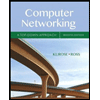
PYTHON:
Can you please help me to modify this code in a way that it prints out the same output as the image below.
class Node:
def __init__(self, key):
self.left = None
self.right = None
self.val = key
# this is the function for Inorder
def printInorder(root):
if root:
# here calling the left child
printInorder(root.left)
# here printing the node data
print(root.val, end=" "),
# here calling the right child
printInorder(root.right)
# this is the Preorder method
def printPreorder(root):
if root:
# here printing the node data
print(root.val, end=" "),
# here calling the left child
printPreorder(root.left)
# here calling the right child
printPreorder(root.right)
# thsi is the postorder method
def printPostorder(root):
if root:
# here calling the left child
printPostorder(root.left)
# here calling the right child
printPostorder(root.right)
# here printing the node data
print(root.val, end=" "),
# this is the main function
if __name__ == "__main__":
root = Node('G')
root.left = Node('D')
root.right = Node('B')
root.left.left = Node('E')
root.left.right = Node('A')
root.right.left = Node('C')
root.right.right = Node('F')
# here calling the inorder method
print("Inorder:")
printInorder(root)
print('')
# here calling the Preorder method
print("Preorder:")
printPreorder(root)
print('')
# here calling the Postorder method
print("Postorder:")
printPostorder(root)
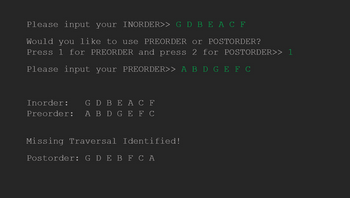

Step by stepSolved in 2 steps

- %matplotlib inlineimport mathimport numpy as npfrom matplotlib import pyplot as pltdef bad_poisson(lmd, k): return pow(lmd,k)*math.exp(-lmd)/math.factorial(k)# bad_poisson(1000,1000) # uncomment to see it breaksarrow_forwardvWhat happens when an object such as an array is no longer referenced by a variable?arrow_forwardHelparrow_forward
- Data structure & Algrothium java program Create the three following classes: 1. class containing two strings attributes first name and last name.2. class containing the name object and an ArrayList of string to store the list gifts. This class would extend the attached NicePersonInterface.java3. class containing one ArrayList of Names to store the naughty names. Another ArrayList of NicePerson to store the nice names and gifts. Add atleast 4 names in each list and display all information.arrow_forwardin java Integer numVals is read from input and integer array userCounts is declared with size numVals. Then, numVals integers are read from input and stored into userCounts. If the first element is less than the last element, then assign Boolean firstSmaller with true. Otherwise, assign firstSmaller with false. Ex: If the input is: 5 40 22 41 84 77 then the output is: First element is less than last element 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 import java.util.Scanner; public class UserTracker { publicstaticvoidmain(String[] args) { Scannerscnr=newScanner(System.in); intnumVals; int[] userCounts; inti; booleanfirstSmaller; numVals=scnr.nextInt(); userCounts=newint[numVals]; for (i=0; i<userCounts.length; ++i) { userCounts[i] =scnr.nextInt(); } /* Your code goes here */ if (firstSmaller) { System.out.println("First element is less than last element"); } else { System.out.println("First element is not less…arrow_forwardCreate a class called CustomerListerwith a mainmethod that instantiates an array of Stringobjects called customerName. The array should have room for five Stringobjects. Use an initializer list to put the following names into the array:Cathy Ben Jorge Wanda FreddieUse newto create a second doublearray called customerBalancein the mainmethod. Allow room for five customer balances, each stored as a double.In the loop that prints each customer name, add some code to prompt the user to enter a balance for that customer. Read the keyboard input with a Scannerobject. Use the following balances for the input:100.00234.562.4932.32400.00After all the balances have been entered, print out each customer and his/her balance.arrow_forward
- /** * TODO: file header */ import java.util.*; public class BSTManual { /** * TODO * @author TODO * @since TODO */ // No style for this file. public static ArrayList<String> insertElements() { ArrayList<String> answer_pr1 = new ArrayList<>(11); /* * make sure you add your answers in the following format: * * answer_pr1.add("1"); --> level 1 (root level) of the output BST * answer_pr1.add("2, X"); --> level 2 of the output BST * answer_pr1.add("3, X, X, X"); --> level 3 of the output BST * * the above example is the same as the second pictoral example on your * worksheet */ //TODO: add strings that represent the BST after insertion. return answer_pr1; } public static ArrayList<String> deleteElements() { ArrayList<String> answer_pr2 = new ArrayList<>(11); /* * Please refer to the example in insertElements() if you lose track * of how to properly enter your answers */ //TODO: add strings that represent the…arrow_forwardfor the class ArrayAverage write a code that calculates the average of the listed numbers with a double result for the class ArrayAverageTester write a for each loop and print out the resultarrow_forwardPYTHON!! class Triangle: def __init__(self,sides): self.a = sides[0] self.b = sides[1] self.c = sides[2] if __name__ == '__main__': t = Triangle([3,6,2]) print(f'Side1 = {t.a}') print(f'Side2 = {t.b}') print(f'Side3 = {t.c}') Based on the code. Assume you are in the Triangle class: Create a method called calcArea. It will calculate and return the area of the triangle using this formula:arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
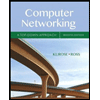
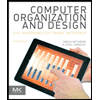
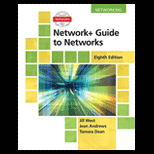
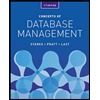
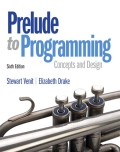
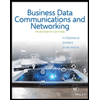