What is Class?
Object Oriented Programming System (OOPs) is a programming model built on the perception of “objects” that contains data and methods. The major purpose of Object Oriented Programming is to increase the maintainability and flexibility of programs. Object Oriented Programming gets together data and its behavior (methods) in a single location (object) which makes it easier to understand.
The building block for the OOPs approach is a Class (CLS). It is commonly said “class is similar to a blueprint for an object.” It can be termed as a user determined data type, which embraces its data members and member functions. These can be retrieved and used by creating an instance of the same class in a system. Data members are the data variables and the member functions are the program functions. They are used to operate these variables as per the given time duration. The data members and member functions outline the possessions and behavior of the objects in the current class system.
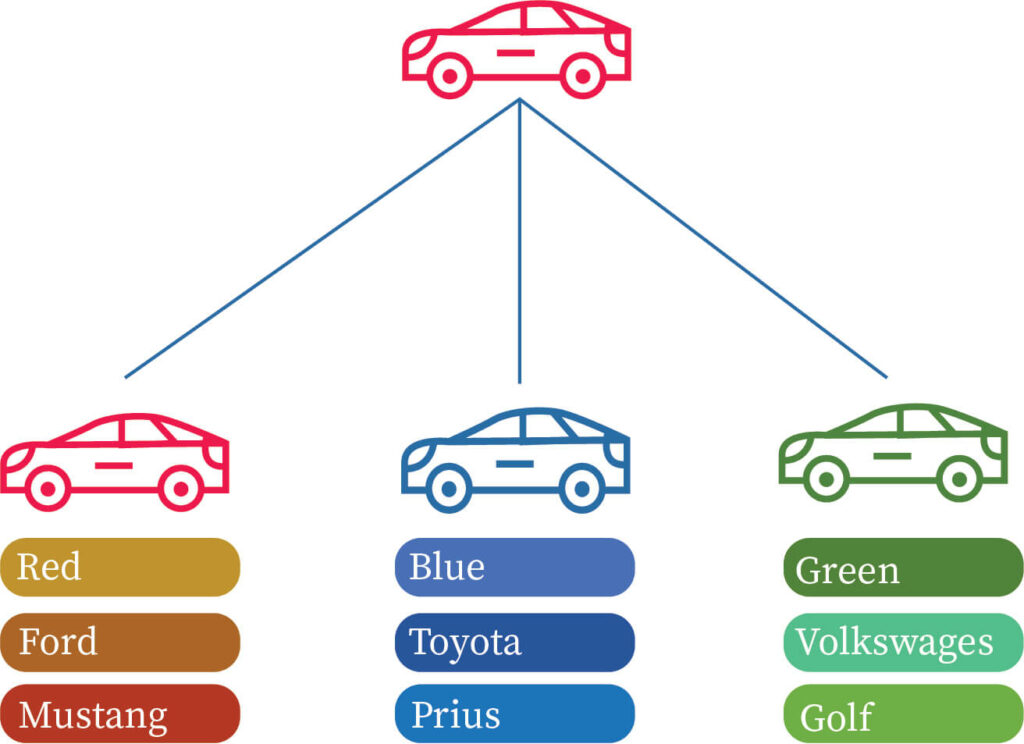
For example: Consider the CLS of bikes. There are a variety of bikes with diverse names and brand yet all of them share a few common properties like 2 wheels, Mileage, RPM, brake type, cost, features etc. So here, Bike is the CLS and wheels, Mileage, RPM, brake type, cost, and features are their properties. Here wheels, RPM are the data members and applying brakes etc. are the member functions. In order to understand class one must thoroughly understand the concept of object.
Object in Class
Object is a classifiable object with a few characteristics and behavior. It is commonly said that “Object is instance of Class”. When a CLS is well-defined there is no memory allocation but when it is instantiated (i.e. an object is created) memory is allocated. When a program is implemented the objects interact by sending and receiving messages to one another.
Every Object holds data and required code to employ the data. at a fixed time duration. These Objects can communicate lacking to know details of subordinate code, it is sufficient to identify the kind of message accepted and kind of reply returned by objects.
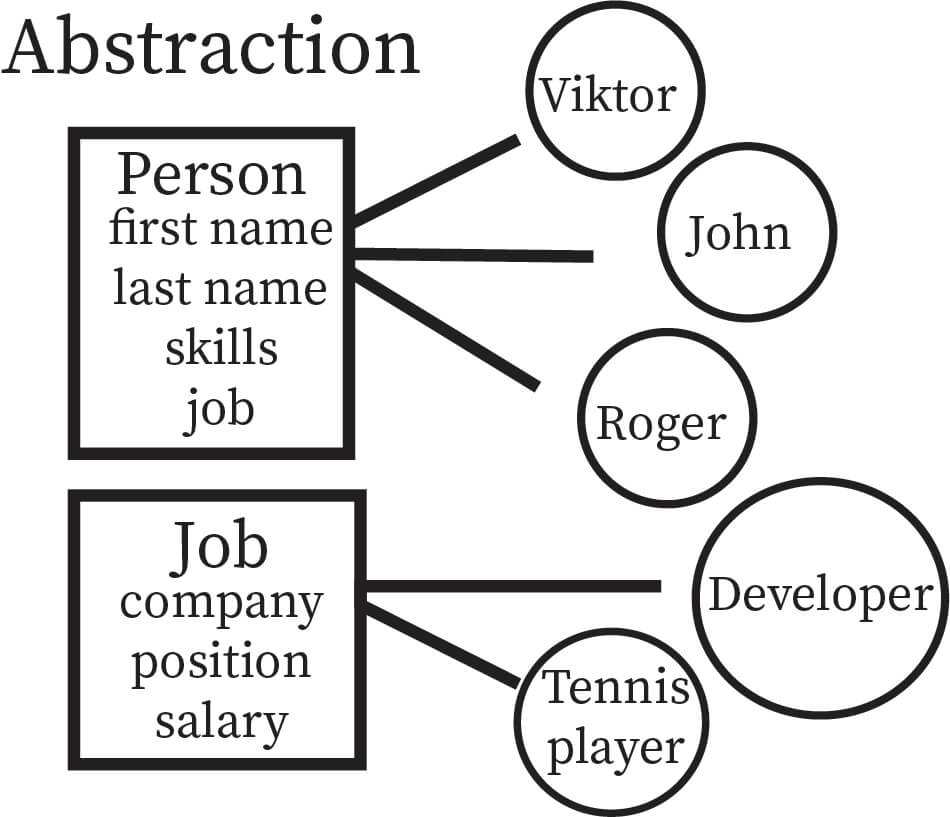
Objects mainly have three characteristics:
- Abstraction: It is a process of displaying only applicable data and masking unnecessary details of an object from the user.
- Encapsulation: It is a process of wrapping object state (fields) and behavior (methods) together.
- Message passing: is a process wherein multiple objects of the same application interact with each other. This is done by a method Invocation process.
Difference between Object and Class
Class | Object |
Create or instantiate an object | Instance of a CLS |
Logical entity | Physical entity |
No memory allocated on creation | Appropriate memory allocation on creation |
Declared once | Multiple objects can be created in a class |
Created using “class” keyword | Created using “new” keyword |
CLS is a type | Object is a variable |
Defining Class & Declaring Objects
- Define a Class: The CLS is defined using a keyword “class” tailed by name of CLS. The form of CLS is defined within curly brackets and completed by a semicolon. For better understanding way of structure is given below:
Syntax:
CLS ClassName
{
Access Specifier;
Data Member;
Member Function ( ) { }
};
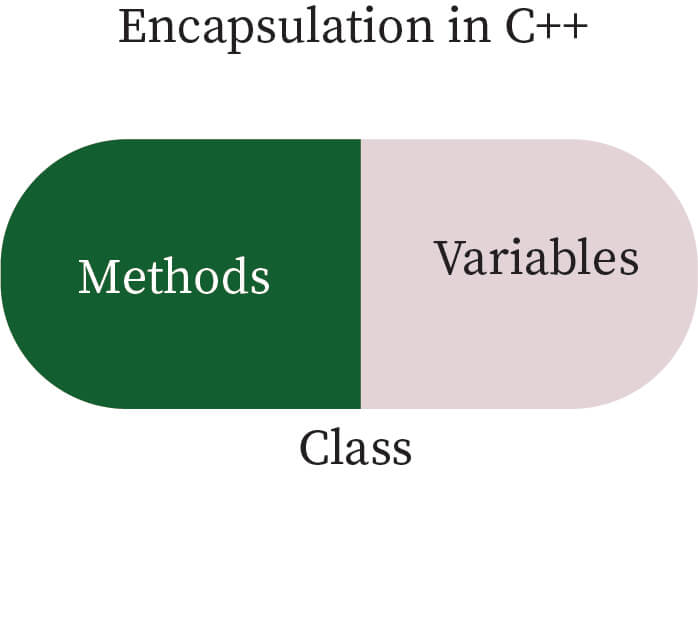
Here is an explanation for above example:
- CLS is the keyword to define a class definition.
- Class Name is the name of the CLS defined by the user.
- Access Specifier can be private, public or protected basically defines the access permissions with respect to an object.
- Data members are the variable in use.
- Member functions are the methods that help access the data members.
- Remember that Class Name must always end with a semi colon.
Example:
Define CLS for a student
CLS student
{
char name[20];
int age;
int USN[10];
char address[30];
char course[10];
public:
void getdetails(){}
};
- Declaring an Object: While a CLS is definite, the description for the object is definite; no memory storage is allocated. In order to use the data and access functions defined in the CLS, an object needs to be created and it is same as for base class.
Syntax:
ClassName ObjectName;
Example:
int main()
{
student s1; // s1 is an object
}
Data Members and Member Functions
- Data member: Data member and its function can be accessed using the dot (.) operator with the object. The access controls are given by the access modifiers. OOPs supports three types of access modifiers namely:
- Public: CLS members are accessible by other classes or functions or current class in the program.
- Private: CLS members declared with private are accessible by member function inside the same current class only.
- Protected: CLS members declared with protected are accessible by member function inside the same CLS and its sub CLSs.
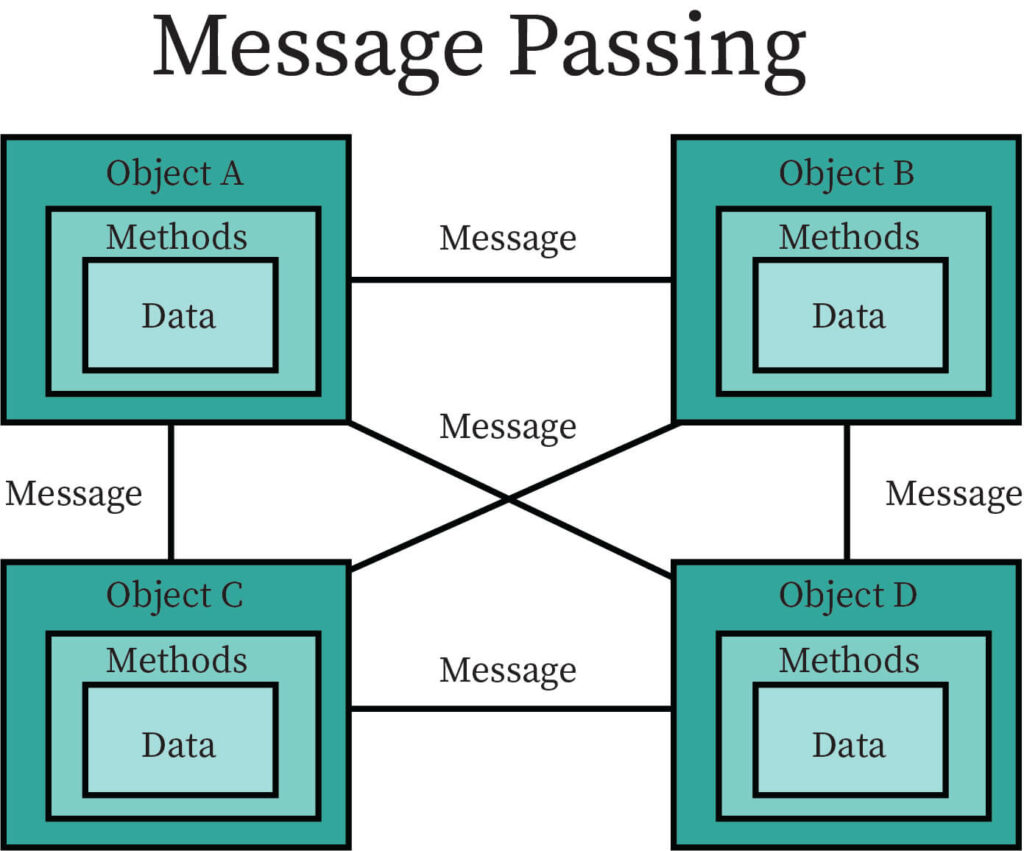
Snippet to demonstrate accessing of data members:
CLS student
{
public:
string stu_name;
void printname()
{
cout << "Student name is: " << stu_name;
}
};
int main ( )
{
student s1;
s1.stu_name = "Laksh"; // accessing member function
s1.printname ( );
return 0;
}
Output:
Student name is: Laksh
Member functions: Member function can be defined in 2 ways:
- Outside CLS definition
- Inside CLS definition
While defining a member function outside the CLS it is to be accessed using scope resolution “::“ operator along with CLS and function name. If no name is given to the CLS it’s become anonymous class.
Constructors and Destructors
- Constructors: These are special CLS members that are called by the complier every time an object is instantiated. Constructors have the comparable name as that of a CLS and don’t have a return type. They can be definite inside a CLS or outside.
Constructors (CNST) are broadly classified as:
- Default CNST
- Parameterized CNST
- Copy CNST
Example:
CLS Student
{
public:
int id;
//Default CNST
Student()
{
cout << "Default CNST called" << endl;
id=-1;
}
//Parameterized CNST
Student(int x)
{
cout << "Parametrized CNST called" << endl;
id=x;
}
};
int main() {
// s1 will call Default CNST
Student s1;
cout << "Student id is: " <<s1.id << endl;
// s1 will call Parameterized CNST
Student s2(21);
cout << "Student id is: " <<s2.id << endl;
return 0;
}
Output:
Default Constructor called
Student id is: -1
Parameterized Constructor called
Student id is: 21
- Destructors: Destructor is special member function which is called-by the compiler when the scope of the object ends. It is a method which is provoked just before the memory of the object is release. Destructors of a CLS hold the same name only that the destructor has a “~” symbol before its name. Also an exception can never be thrown by a destructor.
Syntax:
CLS Student
{
public:
int id;
Example:
//Definition for Destructor
~Student()
{
cout << "Destructor called for id: " << id <<endl;
}
};
int main()
{
Student s1;
s1.id=9;
int i = 0;
while ( i < 4 )
{
Student s2;
s2.id=i;
i++;
} // Scope for s2 ends here
return 0;
} // Scope for s1 ends here
Output:
Destructor called for id: 0
Destructor called for id: 1
Destructor called for id: 2
Destructor called for id: 3
Destructor called for id: 8
Context and Applications
This topic is significant in the professional exams for both undergraduate and graduate courses, especially for
- B.Sc. Computer science
- B.C.A
- M.C.A
- B.Tech Computer science
- M.Tech Computer Science
Want more help with your computer science homework?
*Response times may vary by subject and question complexity. Median response time is 34 minutes for paid subscribers and may be longer for promotional offers.
Search. Solve. Succeed!
Study smarter access to millions of step-by step textbook solutions, our Q&A library, and AI powered Math Solver. Plus, you get 30 questions to ask an expert each month.
Class Homework Questions from Fellow Students
Browse our recently answered Class homework questions.
Search. Solve. Succeed!
Study smarter access to millions of step-by step textbook solutions, our Q&A library, and AI powered Math Solver. Plus, you get 30 questions to ask an expert each month.