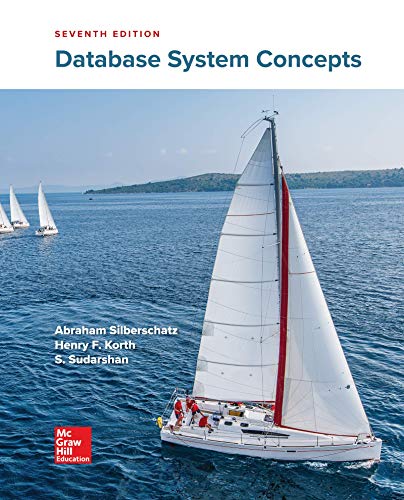
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
// Airline.cpp - This program determines if an airline passenger is
// eligible for a 25% discount.
#include <iostream>
#include <string>
using namespace std;
int main()
{
string passengerFirstName = ""; // Passenger's first name
string passengerLastName = ""; // Passenger's last name
int passengerAge = 0; // Passenger's age
// This is the work done in the housekeeping() function
cout << "Enter passenger's first name: ";
cin >> passengerFirstName;
cout << "Enter passenger's last name: ";
cin >> passengerLastName;
cout << "Enter passenger's age: ";
cin >> passengerAge;
// This is the work done in the detailLoop() function
// Test to see if this customer is eligible for a 25% discount
// This is the work done in the endOfJob() function
return 0;
}
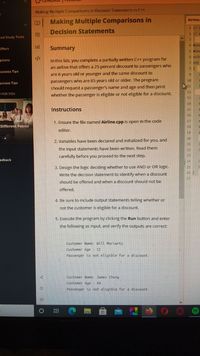
Transcribed Image Text:Making Multiple Comparisons in Decision Statements in C+
Making Multiple Comparisons in
Decision Statements
Airline.
1// A
2 // e
nd Study Tools
3.
4 #inc
Offers
Summary
5 #ind
6 usir
In this lab, you complete a partially written C++ program for
an airline that offers a 25 percent discount to passengers who
ptions
7 int
Success Tips
are 6 years old or younger and the same discount to
6.
passengers who are 65 years old or older. The program
18
uccess Tips
11
should request a passenger's name and age and then print
12
FOR YOU
whether the passenger is eligible or not eligible for a discount.
13
14
Instructions
15
16
17
1. Ensure the file named Airline.cpp is open in the code
Different Points
18
editor.
19
20
2. Variables have been declared and initialized for
you,
and
21
the input statements have been written. Read them
22
carefully before you proceed to the next step.
23
edback
24
3. Design the logic deciding whether to use AND or OR logic.
25
26 }
Write the decision statement to identify when a discount
27
should be offered and when a discount should not be
offered.
4. Be sure to include output statements telling whether or
not the customer is eligible for a discount.
5. Execute the program by clicking the Run button and enter
the following as input, and verify the outputs are correct:
Customer Name: Will Moriarty
Customer Age : 11
Passenger is not eligiblLe for a discount.
Customer Name: James Chung
Customer Age : 64
Passenger is not eligible for a discount.
OO
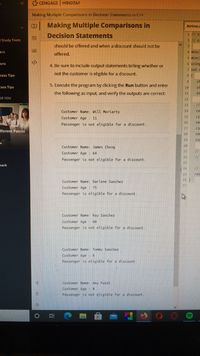
Transcribed Image Text:CENGAGE MINDTAP
Making Multiple Comparisons in Decision Statements in C++
Making Multiple Comparisons in
Airline.c
Decision Statements
1// A
Study Tools
2 // el
should be offered and when a discount should not be
3.
ers
4 #incl
offered.
5 #inc)
ons
4. Be sure to include output statements telling whether or
6 using
7 int
not the customer is eligible for a discount.
cess Tips
st
5. Execute the program by clicking the Run button and enter
=ess Tips
10
st
the following as input, and verify the outputs are correct:
11
in
OR YOU
12
13
//
Customer Name: Will Moriarty
14
co
Customer Age : 11
15
ci
16
co
Passenger is not eligible for a discount.
17
ci
fferent Points
18
COL
19
cir
Customer Name: James Chung
28
21
Customer Age : 64
22
Passenger is not eligible for a discount.
23
pack
24
25
ret
Customer Name: Darlene Sanchez
26 }
27
Customer Age : 75
Passenger is eligible for a discount.
Customer Name: Ray Sanchez
Customer Age : 60
Passenger is not eligible for a discount.
Customer Name: Tommy Sanchez
Customer Age : 6
Passenger is eligible for a discount.
Customer Nane: Amy Patel
Customer Age : 8
Passenger is not eligible for a discount.
日 !
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- products := [5] string {"bread", "milk", "eggs", "butter", "sugar"} price := [5] float64{1.29, 3.75, 3.33, 2.97, 5.28} use a "counting loop" to print the products with their prices example: bread: $1.29 milk: $3.75 eggs: $3.33 butter: $2.97 sugar: $5.28arrow_forward//Below is the starting code for this homework assignment// #include <stdio.h> struct employees { char name[20]; int ssn[9]; int yearBorn, salary; }; struct employees e = {"kim deen", {1,2,3,4,5,6,7,8,9}, 1998, 35000}; void display(struct employees *e) { printf("%s", e->name); printf(" %d%d%d-%d%d-%d%d%d%d", e->ssn[0],e->ssn[1],e->ssn[2],e->ssn[3],e->ssn[4],e->ssn[5],e->ssn[6],e->ssn[7],e->ssn[8]); printf(" %d", e->yearBorn); printf("\n$%d.", e->salary); } int main() { display(&e); return 0; }arrow_forwardWrite a function getNeighbors which will accept an integer array, size of the array and an index as parameters. This function will return a new array of size 2 which stores the neighbors of the value at index in the original array. If this function would result in returning garbage values the new array should be set to values {0,0} instead of values from the array.arrow_forward
- #include using namespace std; int main() { } int kidsInClass1; int kidsInClass2; int numClasses; double kidsAvgMethodl; double kidsAvgMethod2; kidsInClass1= 3; kidsInClass2 = 2; numClasses = 2; Type the program's output kidsAvgMethodl = static_cast (kidsInClass1 + kidsInClass2) /static_cast (numClasses); kidsAvgMethod2 (kidsInClass1 + kidsInClass2) / numClasses; cout << kidsAvgMethod1 << endl; cout << kidsAvgMethod2 << endl; return 0; =arrow_forwardC++ Programmingarrow_forward//Below is the starting code for this homework assignment// #include <stdio.h> struct employees { char name[20]; int ssn[9]; int yearBorn, salary; }; struct employees e = {"kim deen", {1,2,3,4,5,6,7,8,9}, 1998, 35000}; void display(struct employees *e) { printf("%s", e->name); printf(" %d%d%d-%d%d-%d%d%d%d", e->ssn[0],e->ssn[1],e->ssn[2],e->ssn[3],e->ssn[4],e->ssn[5],e->ssn[6],e->ssn[7],e->ssn[8]); printf(" %d", e->yearBorn); printf("\n$%d.", e->salary); } int main() { display(&e); return 0; }arrow_forward
- #include <stdio.h>#include <stdlib.h> int cent50 = 0;int cent20 = 0;int cent10 = 0;int cent05 = 0; //Function definitionvoid calculateChange(int change) {if(change > 0) {if(change >= 50) {change -= 50;cent50++;} else if(change >= 20) {change -= 20;cent20++;} else if(change >= 10) {change -= 10;cent10++;} else if(change >= 05) {change -= 05;cent05++;}calculateChange(change);}} //Define the functionvoid printChange() { if(cent50)printf("\n50 Cents : %d coins", cent50); if(cent20)printf("\n20 Cents : %d coins", cent20); if(cent10)printf("\n10 Cents : %d coins", cent10); if(cent05)printf("\n05 Cents : %d coins", cent05);cent50 = 0;cent20 = 0;cent10 = 0;cent05 = 0; } //Function's definitionint TakeChange() { int change;printf("\nEnter the amount : ");scanf("%d", &change);return change; }//main functionint main() {//call the functionint change = TakeChange(); //use while-loop to repeatedly ask for input to the userwhile(change != -1){if((change %…arrow_forward1 using System; 2 using System.Text; 3 class Program { staticvoid Main(string[] args) { int choice,games_choice; Console.WriteLine("You are on a quest for a rare flavor of chips you saw on an ad. The inside of the bag has an invaluable prize that could change your life forever. You decide to dedicate today to find that bag of chips. Do you go to Walmart or Costco first?"); Console.Write("Press 1 for 'Walmart' or Press 2 for 'Costco'\n"); choice = int.Parse(Console.ReadLine()); if(choice==1) { Console.WriteLine("You begin your meticulous search in the isles of the Walmart Supercenter only to find an empty display of the newly released rare flavor of chips. Out of the corner of your eye you see a nearby shopper with three bags of those rare chips in her basket casually strolling along. Craziness pops in your head and you have to make a quick decision. Your first option is to ask her nicely for one of the bags but that just seems far fetched. Your second option is to challenge her to a…arrow_forward#include <iostream> #include <string> using namespace std; int main() { // Declare and initialize variables. string employeeFirstName; string employeeLastName; double employeeSalary; int employeeRating; double employeeBonus; const double BONUS_1 = .25; const double BONUS_2 = .15; const double BONUS_3 = .10; const double NO_BONUS = 0.00; const int RATING_1 = 1; const int RATING_2 = 2; const int RATING_3 = 3; // This is the work done in the housekeeping() function // Get user input cout << "Enter employee's first name: "; cin >> employeeFirstName; cout << "Enter employee's last name: "; cin >> employeeLastName; cout << "Enter employee's yearly salary: "; cin >> employeeSalary; cout << "Enter employee's performance rating: "; cin >> employeeRating; // This is the work done in the detailLoop() function // Use switch statement to…arrow_forward
- int x1 = 66; int y1 = 39; int d; _asm { } mov EAX, X1; mov EBX, y1; push EAX; push EBX; pop ECX mov d, ECX; What is d in decimal format?arrow_forward#include <iostream> #include <iomanip> #include <string> using namespace std; int main() { constdouble MONSTERA_PRICE =11.50; constdouble PHILODENDRON_PRICE =13.75; constdouble HOYA_PRICE =10.99; constint MAX_POTS =20; constdouble POINTS_PER_DOLLAR =1.0/0.75; char plantType; int quantity; double totalAmount =0.0; int totalPoints =0; int availablePots =0; double plantPrice =0.0; cout << "Welcome to Tom's Plant Shop!" << endl; cout << "******************************" << endl; cout << "Enter your full name: "; string fullName; getline(cin, fullName); output: compiling code... Welcome to Tom's Plant Shop!----------------------------------------Enter your full name: ______ Available plants:M - Monstera ($ 11.5)P - Philodendron ($13.75)H - Hoya ($10.99)Q - Quit shoppingEnter the plant type (M/P/H/Q): ________ Part 1 Tom has recently started a plant-selling business, and he offers three different types of plants, namely Monstera,…arrow_forward// EmployeeBonus2.cpp - This program calculates an employee's yearly bonus. #include <iostream> #include <string> using namespace std; int main() { // Declare and initialize variables. string employeeFirstName; string employeeLastName; double employeeSalary; int employeeRating; double employeeBonus; const double BONUS_1 = .25; const double BONUS_2 = .15; const double BONUS_3 = .10; const double NO_BONUS = 0.00; const int RATING_1 = 1; const int RATING_2 = 2; const int RATING_3 = 3; // This is the work done in the housekeeping() function // Get user input cout << "Enter employee's first name: "; cin >> employeeFirstName; cout << "Enter employee's last name: "; cin >> employeeLastName; cout << "Enter employee's yearly salary: "; cin >> employeeSalary; cout << "Enter employee's performance rating: "; cin >> employeeRating; // This is…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
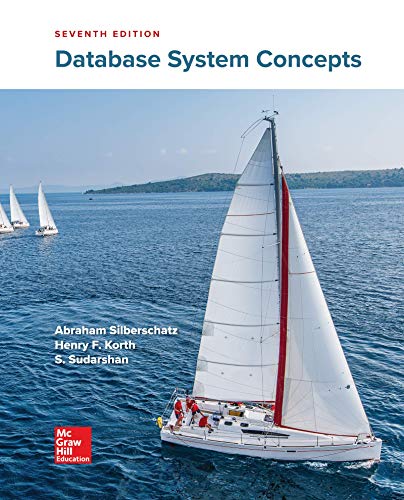
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
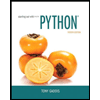
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
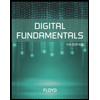
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
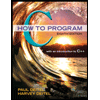
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
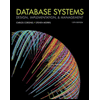
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
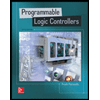
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education