C Sharp, I am trying to get my console to display the vehicle information, by using a base class of Vehicle and derived classes Car, and Truck. I have to add an interface to add and remove cars. But for now, I am just trying to get the vehicle information to display from the Car class. For some reason I am not able to override my methods in the derived classes. What am I am doing wrong ? using CarRentalCompany; using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace CarRentalCompany {//Define an abstract class Vehicle with properties like Make, Model, Year, Status, and Notes public abstract class Vehicle { public string make; public string model; public int year; public string status; public string notes; //Method to Display vechile information public void DisplayVehicleInfomration() { } } public class Car : Vehicle, IRentable { private string v; public Car(string v) { this.v = v; } //Construtor new object is created public Car(string make, string model, int year, string status, string notes) { this.make = make; this.model = model; this.year = year; this.status = status; this.notes = notes; } // public override void DisplayVehicleInfomration() { Console.WriteLine("The make of this vechile is + {make} "); Console.WriteLine($"The model of the vechile is + {model}"); Console.WriteLine($"The yerr of the vechile is + {year}"); Console.WriteLine($"The status of the vechile is + {status}"); Console.WriteLine($"Vechile notes : + {notes}"); } } public class Truck : Vehicle, IRentable { public Truck (string make, string model, int year, string status, string notes) { this.make = make; this.model = model; this.year = year; this.status = status; this.notes = notes; } //Why can't I override ? public override void DispalyVechileInfomration() { } } } //Use an interface IRentable for vehicles that can be rented, ////including methods like Rent and Return. ///Create the name with an I in front and Capital the 1st letter = Interface ///Interfaces are public - so no need to add modifers public interface IRentable { // CarRent(); // CarReturn(); }; using CarRentalCompany;// make sure to list other classes here using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; // need to fix name spacing // How do I fix the namespaces ? namespace CarRentalCompnay { class ProgramA { static void Main(string[] args) { ////Store the objects in a Collection object (List, Array, HashSet, etc...) objects in the collection will be of type Shape Vehicle[] arrayOfVehicles = new Vehicle[5]; arrayOfVehicles[0] = new Car("Toytoa, Camery, 2024,available, notes: Scratch on driver side door "); arrayOfVehicles[1] = new Car(" "); arrayOfVehicles[2] = new Car(" "); // arrayOfVehicles[3] = new Truck(" "); // arrayOfVehicles[4] = new Truck(""); foreach (Vehicle vehicle in arrayOfVehicles) {//How do I get my console to dispaly car information ? Console.WriteLine($" The current {vehicle} is a "); } } } }
C Sharp, I am trying to get my console to display the vehicle information, by using a base class of Vehicle and derived classes Car, and Truck. I have to add an interface to add and remove cars. But for now, I am just trying to get the vehicle information to display from the Car class. For some reason I am not able to override my methods in the derived classes. What am I am doing wrong ? using CarRentalCompany; using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace CarRentalCompany {//Define an abstract class Vehicle with properties like Make, Model, Year, Status, and Notes public abstract class Vehicle { public string make; public string model; public int year; public string status; public string notes; //Method to Display vechile information public void DisplayVehicleInfomration() { } } public class Car : Vehicle, IRentable { private string v; public Car(string v) { this.v = v; } //Construtor new object is created public Car(string make, string model, int year, string status, string notes) { this.make = make; this.model = model; this.year = year; this.status = status; this.notes = notes; } // public override void DisplayVehicleInfomration() { Console.WriteLine("The make of this vechile is + {make} "); Console.WriteLine($"The model of the vechile is + {model}"); Console.WriteLine($"The yerr of the vechile is + {year}"); Console.WriteLine($"The status of the vechile is + {status}"); Console.WriteLine($"Vechile notes : + {notes}"); } } public class Truck : Vehicle, IRentable { public Truck (string make, string model, int year, string status, string notes) { this.make = make; this.model = model; this.year = year; this.status = status; this.notes = notes; } //Why can't I override ? public override void DispalyVechileInfomration() { } } } //Use an interface IRentable for vehicles that can be rented, ////including methods like Rent and Return. ///Create the name with an I in front and Capital the 1st letter = Interface ///Interfaces are public - so no need to add modifers public interface IRentable { // CarRent(); // CarReturn(); }; using CarRentalCompany;// make sure to list other classes here using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; // need to fix name spacing // How do I fix the namespaces ? namespace CarRentalCompnay { class ProgramA { static void Main(string[] args) { ////Store the objects in a Collection object (List, Array, HashSet, etc...) objects in the collection will be of type Shape Vehicle[] arrayOfVehicles = new Vehicle[5]; arrayOfVehicles[0] = new Car("Toytoa, Camery, 2024,available, notes: Scratch on driver side door "); arrayOfVehicles[1] = new Car(" "); arrayOfVehicles[2] = new Car(" "); // arrayOfVehicles[3] = new Truck(" "); // arrayOfVehicles[4] = new Truck(""); foreach (Vehicle vehicle in arrayOfVehicles) {//How do I get my console to dispaly car information ? Console.WriteLine($" The current {vehicle} is a "); } } } }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
100%
C Sharp, I am trying to get my console to display the vehicle information, by using a base class of Vehicle and derived classes Car, and Truck. I have to add an interface to add and remove cars. But for now, I am just trying to get the vehicle information to display from the Car class. For some reason I am not able to override my methods in the derived classes. What am I am doing wrong ? using CarRentalCompany;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace CarRentalCompany
{//Define an abstract class Vehicle with properties like Make, Model, Year, Status, and Notes
public abstract class Vehicle
{
public string make;
public string model;
public int year;
public string status;
public string notes;
//Method to Display vechile information
public void DisplayVehicleInfomration() { }
}
public class Car : Vehicle, IRentable
{
private string v;
public Car(string v)
{
this.v = v;
}
//Construtor new object is created
public Car(string make, string model, int year, string status, string notes)
{
this.make = make;
this.model = model;
this.year = year;
this.status = status;
this.notes = notes;
}
//
public override void DisplayVehicleInfomration()
{
Console.WriteLine("The make of this vechile is + {make} ");
Console.WriteLine($"The model of the vechile is + {model}");
Console.WriteLine($"The yerr of the vechile is + {year}");
Console.WriteLine($"The status of the vechile is + {status}");
Console.WriteLine($"Vechile notes : + {notes}");
}
}
public class Truck : Vehicle, IRentable
{
public Truck (string make, string model, int year, string status, string notes)
{
this.make = make;
this.model = model;
this.year = year;
this.status = status;
this.notes = notes;
}
//Why can't I override ?
public override void DispalyVechileInfomration() {
}
}
}
//Use an interface IRentable for vehicles that can be rented,
////including methods like Rent and Return.
///Create the name with an I in front and Capital the 1st letter = Interface
///Interfaces are public - so no need to add modifers
public interface IRentable
{
// CarRent();
// CarReturn();
};
using CarRentalCompany;// make sure to list other classes here
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
// need to fix name spacing // How do I fix the namespaces ?
namespace CarRentalCompnay
{
class ProgramA
{
static void Main(string[] args)
{ ////Store the objects in a Collection object (List, Array, HashSet, etc...) objects in the collection will be of type Shape
Vehicle[] arrayOfVehicles = new Vehicle[5];
arrayOfVehicles[0] = new Car("Toytoa, Camery, 2024,available, notes: Scratch on driver side door ");
arrayOfVehicles[1] = new Car(" ");
arrayOfVehicles[2] = new Car(" ");
// arrayOfVehicles[3] = new Truck(" ");
// arrayOfVehicles[4] = new Truck("");
foreach (Vehicle vehicle in arrayOfVehicles)
{//How do I get my console to dispaly car information ?
Console.WriteLine($" The current {vehicle} is a ");
}
}
}
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
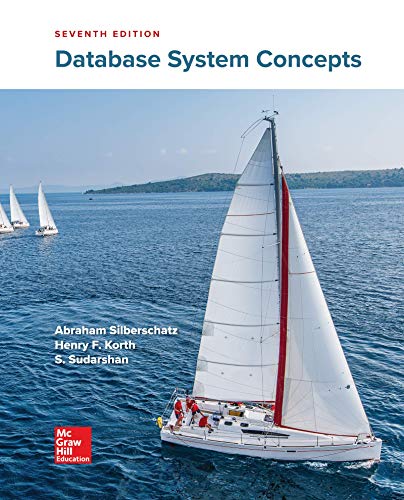
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
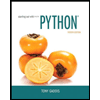
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
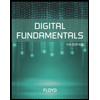
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
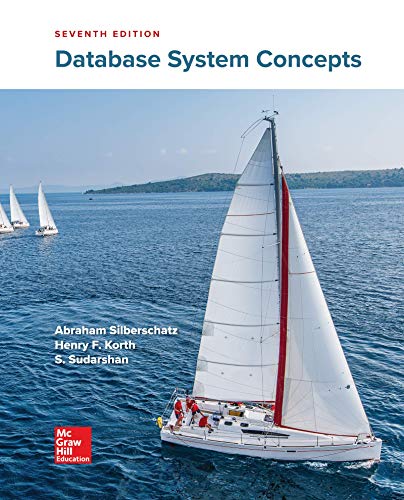
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
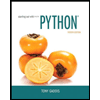
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
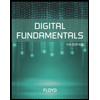
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
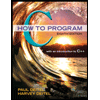
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
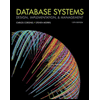
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
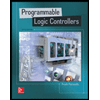
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education