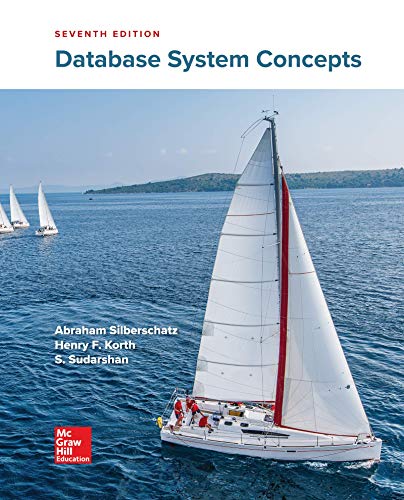
Concept explainers
This assignment is a review of loops. Do not use anything more advanced than a loop, such as programmer-defined functions or arrays or classes.
If you know blackjack, you may be tempted to change the specifications below to make the game more "blackjack-like". Please don't. Be sure to follow these specs exactly and don't try to improve on them. You will be penalized if the specs are not met exactly.
In the card game named 'blackjack' players get two cards to start with, and then they are asked whether or not they want more cards. Players can continue to take as many cards as they like. Their goal is to get as close as possible to a total of 21 without going over. Face cards have a value of 10.
Write a command line game that plays a simple version of blackjack. The program should generate a random number between 1 and 10 each time the player gets a card. Each of the values (1 through 10) must be equally likely. (In other words, this won't be like real black jack where getting a 10 is more likely than getting some other value, because in real black jack all face cards count as 10.) It should keep a running total of the player's cards, and ask the player whether or not it should deal another card. If the player hits 21 exactly, the program should print "Congratulations!" and then ask if the player wants to play again. If the player exceeds 21, the program should print "Bust" and then ask if the player wants to play again. Sample output for the game is provided below. Your program should produce the same output.
If you'd like a little refresher on random number generation, see lesson 7.3.
Important Submission Note:
In order to make your code pass the zyBooks tests, you will need to comment out the line where you seed the random number generator (using srand()).
> First cards: 3, 2 > Total: 5 > Do you want another card? (y/n): y > Card: 6 > Total: 11 > Do you want another card? (y/n): y > Card: 7 > Total: 18 > Do you want another card? (y/n): n > Would you like to play again? (y/n): y > > First cards: 10, 2 > Total: 12 > Do you want another card? (y/n): y > Card: 6 > Total: 18 > Do you want another card? (y/n): y > Card: 7 > Total: 25 > Bust. > Would you like to play again? (y/n): n
Suggestion
Be sure to use iterative development. Start with a small amount of functionality, and then grow it gradually. This way you can compile and run your program after each statement that you write.
You might start by just generating a single card. The program execution might look like this:
> First card: 3
Then generate two cards
> First cards: 3, 2
Next add a variable to store the total, and a statement to show its value:
> First cards: 3, 2 > Total: 5
Next read in a user response and print out the value that was entered
> First cards: 3, 2 > Total: 5 > Do you want another card? (y/n): y > You entered: y
Next you might add a loop, without yet adding the blackjack logic
> First cards: 3, 2 > Total: 5 > Do you want another card? (y/n): y > Do you want another card? (y/n): y > Do you want another card? (y/n): n
Now move the display of the total to the loop
> First cards: 3, 2 > Total: 5 > Do you want another card? (y/n): y > Total: 5 > Do you want another card? (y/n): y > Total: 5 > Do you want another card? (y/n): n
Your next steps might be something like this:
- Generate a new card in each loop and display the value
- Update the total in each loop.
- Check to see if the user busts in each loop
- Wrap the game in loop that handles the Play-Again functionality
Further Hints
If your program is well-structured, output such as "Congratulations!", "Bust!", and "Would you like to play again?" will only occur once.

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 3 images

- Can someone help me set up this code. I am very confused. All information is in the photos. There is also a template but I don’t know how to use it (if you would like to see the template contact me it won’t let me add more than 2 photos). Please please help! The instructions are on the photos as well.arrow_forwardHi, I am making a elevator simulation and I need help in making the passenger classes using polymorphism, I am not sure what to implement in the comment that says logic. Any help is appreciated. There are 4 types of passengers in the system:Standard: This is the most common type of passenger and has a request percentage of 70%. Standard passengers have no special requirements.VIP: This type of passenger has a request percentage of 10%. VIP passengers are given priority and are more likely to be picked up by express elevators.Freight: This type of passenger has a request percentage of 15%. Freight passengers have large items that need to be transported and are more likely to be picked up by freight elevators.Glass: This type of passenger has a request percentage of 5%. Glass passengers have fragile items that need to be transported and are more likely to be picked up by glass elevators. This is what i have so far public abstract class Passenger { protected String type; protected int…arrow_forwardIts missing order and tester class plus look at the question information again i need you to redo it with the new classes tooarrow_forward
- I am not sure how to do this problem recursively DO NOT use System.exit(). DO NOT add project or package statements. DO NOT change the class name. DO NOT change the headers of ANY of the given methods. DO NOT add any new class fields (instance variables). ONLY display the result as specified by the example for each problem. DO NOT print other messages, follow the examples for each problem. You may USE the StdOut library. On RecursiveAppend.java write a recursive method appendNTimes that receives two arguments, a string and an integer. The method appendNTimes returns the original string appended to the original string n times. Use the following method header:public static String appendNTimes (String original, int n) Examples: appendNTimes("cat", 0) returns “cat” appendNTimes("cat", 1) returns “catcat” appendNTimes("cat", 2) returns “catcatcat” The following restrictions apply to method appendNTimes: the CODE MUST BE RECURSIVE Do not use loops (while, do/while, or for).…arrow_forwardPlease help with 3rd section (in images below), code for first and second class included below. Do not change the function names or given starter code in the script. Each class has different requirements, read them carefully. Do not use the exec or eval functions, nor are you allowed to use regular expressions (re module). All methods that output a string must return the string, not print it. If you are unable to complete a method, use the pass statement to avoid syntax errors. class Stack: def __init__(self): self.stack = [] def pop(self): if len(self.stack) < 1: return None return self.stack.pop() def push(self, item): self.stack.append(item) def size(self): return len(self.stack) def peek(self): return self.stack[self.size() - 1] def isEmpty(self): if len(self.stack) < 1: return True return False class Calculator: def __init__(self): self.__expr = None…arrow_forwardI need help in improving my Elevator class and it's subclasses code. This is an elevator simulator that uses polymorphism and object-oriented programming to simulate the movement of elevators in a building with multiple types of passengers and elevators. There are 4 types of elevators in the system:StandardElevator: This is the most common type of elevator and has a request percentage of 70%. Standard elevators have a maximum capacity of 10 passengers.ExpressElevator: This type of elevator has a request percentage of 20%. Express elevators have a maximum capacity of 8 passengers and are faster than standard elevators.FreightElevator: This type of elevator has a request percentage of 5%. Freight elevators have a maximum capacity of 5 passengers and are designed to transport heavy items.GlassElevator: This type of elevator has a request percentage of 5%. Glass elevators have a maximum capacity of 6 passengers and are designed to transport fragile item Classes should have this: Elevator {…arrow_forward
- This is exercise builds on the previous Exercise (Inheritance 2) classes. In this series of exercises we'll take the StudentType class and expand it to also hold a list of courses that the student has taken. Starting out we want to build a CourseType class that will hold the basic course information: Create a CourseType class. (Keep it simple at this point. You don't need to put in "extra" members just to make it a bigger class.) You should create this class with its own .cpp and .h file, but include it in a "project" with your already existing StudentType class. (You can use your own StudentType solution or the one provided from the previous exercise.) Note: At this point you shouldn't be changing anything in the existing StudentType files! Make sure you test your new CourseType class by declaring an object and testing at least it's constructor. Submit: Commented test, implementation and header files (.cpp and .h) for new CourseType along with previous StudentType and PersonType…arrow_forwardIt implies testing in a "BLACK Box." What different variants exist on this theme?arrow_forwardI need help in improving my Elevator class and it's subclasses code. This is an elevator simulator that uses polymorphism and object-oriented programming to simulate the movement of elevators in a building with multiple types of passengers and elevators. There are 4 types of elevators in the system:StandardElevator: This is the most common type of elevator and has a request percentage of 70%. Standard elevators have a maximum capacity of 10 passengers.ExpressElevator: This type of elevator has a request percentage of 20%. Express elevators have a maximum capacity of 8 passengers and are faster than standard elevators.FreightElevator: This type of elevator has a request percentage of 5%. Freight elevators have a maximum capacity of 5 passengers and are designed to transport heavy items.GlassElevator: This type of elevator has a request percentage of 5%. Glass elevators have a maximum capacity of 6 passengers and are designed to transport fragile item Classes should have this: Elevator {…arrow_forward
- Please look at the images for the programming question. I am not sure sure where I should start on this. All coding is done in Java, using IntelliJ Idea.arrow_forwardI need help in improving my code. This is an elevator simulator that uses polymorphism and object-oriented programming to simulate the movement of elevators in a building with multiple types of passengers and elevators.The system has 8 elevators, each of which can be one of 4 types of elevators, with a certainpercentage of requests for each type. Similarly, each passenger can be one of 4 types, with adifferent percentage of requests for each type. The simulation should have 4 types of Passengers: Standard: This is the most common type of passenger and has a request percentage of 70%. Standard passengers have no special requirements. VIP: This type of passenger has a request percentage of 10%. VIP passengers are given priority and are more likely to be picked up by express elevators. Freight: This type of passenger has a request percentage of 15%. Freight passengers have large items that need to be transported and are more likely to be picked up by freight elevators. Glass: This type…arrow_forwardhello c++ programming Superhero battle time! Muscles and Bones are not happy with each other and need to come to some kind of understandingWhat are the odds that Muscles will come out ahead? What about Bones? Start with this runner file that will handle the simulation, and don't modify the file in any way (runner file is below)Spoiler time... When it's all said and done, Muscles should win about 72% of the time, and Bones about 28% Create files TwoAttackSupe.h and TwoAttackSupe.cpp that collectively contain the following functionality: The class name will be TwoAttackSupe The class will be a base class for what you see below in the next blurb The class has one member variable, an integer value that maintains a health value Its only constructor takes one parameter, which is the health value Make sure to set up a virtual destructor since this is a base class Develop a function called IsDefeated() which returns a bool Return true if the health value is less than or equal to 0 Return…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
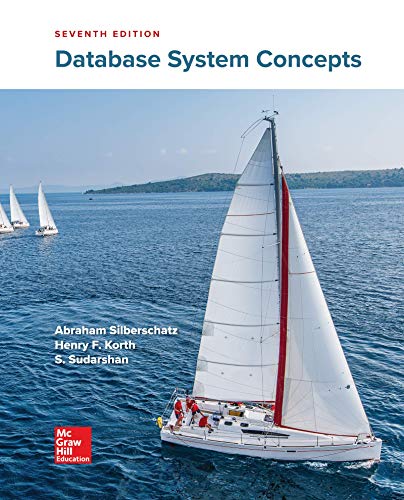
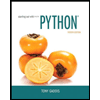
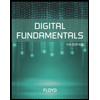
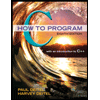
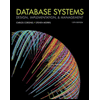
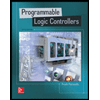