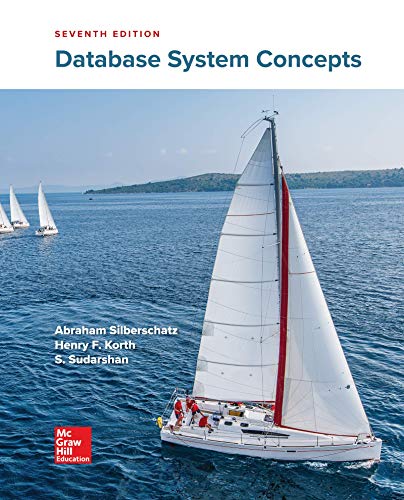
fix the code to able to end the program when press "q". PLease Thank you so much
#include <iostream>
#include <cmath>
class Currency {
protected:
int whole;
int fraction;
virtual std::string get_name() = 0;
public:
Currency() {
whole = 0;
fraction = 0;
}
Currency(double value) {
if (value < 0)
throw "Invalid value";
whole = int(value);
fraction = std::round(100 * (value - whole));
}
Currency(const Currency& curr) {
whole = curr.whole;
fraction = curr.fraction;
}
int get_whole() { return whole; }
int get_fraction() { return fraction; }
void set_whole(int w) {
if (w >= 0)
whole = w;
}
void set_fraction(int f) {
if (f >= 0 && f < 100)
fraction = f;
}
void add(const Currency* curr) {
whole += curr->whole;
fraction += curr->fraction;
if (fraction > 100) {
whole++;
fraction %= 100;
}
}
void subtract(const Currency* curr) {
if (!isGreater(*curr))
throw "Invalid Subtraction";
whole -= curr->whole;
if (fraction < curr->fraction) {
fraction = fraction + 100 - curr->fraction;
whole--;
}
else {
fraction -= curr->fraction;
}
}
bool isEqual(const Currency& curr) {
return curr.whole == whole && curr.fraction == fraction;
}
bool isGreater(const Currency& curr) {
if (whole < curr.whole)
return false;
if (whole == curr.whole && fraction < curr.fraction)
return false;
return true;
}
void print() {
std::cout << whole << "." << fraction << " " << get_name() << std::endl;
}
};
class Krone : public Currency {
protected:
std::string name = "Krone";
std::string get_name() {
return name;
}
public:
Krone() : Currency() { }
Krone(double value) : Currency(value) { }
Krone(Krone& curr) : Currency(curr) { }
};
class Soum : public Currency {
protected:
std::string name = "Soum";
std::string get_name() {
return name;
}
public:
Soum() : Currency() { }
Soum(double value) : Currency(value) { }
Soum(Krone& curr) : Currency(curr) { }
};
int main() {
Currency* currencies[2] = { new Soum(), new Krone() };
while (true) {
currencies[0]->print();
currencies[1]->print();
char oprr;
char oprd;
double value;
char curr[10];
std::cin >> oprr >> oprd >> value >> curr;
std::string currency(curr);
if (oprr == 'q') {
return 0;
}
try {
switch (oprr) {
case 'a':
if (oprd == 's' && currency == "Soum")
currencies[0]->add(new Soum(value));
else if (oprd == 'k' && currency == "Krone")
currencies[1]->add(new Krone(value));
else
throw "Invalid Addition";
break;
case 's':
if (oprd == 's' && currency == "Soum")
currencies[0]->subtract(new Soum(value));
else if (oprd == 'k' && currency == "Krone")
currencies[1]->subtract(new Krone(value));
else
throw "Invalid Subtraction";
break;
default:
throw "Invalid Operator";
}
}
catch (const char e[]) {
std::cout << e << std::endl;
}
}
}
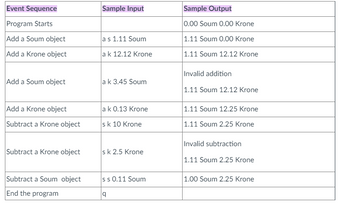

Step by stepSolved in 3 steps with 1 images

- #include <string>#include <iostream>#include <vector>#include <sstream>#include <exception>class CAT{public:float weight() const{return weight_;}unsigned int range() const{return range_;}CAT(float weight= 1.0, unsigned int range=1);int set_weight(float);int set_range(unsigned int power);int dig(double hours);int id() const{return id_;}private:float weight_;unsigned int range_;const int id_;};int compare(CAT,CAT); Task: Define the public function int CAT::set_range(unsigned int power) : If the value passed by power is smaller than 100 and at same time range_ is smaller or equal to 100, then range_ is set to the sum of power and 2. After this -1 is the return value of the function. Else If the value passed by power is smaller than range_ , then range_ is to be set to power times 100 and then power times -1 is the return value of the function. Else If the value passed by power is larger or equal to the value of range_ ,then the function should set…arrow_forward#include <iostream> #include <iostream> using namespace std; class Fraction { public: int numerator; int denominator; void set(int n, int d); void print(); Fraction multipliedBy(Fraction f); Fraction dividedBy(Fraction f); Fraction addedTo(Fraction f); Fraction subtract(Fraction f); bool isEqualTo(Fraction f); }; void Fraction::set(int n, int d) { numerator = n; denominator = d; } void Fraction::print() { cout << numerator << "/" << denominator; } Fraction Fraction::multipliedBy(Fraction f) { Fraction results; results.numerator = this -> numerator * f.numerator; results.denominator = this -> denominator * f.denominator; return results; } Fraction Fraction::dividedBy(Fraction f) { Fraction results; results.numerator = this -> numerator * f.denominator; results.denominator = this -> denominator * f.numerator; return results; } Fraction Fraction::addedTo(Fraction f) { Fraction results; results.numerator = (this ->…arrow_forward#include <iostream> using namespace std; int times(int mpr, int mcand) { int prod = 0; while (mpr != 0) { if (mpr % 2 == 1) prod = prod + mcand; mpr /= 2; mcand *= 2; } return prod; } int main(){ int n, m; cout << "Enter two numbers: "; cin >> n >> m; cout << "Product: " << times(n, m) << endl; return 0; } convert the following c++ code into pep/9 assembly languagearrow_forward
- Create an Organization class. Organization has 10 Employees (Hint: You will need an array of pointers to Employee class) Organization can calculate the total amount to be paid to all employees Organization can print the details(name & salary) of all employees note: write code in main,header and function.cpp filearrow_forwardmain.cpp: #include <iostream>#include <iomanip>#include "Calculator.h"using namespace std; int main() {Calculator calc; double num1; double num2; cin >> num1; cin >> num2; cout << fixed << setprecision(1); // 1. The initial value cout << calc.GetValue() << endl; // 2. The value after adding num1 calc.Add(num1); cout << calc.GetValue() << endl; // 3. The value after multiplying by 3 calc.Multiply(3); cout << calc.GetValue() << endl; // 4. The value after subtracting num2 calc.Subtract(num2); cout << calc.GetValue() << endl; // 5. The value after dividing by 2 calc.Divide(2); cout << calc.GetValue() << endl; // 6. The value after calling the clear() method calc.Clear(); cout << calc.GetValue() << endl; return 0;} Calculator.h: #ifndef CALCULATORH#define CALCULATORH class Calculator { public: //…arrow_forward#include <iostream> using namespace std; int times(int mpr, int mcand) { int prod = 0; while (mpr != 0) { if (mpr % 2 == 1) prod = prod + mcand; mpr /= 2; mcand *= 2; } return prod; } int main(){ int n, m; cout << "Enter two numbers: "; cin >> n >> m; cout << "Product: " << times(n, m) << endl; return 0; } convert the following c++ code into pep/9 assembly languagearrow_forward
- #include <stdio.h>#include <stdlib.h> int cent50 = 0;int cent20 = 0;int cent10 = 0;int cent05 = 0; //Function definitionvoid calculateChange(int change) {if(change > 0) {if(change >= 50) {change -= 50;cent50++;} else if(change >= 20) {change -= 20;cent20++;} else if(change >= 10) {change -= 10;cent10++;} else if(change >= 05) {change -= 05;cent05++;}calculateChange(change);}} //Define the functionvoid printChange() { if(cent50)printf("\n50 Cents : %d coins", cent50); if(cent20)printf("\n20 Cents : %d coins", cent20); if(cent10)printf("\n10 Cents : %d coins", cent10); if(cent05)printf("\n05 Cents : %d coins", cent05);cent50 = 0;cent20 = 0;cent10 = 0;cent05 = 0; } //Function's definitionint TakeChange() { int change;printf("\nEnter the amount : ");scanf("%d", &change);return change; }//main functionint main() {//call the functionint change = TakeChange(); //use while-loop to repeatedly ask for input to the userwhile(change != -1){if((change %…arrow_forwardIn C++ struct myGrades { string class; char grade; }; Declare myGrades as an array that can hold 5 sets of data and then set a class string in each position as follows: “Math” “Computers” “Science” “English” “History” and give each class a letter gradearrow_forward// EmployeeBonus.cpp - This program calculates an employee's yearly bonus. #include <iostream> #include <string> using namespace std; int main() { // Declare and initialize variables here string employeeFirstName; string employeeLastName; double numTransactions; double numShifts; double dollarValue; double score; double bonus; const double BONUS_1 = 50.00; const double BONUS_2 = 75.00; const double BONUS_3 = 100.00; const double BONUS_4 = 200.00; // This is the work done in the housekeeping() function cout << "Enter employee's first name: "; cin >> employeeFirstName; cout << "Enter employee's last name: "; cin >> employeeLastName; cout << "Enter number of shifts: "; cin >> numShifts; cout << "Enter number of transactions: "; cin >> numTransactions; cout << "Enter dollar value of transactions: "; cin >> dollarValue; // This is…arrow_forward
- #include using namespace std; void scanPrice (string [], double []); // Do not modify the code double printReceipt(string [], double[]); // Do not modify the code int main () { double sum; string item [3] = {"Vegetable","Egg", "Meat"}; double price [3];| %3D scanPrice (item,price); sum = RrintReceipt (item,price); cout Price: 10 The price of Vegetable is RM 2 The price of Egg is RM 3 The price of Meat is RM 10 Your total expenses is RM 15arrow_forward#include <iostream> using namespace std; int times(int mpr, int mcand) { int prod = 0; while (mpr != 0) { if (mpr % 2 == 1) prod = prod + mcand; mpr /= 2; mcand *= 2; } return prod; } int main(){ int n, m; cout << "Enter two numbers: "; cin >> n >> m; cout << "Product: " << times(n, m) << endl; return 0; } convert the following c++ code into pep/9 assembly languagearrow_forwardmain.c magic.h 2. Got to Believe in MAGIC! (from codeChum) #include 1 #include"magic.h" int main(void) { 2 by Catherine Arellano 3 int num getNum(); isMagic(num); 4 I recently confessed my love to a very beautiful int res Math major student whom I've been crushing on for display(res); return 0; } 8 years and definitely asked her out. But she said 7 that I can only date her if I prove that magic is real through numbers. Eager to win her heart, I researched and came across Magic Number. A magic number is a number which is equal to the product of sum of all digits of this number and reverse of this sum. For example, 1729 is a magic 8 number. I know that this is it and I found it. If you could help me determine if a number is a magic number or not, then I would treat you to lunch forever. Implement the following functions: int getNum(); /*accepts integer input from the user*/ int isMagic (int num); /*returns 1 if num is a magic number, else, return 0. */arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
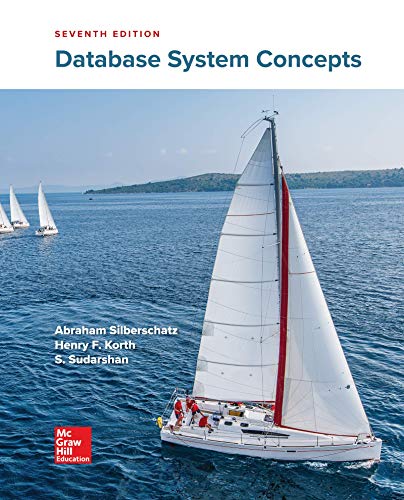
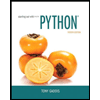
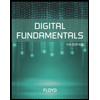
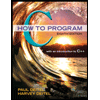
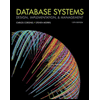
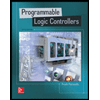