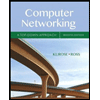
Write a class called Candidate to store details of a candidate in the election.
The class must define fields to store the following details:
• The name of the candidate. The value will be a String which will
always have a length of at least 1 character.
• The number of votes the candidate has received. This is an integer
value. The value will always be greater-than or equal to zero.
The class must have a single constructor that takes the name of a candidate.
You do not need to check that the value passed to the constructor is valid.
You may assume that it always will be. In other words, you may assume that
the name String will be at least one character long. The initial value of votes
must always be zero when the object is constructed and it is not passed as a
parameter to the constructor.
The class must define the following methods:
• An accessor (get) method for each field.
• A method called voteFor that adds 1 to the votes field.
• A method called print to print the values of the fields on a single line
in exactly the following format:
Dodgy Drew has 5 votes.
where "Dodgy Drew" is an example of a String in the name field and 5
is an example of the current value in the votes field. Each Candidate
object will have its own particular values for these fields.
THEN
Write a class called Election that will store Candidate objects for a
particular election event.
Write the Election class with the following features:
• It must use a suitable list-style collection class from the java.util
package to store multiple Candidate objects;
• An addCandidate method that takes a Candidate object as
parameter and stores it in the collection. You may assume that no two
Candidate objects with the same name will be stored via this method.
Note that this method must not create a Candidate object.
• A getCandidates method that returns the collection of Candidate
objects.
• A printAll method that iterates over the collection and prints out
details of all the candidates in the collection by calling each Candidate
object’s print method. At the end of the method, a summary of the
total number of votes cast so far for all candidates must be printed. For
instance:
Dodgy Drew has 5 votes.
Trustworthy Taylor has 12 votes.
Posh Phil has 6 votes.
Total votes cast: 23
• A findCandidate method that takes a String as a parameter and
returns the Candidate object whose name field exactly matches the
parameter’s value. If there is no match, return null. It is guaranteed
that all candidates will have different names.
• A findTopCandidates method that takes a single integer as a
parameter (representing a number of votes) and returns a separate list
of all Candidate objects whose votes field has a value greater-than or
equal-to the parameter’s value.
No Candidate objects are to be removed from the Election object’s list
and the list object returned must be a different object from the Election
object’s list of all donors. The list must be empty if no candidate has
sufficient votes.
• A removeLast method that finds the Candidate(s) with the fewest
number of votes and removes them from the collection. The method
must return the number of candidates removed.
The following rules apply: If more than one candidate has the fewest
number of votes then all of those matching candidates must be
removed, unless removing them all would mean that there were no
candidates left. For instance:
o Three candidates with 5, 4 and 3 votes each: the one with 3
votes would be removed, leaving two remaining. The method
returns 1.
o Three candidates with 5, 3 and 3 votes each: the ones with 3
votes would be removed, leaving one remaining. The method
returns 2.
o Three candidates with 3, 3 and 3 votes each: no one would be
removed, leaving three remaining. The method returns 0.

Step by stepSolved in 2 steps with 4 images

is this just for the candidate class or is the election class included?
is this just for the candidate class or is the election class included?
- A field has access that is somewhere between public and private. static final Opackage Oprotectedarrow_forwardCreate a class Divide with the following fields, constructors and methods:Fields: create two double fields called numerator and denominator Constructors:- a no-argument constructor- a constructor with two parameters Methods:- write a getter to return the result of numerator divided by denominator- Create 4 overloaded calculate methods. Note: Division would be numerator divided by denominator 1. method receives two double parameters and returns the result of the division as double 2. method receives two integer parameters and returns the result of the division as double 3. method receives one integer parameter and another as double parameter and returns the result of the division as int 4. method receives two String parameters and returns the result of the division as int Create a demo class to test the two constructors, all the methods and display the returned values. You can hard code the parameters values when calling the constructor and the methods.arrow_forwardCreate a class called Staff that has the following attributes: Staff String - FirstName String - LastName String - Department String - Title Integer - UIN Staff() Staff(FirstName, LastName, Department, Title, UIN) getFirstName() - String setFirstName(String) - void getLastName() - String setLastName(String) - void getDepartment() - String setDepartment(String) - void getTitle() - String setTitle(String) - void getUIN() - Integer setUIN(Integer) - void toString() - String compareTo(Staff) - Integer equals(Staff) - Boolean PreviousNextarrow_forward
- 1. Create a class as described below: Class Name: Room Fields: standard room fee amenities total cost Constructors:A default constructor to initialize the fields. The standard room fee field should be set to $200 and the amenities field to zero. Another constructor with one argument. It should assign this value to the standard room fee and the amenities field to the default value of $75. Methods: getStandardFee() - This methods returns the standard room fee field value. getAmenities() - This method returns the amenities field value. getTotalCost() - This method returns the total cost field value. calcTotalCost() - This method calculated the total cost for an instance of the class setStandard Fee() - This method has one argument representing the standard room fee. setAmenities() - This method has one argument representing the amenities for the room.arrow_forwardDesign a class named Fan to represent a fan. The class contains: VideoNote The Fan class ▪ Three constants named SLOW, MEDIUM, and FAST with the values 1, 2, and 3 to denote the fan speed. ▪ A private int data field named speed that specifies the speed of the fan (the default is SLOW). ▪ A private boolean data field named on that specifies whether the fan is on (the default is false). ▪ A private double data field named radius that specifies the radius of the fan (the default is 5). ▪ A string data field named color that specifies the color of the fan (the default is blue). ▪ The accessor and mutator methods for all four data fields. ▪ A no-arg constructor that creates a default fan. ▪ A method named toString() that returns a string description for the fan. If the fan is on, the method returns the fan speed, color, and radius in one combined string. If the fan is not on, the method returns the fan color and radius along with the string "fan is off" in one combined string. Draw the UML…arrow_forwardCreate a class of Dog, some of the differences you might have listed out maybe breed, age, size, color, etc. Then create characteristics (breed, age, size, color) can form a data member for your object. Then list out the common behaviors of these dogs like sleep, sit, eat, etc. Design the class dogs with objects and methods.arrow_forward
- 1. Create a class called Elevator that can be moved between floors in an N-storey building. Elevator uses a constructor to initialize the number of floors (N) in the building when the object is instantiated. Elevator also has a default constructor that creates a five- (5) storey building. The Elevator class has a termination condition that requires the elevator to be moved to the main (i.e., first) floor when the object is cleaned up. Write a finalize() method that satisfies this termination condition and verifies the condition by printing a message to the output, Elevator ending: elevator returned to the first floor. In main(), test at least five (5) possible scenarios that can occur when Elevator is used in a building with many floors (e.g., create, move from one floor to another, etc.). Hint: Termination occurs when the instance is set to null. You may wish to investigate “garbage collection” for this exercise.arrow_forwardDesign a class named octopus that holds attributes for an octopus named weight and color. Include methods to set and get each of these attributes. Create the class diagram and write the class pseudocode that defines the class. Create a small program that declares two octopus objects and set the octopus's name weight and color attributes the program must also output each octopus's name weight and color after they are set. Please write out the pseudocode. I have a hard time with this.arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
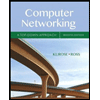
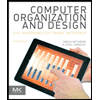
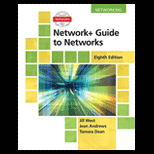
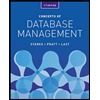
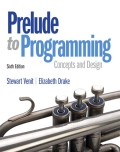
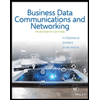