#include #include #include using namespace std; struct Teletype { string name; string phonenum; Teletype *nextaddr; }; void populate(Teletype *); void displayrecord(Teletype *); //void insertrecord(Teletype *); // create //void removerecord(Teletype *); //create //void modifyrecord(Teletype *); // create //int find(TeleType *, string); // Extra Credit create bool check(); int main() { int location = 0; int count = 0; char answery_n; Teletype *list, *current; list = new Teletype; current = list; cout << "Please "; do { count++; populate(current); if (check() == false) { cout << " Not storage available" << endl; } else { current->nextaddr = new Teletype; current = current->nextaddr; cout << "Would you like to input more data? y/n ?: "; cin >> answery_n; cout << endl; cin.get(); if (answery_n != 'y') { current->nextaddr = NULL; break; } } } while (answery_n == 'y'); cout << "The linked list records: " << endl; displayrecord(list); cout << "There are " << count << " records in the data file. " << endl; while (1) { cout << "Select from the menu " << endl; cout << "1. Insert new structure in the linked list" << endl; cout << "2. Modify an existing structure in the linked list" << endl; cout << "3. Delete an existing structure from the list" << endl; cout << "4. Find an existing structure from the list" << endl; cout << "5. Exit from the program" << endl; cin >> answery_n; //**************************************** // Continue … } system("pause"); return 0; } //******************************* void populate(Teletype *record) { cout << "Enter a Name: " << endl; getline(cin, record->name); cout << "Enter Phone Number: " << endl; getline(cin, record->phonenum); return; } //******************************* void displayrecord(Teletype *contents) { while (contents != NULL) { cout << endl << setiosflags(ios::left) << setw(29) << contents->name << setw(19) << contents->phonenum; contents = contents->nextaddr; } cout << endl; return; } //******************************* bool check() { if (new Teletype == NULL) { return false; } else { return true; } }
#include <iostream> #include <iomanip> #include <string> using namespace std;
struct Teletype { string name; string phonenum; Teletype *nextaddr;
};
void populate(Teletype *); void displayrecord(Teletype *); //void insertrecord(Teletype *); // create //void removerecord(Teletype *); //create //void modifyrecord(Teletype *); // create //int find(TeleType *, string); // Extra Credit create
bool check();
int main() { int location = 0; int count = 0; char answery_n;
Teletype *list, *current;
list = new Teletype; current = list;
cout << "Please ";
do { count++; populate(current); if (check() == false) { cout << " Not storage available" << endl; } else { current->nextaddr = new Teletype; current = current->nextaddr; cout << "Would you like to input more data? y/n ?: "; cin >> answery_n; cout << endl; cin.get();
if (answery_n != 'y') { current->nextaddr = NULL; break; } } } while (answery_n == 'y');
cout << "The linked list records: " << endl; displayrecord(list);
cout << "There are " << count << " records in the data file. " << endl;
while (1) { cout << "Select from the menu " << endl; cout << "1. Insert new structure in the linked list" << endl; cout << "2. Modify an existing structure in the linked list" << endl; cout << "3. Delete an existing structure from the list" << endl; cout << "4. Find an existing structure from the list" << endl; cout << "5. Exit from the program" << endl; cin >> answery_n;
//**************************************** // Continue …
}
system("pause"); return 0;
}
//******************************* void populate(Teletype *record) { cout << "Enter a Name: " << endl; getline(cin, record->name); cout << "Enter Phone Number: " << endl; getline(cin, record->phonenum); return; }
//******************************* void displayrecord(Teletype *contents) { while (contents != NULL) { cout << endl << setiosflags(ios::left) << setw(29) << contents->name << setw(19) << contents->phonenum; contents = contents->nextaddr; } cout << endl; return; } //******************************* bool check() { if (new Teletype == NULL) { return false; } else { return true; }
} |

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

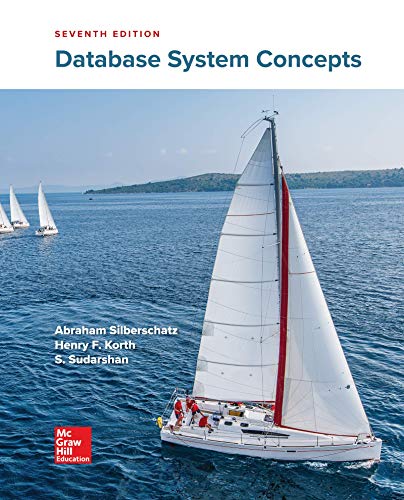
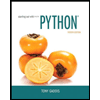
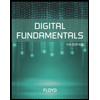
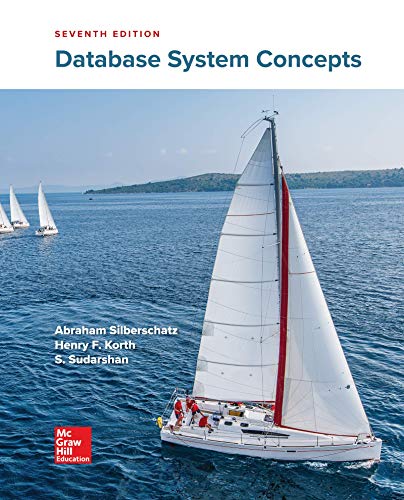
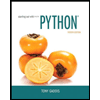
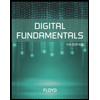
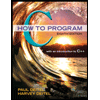
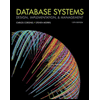
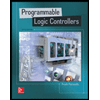