Below for each class you find a UML and description of the public interface. Implementing the public interface as described is madatory. There's freedom on how to implement these classes.The private properties and private methods are under your control.. There are multiple ways of implementing all these classes. Feel free to add private properties and methods. For each object, it's mandatory to create a header file (.h), implementation file (.cpp) and a driver. Blank files are included. The header should have the class definition in it. The implementation file should contain the implementations of the methods laid out in the header fine. And finally the Driver should test/demonstrate all the features of the class. It's best to develop the driver as the class is being written. Check each section to see if there are added additional requirements for the driver. Two test suites are included so that work can be checked. It's important to implement the drivers to test and demonstrate Classes functionality. Song.h : #include #include using namespace std; #ifndef SONG_H #define SONG_H #endif //SONG_H SongTest.cpp : #define CATCH_CONFIG_MAIN #include "../Song.h" #include #include TEST_CASE("Should be able to create a Song") { Song *song = new Song("Bohemian Rhapsody", "Queen", 5, 54, "Rock", 1975); } TEST_CASE("Should be able to get the song title") { Song song2("Shape of You", "Ed Sheeran", 3, 54, "Pop", 2017); string title = song2.getTitle(); REQUIRE_THAT("Shape of You", Catch::Matchers::Equals(title)); } TEST_CASE("Should be able to get the song artist") { Song song3("Imagine", "John Lennon", 3, 3, "Rock", 1971); string title = song3.getArtist(); REQUIRE_THAT("John Lennon", Catch::Matchers::Equals(title)); } TEST_CASE("Should be able to get the song duration") { Song song3("Imagine", "John Lennon", 3, 3, "Rock", 1971); string duration = song3.getDuration(); REQUIRE_THAT("3:03", Catch::Matchers::Equals(duration)); } TEST_CASE("Should be able to get the song genre") { Song song3("Imagine", "John Lennon", 3, 3, "Rock", 1971); string genre = song3.getGenre(); REQUIRE_THAT("Rock", Catch::Matchers::Equals(genre)); } TEST_CASE("Should be able to get the song release year") { Song song3("Imagine", "John Lennon", 3, 3, "Rock", 1971); int releaseYear = song3.getReleaseYear(); REQUIRE(1971 == releaseYear); } TEST_CASE("Should be able to get a string representation of the song") { Song *song = new Song("Bohemian Rhapsody", "Queen", 5, 54, "Rock", 1975); CHECK_THAT(song->toString(), Catch::Matchers::ContainsSubstring("Bohemian Rhapsody")); CHECK_THAT(song->toString(), Catch::Matchers::ContainsSubstring("Queen")); } TEST_CASE("Should be able to add a comment to a song") { Song *song = new Song("Bohemian Rhapsody", "Queen", 5, 54, "Rock", 1975); song->addComment("One of the greatest rock songs ever!"); } TEST_CASE("Outputting the Song as a string should include song's comments") { Song *song = new Song("Bohemian Rhapsody", "Queen", 5, 54, "Rock", 1975); song->addComment("One of the greatest rock songs ever!"); song->addComment("Amazing vocals!"); CHECK_THAT(song->toString(), Catch::Matchers::ContainsSubstring("One of the greatest rock songs ever!")); CHECK_THAT(song->toString(), Catch::Matchers::ContainsSubstring("Amazing vocals!")); } SongDriver.cpp: #include using namespace std; #include "Road.h" int main() { std::cout << "Hello, Song Driver!" << std::endl; return 0; } Song.cpp :
Below for each class you find a UML and description of the public interface. Implementing the public interface as described is madatory. There's freedom on how to implement these classes.The private properties and private methods are under your control.. There are multiple ways of implementing all these classes. Feel free to add private properties and methods. For each object, it's mandatory to create a header file (.h), implementation file (.cpp) and a driver. Blank files are included. The header should have the class definition in it. The implementation file should contain the implementations of the methods laid out in the header fine. And finally the Driver should test/demonstrate all the features of the class. It's best to develop the driver as the class is being written. Check each section to see if there are added additional requirements for the driver. Two test suites are included so that work can be checked. It's important to implement the drivers to test and demonstrate Classes functionality.
Song.h :
#include <vector>
using namespace std;
#ifndef SONG_H
#define SONG_H
#endif //SONG_H
#include "../Song.h"
#include <catch2/catch_test_macros.hpp>
#include <catch2/matchers/catch_matchers_string.hpp>
TEST_CASE("Should be able to create a Song") {
Song *song = new Song("Bohemian Rhapsody", "Queen", 5, 54, "Rock", 1975);
}
TEST_CASE("Should be able to get the song title") {
Song song2("Shape of You", "Ed Sheeran", 3, 54, "Pop", 2017);
string title = song2.getTitle();
REQUIRE_THAT("Shape of You", Catch::Matchers::Equals(title));
}
TEST_CASE("Should be able to get the song artist") {
Song song3("Imagine", "John Lennon", 3, 3, "Rock", 1971);
string title = song3.getArtist();
REQUIRE_THAT("John Lennon", Catch::Matchers::Equals(title));
}
TEST_CASE("Should be able to get the song duration") {
Song song3("Imagine", "John Lennon", 3, 3, "Rock", 1971);
string duration = song3.getDuration();
REQUIRE_THAT("3:03", Catch::Matchers::Equals(duration));
}
TEST_CASE("Should be able to get the song genre") {
Song song3("Imagine", "John Lennon", 3, 3, "Rock", 1971);
string genre = song3.getGenre();
REQUIRE_THAT("Rock", Catch::Matchers::Equals(genre));
}
TEST_CASE("Should be able to get the song release year") {
Song song3("Imagine", "John Lennon", 3, 3, "Rock", 1971);
int releaseYear = song3.getReleaseYear();
REQUIRE(1971 == releaseYear);
}
TEST_CASE("Should be able to get a string representation of the song") {
Song *song = new Song("Bohemian Rhapsody", "Queen", 5, 54, "Rock", 1975);
CHECK_THAT(song->toString(), Catch::Matchers::ContainsSubstring("Bohemian Rhapsody"));
CHECK_THAT(song->toString(), Catch::Matchers::ContainsSubstring("Queen"));
}
TEST_CASE("Should be able to add a comment to a song") {
Song *song = new Song("Bohemian Rhapsody", "Queen", 5, 54, "Rock", 1975);
song->addComment("One of the greatest rock songs ever!");
}
TEST_CASE("Outputting the Song as a string should include song's comments") {
Song *song = new Song("Bohemian Rhapsody", "Queen", 5, 54, "Rock", 1975);
song->addComment("One of the greatest rock songs ever!");
song->addComment("Amazing vocals!");
CHECK_THAT(song->toString(), Catch::Matchers::ContainsSubstring("One of the greatest rock songs ever!"));
CHECK_THAT(song->toString(), Catch::Matchers::ContainsSubstring("Amazing vocals!"));
}
using namespace std;
#include "Road.h"
int main() {
std::cout << "Hello, Song Driver!" << std::endl;
return 0;
}


Step by step
Solved in 3 steps

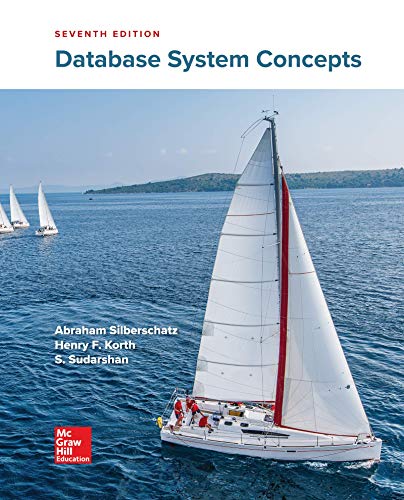
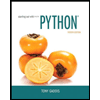
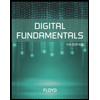
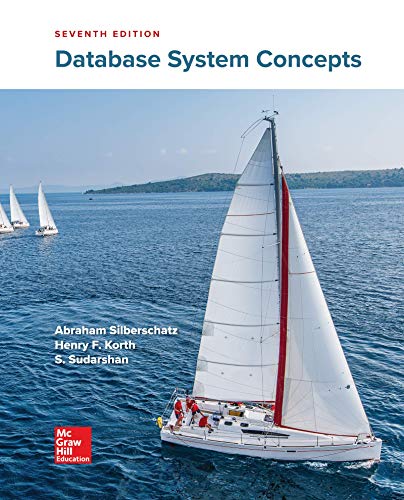
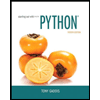
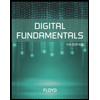
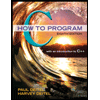
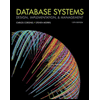
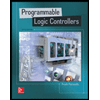