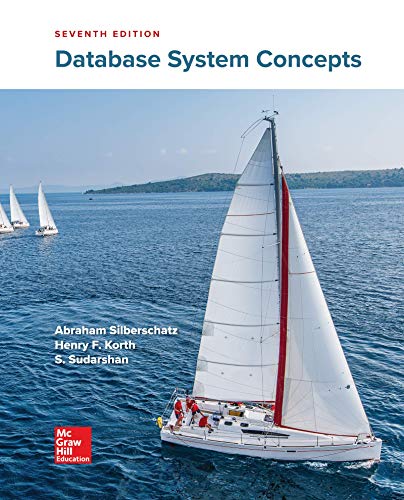
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
Please fill in the blanks. Programing language is C.
/*For simplicity, this program will get the information of each client,
and print it right back.
We could get the information of all clients first, then print them
all later for better readability using an array of struct.*/
#include<stdio.h>
#include<stdbool.h>
#define length 70 //assume there's no names longer than 70
/*It's good practice to add all function headers
to the top of the program*/
__1__ __2__ getEmployeeInfo();
__3__ printEmployeeInfo(__4__ __5__ em);
__6__ __7__ getClientInfo(__8__ i);
__9__ printClientInfo(__10__ __11__ cli, __12__ i);
/*Employee struct:
all the arrays use the same constant size defined on top
name-first, last-(string)
title (string)
number of clients (integer)
number of years working at the company(can take decimal points). */
__13__ employee
{
__14__ first_name[__15__];
__16__ last_name[__17__];
__18__ title[__19__];
__20__ num_clients;
__21__ num_yrs_worked;//3 and a half year would be 3.5
};
/*Client struct:
name (first, last)
client ID (integer)
age (integer)
number of products purchased (integer).*/
__22__ client
{
__23__ first_name[__24__];
__25__ last_name[__26__];
__27__ clientID;
__28__ age;
};
/*EMPLOYEE
This function get an employee's info,
save it to the employee struct, and
return the employee struct*/
__29__ __30__ getEmployeeInfo()
{
struct employee emp;
printf("\nNeed 2 values. Enter the employee full name (first, then last): ");
scanf(" %s %__31__",__32__, emp.last_name);
printf("Enter the employee's title: ");
scanf(" __33__",__34__);
printf("Need 2 numbers. Enter the number of clients, then years of employment:");
scanf("%__35__ %__36__", __37__, __38__);
return __39__;
}
/*This function takes an employee struct and print their info
Ex: Hello first_name last_name. Title: def. Number of clients: abc. #years of employment: xyz.*/
__40__ printEmployeeInfo(__41__ __42__ em)
{
printf("xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx\n");
printf("Printing back Employee Info\n");
printf("\nHello %s %s.\nTitle: %s.\n", em.first_name, em.last_name, em.title);
printf("Number of clients:__43__.\n", __44__);
printf("Number years of employment: %.1f.\n", em.num_yrs_worked);
printf("xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx");
}
/*CLIENT*/
/*This function take an index, get a client's info and return the client struct*/
__45__ __46__ getClientInfo(int i)
{
printf("\nProcessing Client #%d", i+1);
struct client cli;
printf("\nNeed 2 values. Enter the client full name (first, then last): ");
scanf(" __47__ __48__",__49__, __50__);
printf("Need 2 numbers. Enter your client ID, followed by your age: ");
scanf("__51__ __52__",__53__, __54__);
return __55__;
}
/*This function takes an client struct and print their info:
Ex: Hello first_name last_name. Client ID: abc. Age: xyz. */
__56__ printClientInfo(struct client cli, int i)
{
printf("\n------------------------\n");
printf("Printing Client #%d info: ", i+1);
printf("\nHello %__57__ %__58__.\n", __59__, cli.last_name);
printf("ClientID: %__60__.\n", __61__);
printf("Age: %__62__.", __63__);
printf("\n------------------------\n");
}
//MAIN
int main()
{
//Create 2 struct for employee, and client in that order
struct employee my_emp;
struct client my_cli;
printf("Hi, I'm here to take some statistics: ");
/*Get and print EMPLOYEE's info*/
__64__ = getEmployeeInfo();
printEmployeeInfo(__65__);
/*Get and print CLIENTs's info
Use \ to break printf to multiple lines without getting errors*/
printf("\n\nNow, let's move on to your client(s). \nAccording to the chart,\
you have %d clients.\n", my_emp.num_clients);
//Going through all the clients, get&print each client's info
for(int i = 0; i < __66__; i ++)
{
__67__ = getClientInfo(i);
printClientInfo(__68__, i);
}
printf("\n\nThank you for your patience. You have a great day :)\n");
return 0;
}
![Task 3: Struct
You will write a program to keep track of a company's employee and his/her clients. We'll have:
1. TWO structures: the employee and the client.
2. 2 functions for each struct (different structure >>> different number of parameters) to:
Get the information (initialization)
Print back the information
We'll assume there will be only 1 employee. # of clients depends on the user's inputs.
Here's the information needed for each struct:
Employee struct: first name, last name, title, number of clients, and number of years
working at the company (take decimal points).
Client struct: first name, last name, age, client ID, and the number of products purchased.
In main, we'll:
• declare each struct
for each struct, call their corresponding function to get the information, and print it back.
Hint:
Depends on how many clients, you'll need to use loops to get information for all of them
(we'll use for loop in the quiz).
You can get number of clients from the employee.
#define length 50 will create a constant global variable length of size 50 to be used in any
function. Put this line on top of your program.
o For example, char lastName[length] uses length global variable.
• For scanf and printf and the character array, you can use %s instead of %c to get the full
name instead of just the initial ("Bob" vs 'B'). We'll talk about it more next week.
o
For example: char first_name[length]; scanf("%s", first_name) for "Bob".
o Notice there is no & next to first_name. Only for %s when scanning.
•
•](https://content.bartleby.com/qna-images/question/13b8e596-45c0-434d-ab2b-ea5ebc953e71/1a100428-8f98-4e66-ab5f-56d6a2313b03/uk2yjeu_thumbnail.jpeg)
Transcribed Image Text:Task 3: Struct
You will write a program to keep track of a company's employee and his/her clients. We'll have:
1. TWO structures: the employee and the client.
2. 2 functions for each struct (different structure >>> different number of parameters) to:
Get the information (initialization)
Print back the information
We'll assume there will be only 1 employee. # of clients depends on the user's inputs.
Here's the information needed for each struct:
Employee struct: first name, last name, title, number of clients, and number of years
working at the company (take decimal points).
Client struct: first name, last name, age, client ID, and the number of products purchased.
In main, we'll:
• declare each struct
for each struct, call their corresponding function to get the information, and print it back.
Hint:
Depends on how many clients, you'll need to use loops to get information for all of them
(we'll use for loop in the quiz).
You can get number of clients from the employee.
#define length 50 will create a constant global variable length of size 50 to be used in any
function. Put this line on top of your program.
o For example, char lastName[length] uses length global variable.
• For scanf and printf and the character array, you can use %s instead of %c to get the full
name instead of just the initial ("Bob" vs 'B'). We'll talk about it more next week.
o
For example: char first_name[length]; scanf("%s", first_name) for "Bob".
o Notice there is no & next to first_name. Only for %s when scanning.
•
•
Expert Solution

arrow_forward
Step 1
The complete C program is given below:
Step by stepSolved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- This is loosely based on a program in Python for a sub-list sorting program. What are the coupling and cohesion levels in each function block in this structure chart, and explain what makes them that type of coupling and cohesion:.arrow_forwardWRITE A PROGRAM IN C++ You work for an loan analytics organization have been tasked with writing a program that simulates an analysis of loan applications. Code a modular program that uses parallel arrays to store loan application credit scores (values generated between 300 and 900), score ratings (poor, fair, good, very good, or exceptional based on credit score) and loan status (approved or declined applications based on credit score). The program must do the following: The program must first ask the user for the number of loan applications that will be in the simulation. This must be done by calling the getNumLoanApplications() function (definition below). After the input of the number of accounts, the program must use loops and random numbers to generate the credit score, score rating, and application result for each loan application in parallel arrays. These arrays must not be declared globally (major error). The following arrays must be declared and populated: creditScores[]…arrow_forwardIn C++, write a program that reads in an array of type int. You may assume that there are fewer than 20 entries in the array. The output must be a two-column list. The first column is a list of the distinct array elements and the second column is the count of the number of occurences of each element.arrow_forward
- python only define the following function: This function must reset the value of every task in a checklist to False. It accept just one parameter: the checklist object to reset, and it must return the (now modified) checklist object that it was given. Define resetChecklist with 1 parameter Use def to define resetChecklist with 1 parameter Use a return statement Within the definition of resetChecklist with 1 parameter, use return _in at least one place. Use any kind of loop Within the definition of resetChecklist with 1 parameter, use any kind of loop in at least one place.arrow_forwardWrite in C++ 1. Define a struct for a soccer player that stores their name, jersey number, and total points scored. 2.Using the struct in #1, write a function that takes an array of soccer players and its size as arguments and returns the average number of points scored by the players. 3.Using the struct in #1, write a function that takes an array of soccer players and its size as arguments and returns the index of the player who scored the most points. 4.Using the struct in #1, write a function that sorts an array of soccer players by name. 5.Using the struct in #1, write a function that takes an (unsorted) array of soccer players and its size and a number as arguments, and returns the name of the soccer player with that number. It should not do any extra unnecessary work. 6.Define a function to find a given target value in an array, but use pointer notation rather than array notation whenever possible. 7.Write a swap function, that swaps the values of two variables in main, but use…arrow_forwardI wrote this code in c programming. what this code should do is ask how many times its going to run and run that many times(this works), ask how many books there are in a place(this works), then ask the max number of pages you want to read(this works), then asks how many pages there are in a book and put that into an array(works), then sorts the array of pages(works), and then adds the number in the array until you cant which is less than or equal to the max number of pages you want to read, and puts how many books you read(does not work), and then prints out how many books you read. a sample input is 35 206 12 3 10 25 2112 3 6 10 210 319 6 6 3 8 2 12 15 13 7 sample out put should be 345 #include <stdio.h> #include <stdlib.h> void MergeSort(int values[], int start, int end); void Merge(int values[], int start, int middle, int end); void add(int pages[], int count[], long long maxPages, long long total); void Print_Array(int count[], int cases); int main(void) {…arrow_forward
- A pointer variable is just what it sounds like. What is its purpose? Exactly what does it mean to have a "dynamic array"? Pointers and dynamic arrays have what relationship?arrow_forwardIn C++ please follow the instructions Write two code blocks -- one code block to declare a bag and its companion type-tracking array (both using the STL vector), and another code block to create and declare a Cat object and put it into the bag. Name the arrays and variables as you wish. Use any data type tracking and any designation for cats -- your choices. Assume that struct Cat is already defined and that all required libraries are properly included -- just write the two separate code blocks, separated by one or more blank lines.arrow_forwardC++ Language Please add an execution chart for this code like the example below. I have provided the code and the example execution chart. : JUST NEED EXECUTION CHARTTT. Thanks Sample Execution Chart Template//your header files// constant size of the array// declare an array of BankAccount objects called accountsArray of size =SIZE// comments for other declarations with variable names etc and their functionality// function prototypes// method to fill array from file, details of input and output values and purpose of functionvoid fillArray (ifstream &input,BankAccount accountsArray[]);// method to find the largest account using balance as keyint largest(BankAccount accountsArray[]);// method to find the smallest account using balance as keyint smallest(BankAccount accountsArray[]);// method to display all elements of the accounts arrayvoid printArray(BankAccount accountsArray[]);int main() {// function calls come here:// give the function call and a comment about the purpose,//…arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
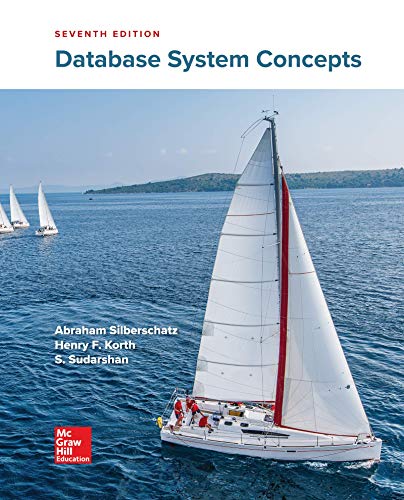
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
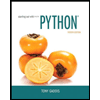
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
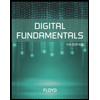
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
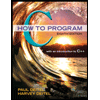
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
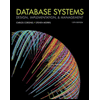
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
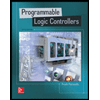
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education