import javax.swing.JFrame; public class Bagel { //----------------------------------------------------------------- // Creates and displays the controls for a bagel shop. //----------------------------------------------------------------- public static void main (String[] args) { JFrame frame = new JFrame ("Bagel Shop"); frame.setDefaultCloseOperation (JFrame.EXIT_ON_CLOSE); frame.getContentPane().add(new BagelControls()); frame.pack(); frame.setVisible(true); } } import java.awt.*; import java.awt.event.*; import javax.swing.*; public class BagelControls extends JPanel { private JComboBox bagelCombo; private JButton calcButton; private JLabel cost; private double bagelCost; public BagelControls() { String[] types = {"Make A Selection...", "Plain", "Asiago Cheese", "Cranberry"}; bagelCombo = new JComboBox(types); calcButton = new JButton("Calc"); cost = new JLabel("Cost = " + bagelCost); setPreferredSize (new Dimension (400, 100)); setBackground(Color.cyan); add(bagelCombo); add(calcButton); add(cost); calcButton.addActionListener (new CalcListener()); } private class CalcListener implements ActionListener { public void actionPerformed (ActionEvent event) { int bagelType = bagelCombo.getSelectedIndex(); switch(bagelType) { case 0: JOptionPane.showMessageDialog(null, "Please select a bagel selection."); break; case 1: bagelCost = 1.00; break; case 2: bagelCost = 2.00; break; case 3: bagelCost = 3.00; break; } cost.setText("Cost = " + bagelCost); } }
BAGEL FILES
import javax.swing.JFrame;
public class Bagel
{
//-----------------------------------------------------------------
// Creates and displays the controls for a bagel shop.
//-----------------------------------------------------------------
public static void main (String[] args)
{
JFrame frame = new JFrame ("Bagel Shop");
frame.setDefaultCloseOperation (JFrame.EXIT_ON_CLOSE);
frame.getContentPane().add(new BagelControls());
frame.pack();
frame.setVisible(true);
}
}
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class BagelControls extends JPanel
{
private JComboBox bagelCombo;
private JButton calcButton;
private JLabel cost;
private double bagelCost;
public BagelControls()
{
String[] types = {"Make A Selection...", "Plain",
"Asiago Cheese", "Cranberry"};
bagelCombo = new JComboBox(types);
calcButton = new JButton("Calc");
cost = new JLabel("Cost = " + bagelCost);
setPreferredSize (new Dimension (400, 100));
setBackground(Color.cyan);
add(bagelCombo);
add(calcButton);
add(cost);
calcButton.addActionListener (new CalcListener());
}
private class CalcListener implements ActionListener
{
public void actionPerformed (ActionEvent event)
{
int bagelType = bagelCombo.getSelectedIndex();
switch(bagelType)
{
case 0:
JOptionPane.showMessageDialog(null, "Please select a bagel selection.");
break;
case 1:
bagelCost = 1.00;
break;
case 2:
bagelCost = 2.00;
break;
case 3:
bagelCost = 3.00;
break;
}
cost.setText("Cost = " + bagelCost);
}
}
}


Trending now
This is a popular solution!
Step by step
Solved in 2 steps

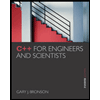
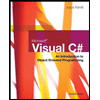
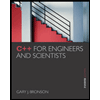
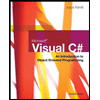
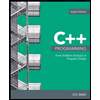
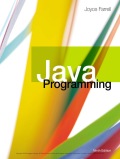