c++ A FastCritter moves twice as fast as a regular critter. When asked to move by n steps, it actually moves by 2 * n steps. Implement a FastCritter class derived from Critter whose move function behaves as described. Complete the following file: fastcritter_Tester.cpp #include using namespace std; #include "critter.h" /** A FastCritter moves twice as fast as a regular critter. */ class FastCritter:public Critter { public: void move(int steps); }; . . . int main() { FastCritter speedy; speedy.move(10); cout << speedy.get_history() << endl; cout << "Expected: [move to 20]" << endl; speedy.move(-1); cout << speedy.get_history() << endl; cout << "Expected: [move to 20, move to 18]" << endl; return 0; } Use the following file: critter.h #ifndef CRITTER_H #define CRITTER_H #include #include using namespace std; /** A simulated critter. */ class Critter { public: /** Constructs a critter at position 0 with blank history. */ Critter(); /** Gets the history of this critter. @return the history */ string get_history() const; /** Adds to the history of this critter. @param event the event to add to the history */ void add_history(string event); /** Gets the position of this critter. @return the position */ int get_position() const; /** Moves this critter. @param steps the number of steps by which to move. */ void move(int steps); /** The action of this critter in one step of the simulation. */ void act(); private: int position; vector history; }; #endif
c++
A FastCritter moves twice as fast as a regular critter. When asked to move by n steps, it actually moves by 2 * n steps. Implement a FastCritter class derived from Critter whose move function behaves as described.
Complete the following file:
fastcritter_Tester.cpp
#include <iostream>
using namespace std;
#include "critter.h"
/**
A FastCritter moves twice as fast as a regular critter.
*/
class FastCritter:public Critter
{
public:
void move(int steps);
};
. . .
int main()
{
FastCritter speedy;
speedy.move(10);
cout << speedy.get_history() << endl;
cout << "Expected: [move to 20]" << endl;
speedy.move(-1);
cout << speedy.get_history() << endl;
cout << "Expected: [move to 20, move to 18]" << endl;
return 0;
}
Use the following file:
critter.h
#ifndef CRITTER_H
#define CRITTER_H
#include <string>
#include <vector>
using namespace std;
/** A simulated critter. */
class Critter { public:
/** Constructs a critter at position 0 with blank history. */
Critter();
/** Gets the history of this critter. @return the history */
string get_history() const;
/** Adds to the history of this critter. @param event the event to add to the history */
void add_history(string event);
/** Gets the position of this critter. @return the position */
int get_position() const;
/** Moves this critter. @param steps the number of steps by which to move. */
void move(int steps);
/** The action of this critter in one step of the simulation. */
void act();
private:
int position;
vector<string> history; };
#endif

Step by step
Solved in 2 steps with 1 images

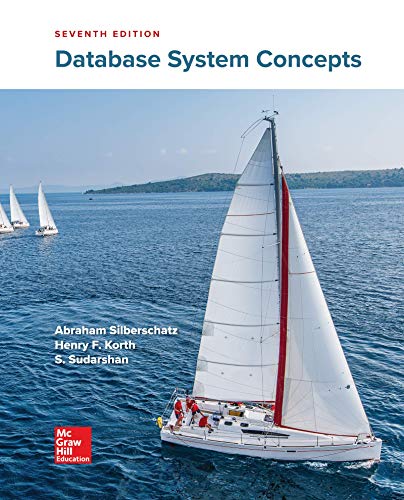
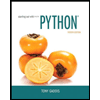
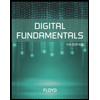
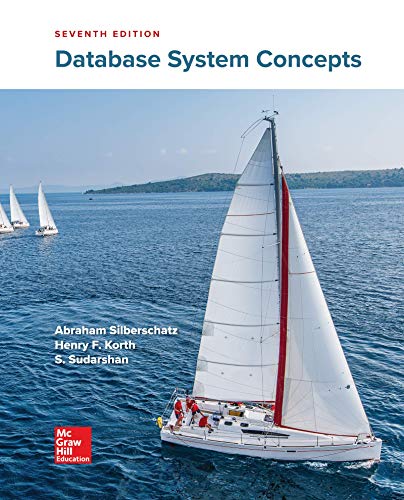
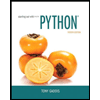
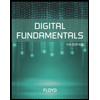
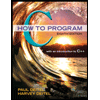
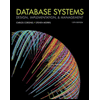
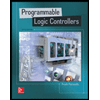