "salt and vinegar chips", "alfredo sauce"]) >>> on_sale = np.array([True, False, True, True, True, True, False, False, False, True, False, True, False])…arrow_forwardSuppose we have an array as int array[] = {1.2,8.7,6.7,9.6,5.4,4.2,3};. Provide a code that reverses the elements in the array such that the first one becomes the last one and so on. Hint: You could make use of a temporary variable. c++ languagearrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
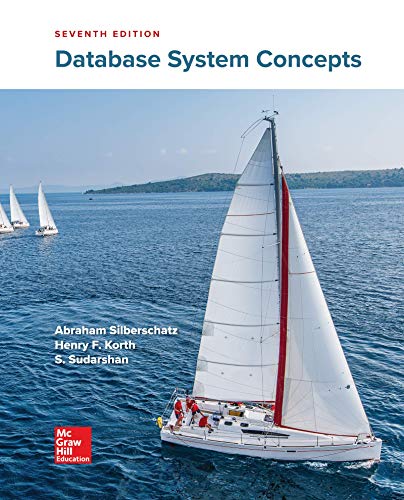
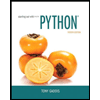
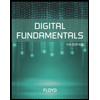
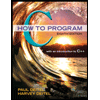
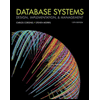
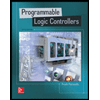