var adventurersName = ["George", " Tim", " Sarah", " Mike", " Edward"]; var adventurersKilled = 3; var survivors; var leader = "Captain Thomas King"; var numberOfAdventurers = adventurersName.length; survivors = numberOfAdventurers - adventurersKilled; console.log("Welcome to The God Among Us\n"); console.log("A group of adventurers began their search for the mystical god said to live among us. In charge of the squad was " + leader + " who was famous for his past exploits. Along the way, the group of comrades were attacked by the god's loyal followers. The adventurers fought with bravado and strength under the tutelage of "+ leader + " the followers were defeated but they still suffered great losses. After a headcount of the remaining squad, "+ adventurersKilled +" were found to be dead which left only " + survivors + " remaining survivors.\n"); console.log("Current Statistics :\n"); console.log("Total Adventurers = " + numberOfAdventurers); console.log("Total Killed = " + adventurersKilled); console.log("Total Survived = " + survivors); const prompt = require('prompt-sync')(); var user_life = 3; var correct_answer = "2" var userIsCorrect; var options = ["\nOption 1 Enter the village hut?","Option 2 Eat the turkey leg?","Option 3 Sit on the stool?","Option 4 Talk with the shadowy figure?\n"]; var leader = prompt("Enter Leader Name: ") while (user_life>0) { user_life = game(leader,options); if (user_life === 0) { var play_again = prompt("Do You Want Play Again (y/n)? "); if (play_again =="y") { user_life = 3 var leader = prompt("Enter Leader Name:") } else { console.log(` Game Ended! Bye!`) break; } } } function game(leader,options) { showOptions(options); const input = prompt("Enter Your choice (Number) "); userIsCorrect = (input == correct_answer); if (userIsCorrect) { console.log("Yay, you picked the right option. You rest a day and come to a new village that looks exactly the same. Which option do you choose?"); }else{ user_life= user_life-1; console.log("One Life is Lost!") } displayStats(user_life,leader); return user_life; } function showOptions(option) { console.log(option[0]); console.log(option[1]); console.log(option[2]); console.log(option[3]); } function displayStats(user_life,name) { console.log(` Current Statistics: Leader Name: ${name} Lives Remaining: ${user_life}`) console.log("Adventurers in your party = " + survivors); } What needs to be changed in the Notepad++ code for the correct answers to be 1, 2 and 3 instead of just 2? Also, How do I get "Game ended, Bye to show up without closing the cmd?
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
var adventurersName = ["George", " Tim", " Sarah", " Mike", " Edward"];
var adventurersKilled = 3;
var survivors;
var leader = "Captain Thomas King";
var numberOfAdventurers = adventurersName.length;
survivors = numberOfAdventurers - adventurersKilled;
console.log("Welcome to The God Among Us\n");
console.log("A group of adventurers began their search for the mystical god said to live among us. In charge of the squad was " + leader + " who was famous for his past exploits. Along the way, the group of comrades were attacked by the god's loyal followers. The adventurers fought with bravado and strength under the tutelage of "+ leader + " the followers were defeated but they still suffered great losses. After a headcount of the remaining squad, "+ adventurersKilled +" were found to be dead which left only " + survivors + " remaining survivors.\n");
console.log("Current Statistics :\n");
console.log("Total Adventurers = " + numberOfAdventurers);
console.log("Total Killed = " + adventurersKilled);
console.log("Total Survived = " + survivors);
const prompt = require('prompt-sync')();
var user_life = 3;
var correct_answer = "2"
var userIsCorrect;
var options = ["\nOption 1 Enter the village hut?","Option 2 Eat the turkey leg?","Option 3 Sit on the stool?","Option 4 Talk with the shadowy figure?\n"];
var leader = prompt("Enter Leader Name: ")
while (user_life>0) {
user_life = game(leader,options);
if (user_life === 0) {
var play_again = prompt("Do You Want Play Again (y/n)? ");
if (play_again =="y") {
user_life = 3
var leader = prompt("Enter Leader Name:")
} else {
console.log(`
Game Ended!
Bye!`)
break;
}
}
}
function game(leader,options) {
showOptions(options);
const input = prompt("Enter Your choice (Number) ");
userIsCorrect = (input == correct_answer);
if (userIsCorrect) {
console.log("Yay, you picked the right option. You rest a day and come to a new village that looks exactly the same. Which option do you choose?");
}else{
user_life= user_life-1;
console.log("One Life is Lost!")
}
displayStats(user_life,leader);
return user_life;
}
function showOptions(option) {
console.log(option[0]);
console.log(option[1]);
console.log(option[2]);
console.log(option[3]);
}
function displayStats(user_life,name) {
console.log(`
Current Statistics:
Leader Name: ${name}
Lives Remaining: ${user_life}`)
console.log("Adventurers in your party = " + survivors);
}
What needs to be changed in the Notepad++ code for the correct answers to be 1, 2 and 3 instead of just 2? Also, How do I get "Game ended, Bye to show up without closing the cmd?

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

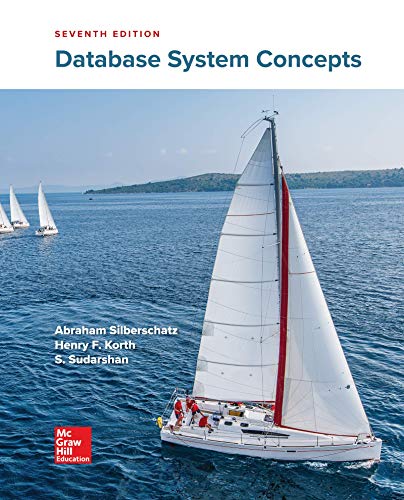
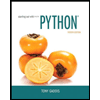
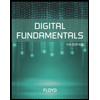
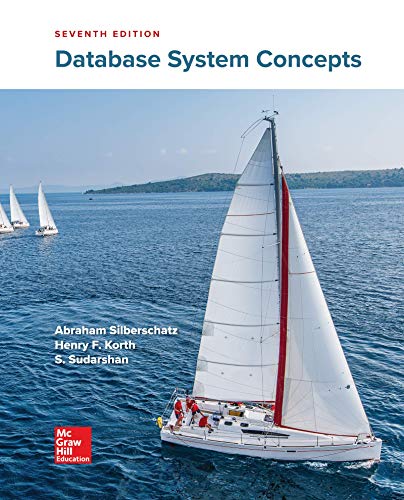
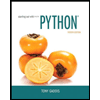
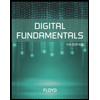
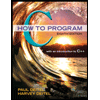
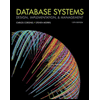
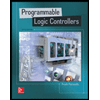