def calculate_growth_cycle(plant_name): if(plant_name == "strawberry"): print("### The life cycle of a strawberry ###\nA seed takes 110 days to reach maturity.") elif(plant_name == "cucumber"): print("### The life cycle of a cucumber ###\nA seed takes 76 days to reach maturity.") elif(plant_name == "potato"): print("### The life cycle of a potato ###\nA seed takes 120 days to reach maturity.") else: print('Your plant is available, please try "strawberry", "cucumber" or "potato"') Plants Growth Cycle Learning Objectives In this lab, you will practice: Defining a function to match the given specifications Calling the function in your program Using if statements (can combine them with dictionaries) Instructions For every plant, there is a growth cycle. The number of days that it takes starting from being a seed and ending in being a fruit is what is called the growth cycle. Write a function that takes a plant's name as an argument and returns its growth cycle (in days). In your program: Input from the user the name of a plant Check if the input is either "strawberry", "cucumber" or "potato", if Yes: 2.1 Call calculate_growth_cycle 2.2. In function calculate_growth_cycle, check over the plant's name: 2.2.1 If strawberry, print "### The life cycle of a strawberry ###" and return 110 2.2.2 If cucumber, print "### The life cycle of a cucumber ###" and return 76 2.2.3 If potato, print "### The life cycle a potato ###" and return 120 2.3 With the growth cycle number returned, your program should print "A seed takes days to reach maturity." If not, your program should print "Your plant is available, please try "strawberry", "cucumber" or "potato" Example Input potato Output ### The life cycle of a potato ### A seed takes 120 days to reach maturity. Input Mango Output Your plant is available, please try "strawberry", "cucumber" or "potato" References Strawberry growth cycle Cucumber growth cycle Potato growth cycle This is my code below: if __name__ == "__main__": plant_name = input() plant_name = plant_name.lower() calculate_growth_cycle(plant_name) The lab say: Function works incorrectly, check the parametrs and return values
def calculate_growth_cycle(plant_name):
if(plant_name == "strawberry"):
print("### The life cycle of a strawberry ###\nA seed takes 110 days to reach maturity.")
elif(plant_name == "cucumber"):
print("### The life cycle of a cucumber ###\nA seed takes 76 days to reach maturity.")
elif(plant_name == "potato"):
print("### The life cycle of a potato ###\nA seed takes 120 days to reach maturity.")
else:
print('Your plant is available, please try "strawberry", "cucumber" or "potato"')
Plants Growth Cycle
Learning Objectives
In this lab, you will practice:
- Defining a function to match the given specifications
- Calling the function in your program
- Using if statements (can combine them with dictionaries)
Instructions
For every plant, there is a growth cycle. The number of days that it takes starting from being a seed and ending in being a fruit is what is called the growth cycle. Write a function that takes a plant's name as an argument and returns its growth cycle (in days).
In your program:
-
Input from the user the name of a plant
-
Check if the input is either "strawberry", "cucumber" or "potato", if Yes:
2.1 Call calculate_growth_cycle
2.2. In function calculate_growth_cycle, check over the plant's name:
2.2.1 If strawberry, print "### The life cycle of a strawberry ###" and return 110
2.2.2 If cucumber, print "### The life cycle of a cucumber ###" and return 76
2.2.3 If potato, print "### The life cycle a potato ###" and return 120
2.3 With the growth cycle number returned, your program should print "A seed takes <growth_number> days to reach maturity."
If not, your program should print "Your plant is available, please try "strawberry", "cucumber" or "potato"
Example
Input
potatoOutput
### The life cycle of a potato ### A seed takes 120 days to reach maturity.Input
MangoOutput
Your plant is available, please try "strawberry", "cucumber" or "potato"References
Strawberry growth cycle
Cucumber growth cycle
Potato growth cycle
This is my code below:
if __name__ == "__main__":
plant_name = input()
plant_name = plant_name.lower()
calculate_growth_cycle(plant_name)
The lab say:
Function works incorrectly, check the parametrs and return values


As given, I need to write a Python program according to the given requirements.
What's wrong with your code is -
You have not returned any value in your function calculate_growth_cycle(). The number of days should be returned in the function. As you haven't done that part, you are getting an error.
I have provided the complete code with detailed comments and output screenshots in the following steps.
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 4 images

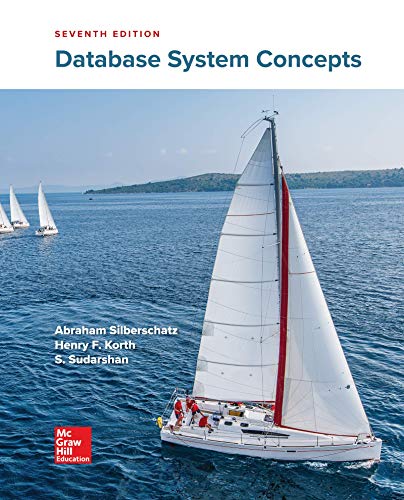
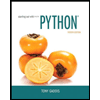
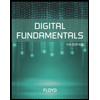
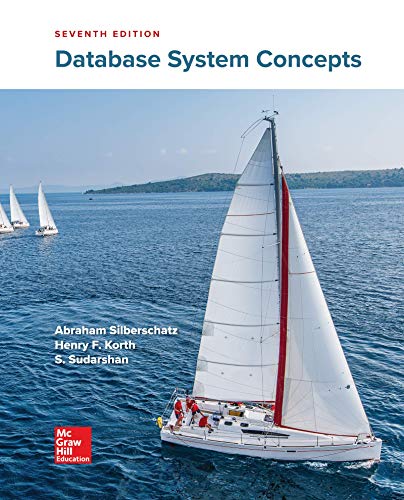
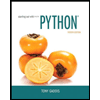
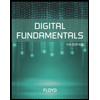
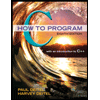
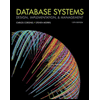
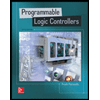