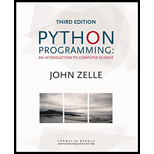
Python Programming: An Introduction to Computer Science, 3rd Ed.
3rd Edition
ISBN: 9781590282755
Author: John Zelle
Publisher: Franklin, Beedle & Associates
expand_more
expand_more
format_list_bulleted
Question
Chapter 9, Problem 9PE
Program Plan Intro
Blackjack game
Program Plan:
- Import the header file.
- Define the main method.
- Call the “printIntro ()” method.
- Get the input from the user.
- Iterate “i” till it reaches 13.
- Increment the “i” value
- Call the “simNHands ()” method and store it in a variable.
- Call the “printSummary ()” method.
- Define the “printIntro ()” method.
- Display the messages.
- Define the “simNHands ()” method.
- Display the starting card number.
- Set the values.
- Iterate “i” until it reaches “n” value
- Check the result of “simOneHand ()” is false
- Increment the “busts” value
- Check the result of “simOneHand ()” is false
- Return the “busts” value.
- Definition of “simOneHand ()” method
- Set the value
- Check “x” value is equal to 11 or 12 or 13
- Set “x” as 10
- Call the simOneCard ()” method and store it in a “y” variable.
- Calculate “hand” value
- Check the “hand” value is less than 17
- Check “hand” is less than or equal to 10
- Check the result of “hasAce (x)” is true
- Call the “simOneCard()” method
- Check the result of “hasAce ()” is false.
- Calculate the “hand” value
- Check the condition
- Calculate the “hand” value
- Otherwise, calculate the “hand” value.
-
- Check the condition
- Calculate the “hand” value.
- Otherwise, break the loop.
- Check “hand” value is less than 17 and greater than 10
- Call the method
- Calculate the “hand” value.
- Check the condition
-
- Check the result of “hasAce (x)” is true
- Check “hand” is less than or equal to 10
- Check the “hand” is greater than 21
- Return the result as false
- Otherwise, return true.
- Define the “simOneCard ()” method
- Call the method with the arguments
- Check “x” is equal to 11 or 12 or 13
- Set the “x” as 10
- Return “x”
- Otherwise, return “x” value
- Define the “hasAce ()” method
- Check “x” is equal to 1
- Return true
- Otherwise, return false.
- Check “x” is equal to 1
- Define the “printSummary ()” method
- Declare the array
- Display the output.
- Call the main method.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Of the five primary components of an information system (hardware, software, data, people, process), which do you think is the most important to the success of a business organization?
Part A - Define each primary component of the information system.
Part B - Include your perspective on why your selection is most important.
Part C - Provide an example from your personal experience to support your answer.
Management Information Systems
Q2/find the transfer function C/R for the system shown in the figure
Re
ད
Chapter 9 Solutions
Python Programming: An Introduction to Computer Science, 3rd Ed.
Ch. 9 - Prob. 1TFCh. 9 - Prob. 2TFCh. 9 - Prob. 3TFCh. 9 - Prob. 4TFCh. 9 - Prob. 5TFCh. 9 - Prob. 6TFCh. 9 - Prob. 7TFCh. 9 - Prob. 8TFCh. 9 - Prob. 9TFCh. 9 - Prob. 10TF
Ch. 9 - Prob. 1MCCh. 9 - Prob. 2MCCh. 9 - Prob. 3MCCh. 9 - Prob. 4MCCh. 9 - Prob. 5MCCh. 9 - Prob. 6MCCh. 9 - Prob. 7MCCh. 9 - Prob. 8MCCh. 9 - Prob. 9MCCh. 9 - Prob. 10MCCh. 9 - Prob. 2DCh. 9 - Prob. 3DCh. 9 - Prob. 1PECh. 9 - Prob. 2PECh. 9 - Prob. 3PECh. 9 - Prob. 4PECh. 9 - Prob. 5PECh. 9 - Prob. 6PECh. 9 - Prob. 7PECh. 9 - Prob. 8PECh. 9 - Prob. 9PECh. 9 - Prob. 10PECh. 9 - Prob. 11PECh. 9 - Prob. 12PECh. 9 - Prob. 13PECh. 9 - Prob. 14PE
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Please original work select a topic related to architectures or infrastructures (Data Lakehouse Architecture). Discussing how you would implement your chosen topic in a data warehouse project Please cite in text references and add weblinksarrow_forwardPlease original work What topic would be related to architectures or infrastructures. How you would implement your chosen topic in a data warehouse project. Please cite in text references and add weblinksarrow_forwardWhat is cloud computing and why do we use it? Give one of your friends with your answer.arrow_forward
- What are triggers and how do you invoke them on demand? Give one reference with your answer.arrow_forwardDiscuss with appropriate examples the types of relationships in a database. Give one reference with your answer.arrow_forwardDetermine the velocity error constant (k,) for the system shown. + R(s)- K G(s) where: K=1.6 A(s+B) G(s) = as²+bs C(s) where: A 14, B =3, a =6. and b =10arrow_forward
- • Solve the problem (pls refer to the inserted image)arrow_forwardWrite .php file that saves car booking and displays feedback. There are 2 buttons, which are <Book it> <Select a date>. <Select a date> button gets an input from the user, start date and an end date. Book it button can be pressed only if the start date and ending date are chosen by the user. If successful, it books cars for specific dates, with bookings saved. Booking should be in the .json file which contains all the bookings, and have the following information: Start Date. End Date. User Email. Car ID. If the car is already booked for the selected period, a failure message should be displayed, along with a button to return to the homepage. In the booking.json file, if the Car ID and start date and end date matches, it fails Use AJAX: Save bookings and display feedback without page refresh, using a custom modal (not alert).arrow_forwardWrite .php file with the html that saves car booking and displays feedback. Booking should be in the .json file which contains all the bookings, and have the following information: Start Date. End Date. User Email. Car ID. There are 2 buttons, which are <Book it> <Select a date> Book it button can be pressed only if the start date and ending date are chosen by the user. If successful, book cars for specific dates, with bookings saved. If the car is already booked for the selected period, a failure message should be displayed, along with a button to return to the homepage. Use AJAX: Save bookings and display feedback without page refresh, using a custom modal (not alert). And then add an additional feature that only free dates are selectable (e.g., calendar view).arrow_forward
- • Solve the problem (pls refer to the inserted image) and create line graph.arrow_forwardwho started the world wide webarrow_forwardQuestion No 1: (Topic: Systems for collaboration and social business The information systems function in business) How does Porter's competitive forces model help companies develop competitive strategies using information systems? • List and describe four competitive strategies enabled by information systems that firms can pursue. • Describe how information systems can support each of these competitive strategies and give examples.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrOperations Research : Applications and AlgorithmsComputer ScienceISBN:9780534380588Author:Wayne L. WinstonPublisher:Brooks Cole
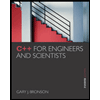
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
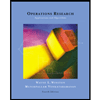
Operations Research : Applications and Algorithms
Computer Science
ISBN:9780534380588
Author:Wayne L. Winston
Publisher:Brooks Cole
Java random numbers; Author: Bro code;https://www.youtube.com/watch?v=VMZLPl16P5c;License: Standard YouTube License, CC-BY