class ArrayStackException extends Exception { ArrayStackException(String m) { super(m); } } public class ArrayStack { private int s[] = new int[100]; private int top=0; public void push(int data) throws ArrayStackException { if (top>=s.length) throw new ArrayStackException("Push overflow: " + data); s[top]=data; top++; } public int pop() throws ArrayStackException { if (top<=0) throw new ArrayStackException("Pop underflow: "); int value = s[top-1]; top--; return value; } public int peek() { int value = s[top-1]; return value; } public void clear() { top=0; } public int size() { return top; } public void display() { System.out.print( "size =" +top + ": "); for(int i =0; i
class ArrayStackException extends Exception {
ArrayStackException(String m) {
super(m);
}
}
public class ArrayStack {
private int s[] = new int[100];
private int top=0;
public void push(int data) throws ArrayStackException {
if (top>=s.length) throw new ArrayStackException("Push overflow: " + data);
s[top]=data;
top++;
}
public int pop() throws ArrayStackException {
if (top<=0) throw new ArrayStackException("Pop underflow: ");
int value = s[top-1];
top--;
return value;
}
public int peek() {
int value = s[top-1];
return value;
}
public void clear() {
top=0;
}
public int size() {
return top;
}
public void display() {
System.out.print( "size =" +top + ": ");
for(int i =0; i<top;i++) {
System.out.print( s[i] + " ");
}
System.out.println( );
}
public static void main(String[] s) {
ArrayStack mystack = new ArrayStack();
try {
mystack.push(30);
mystack.push(20);
mystack.push(10);
mystack.display();
mystack.pop();
mystack.display();
mystack.pop();
mystack.pop();
mystack.pop();
mystack.pop();
} catch (ArrayStackException e) {
System.out.println("Exception "+e.getMessage());
}
}
}
Modify the code to store a character instead of a String. Name it CharStack.
Use CharStack to implement a nesting bracket validator.
Examples:
( x+y / { a } ) + [ X ] // valid
{ f + ( c + g } ) // not valid
Name the program Nest.java create a method:
int nested( String s )
The method will return the location of a nesting failure or -1 if the string s is
correctly nested.
Your program should check for the following pairs of nesting characters: {} [] ()
Hint: Parse the string one character at a time from beginning to end using a
loop.
When you encounter a opening character [{( push it on the stack.
When you encounter a closing character ]}) pop the stack and check to make sure that the closing
character matches the opening character. If they do not match return the character location.
If you process the entire string and the stack is empty then you have a valid matched expression
return -1.
If you ever throw a StackException then the expression is not balanced.
Your test driver should test your nested() method with the expressions

Step by step
Solved in 5 steps with 3 images

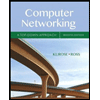
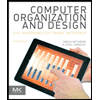
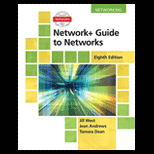
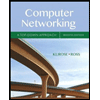
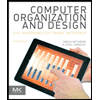
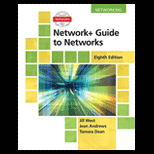
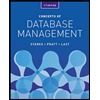
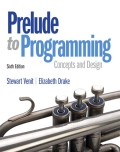
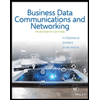