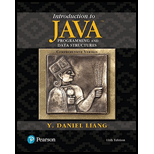
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
11th Edition
ISBN: 9780134670942
Author: Y. Daniel Liang
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Textbook Question
Chapter 8.8, Problem 8.8.3CP
Show the output of the following code:
int[][][] array= {{{1, 2}, {3, 4}}, {{5, 6},{7, 8}}};
System.out.println(array[0] [0](0]);
System.out.println(array [1] [1] [1]);
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
int [] arrayInt={3,54,90,22,32};
To print the last element in the arrayInt you will write:
a.
System.out.println(arrayInt[5]);
b.
System.out.println(arrayInt[0]);
c.
System.out.println(arrayInt[length]);
d.
System.out.println(arrayInt[4]);
Print the elements of the array ‘a’ using the mentioned notations:
int a[]={1,2,3,4,5};
int *p;
p = a;
for (int i = 0; i< 5; i++)
{
//Subscript notation with name of array
//Subscript notation with pointer 'p'
//Offset notation using array name
//Offset notation using pointer 'p'
}
void showArray ( int a[ ], int size ); // shows the array in the format "int a [ ] = { 3, 7, 4, ... ,5, 6, 3}
void showReverse ( int a[ ], int size ); // shows the array in reverse "int a [ ] = { 3, 6, 5, ... , 4, 7, 3 } "
int lowest ( int a[ ], int size ); // finds and returns the lowest value in the array (should be 3)
int highest ( int a[ ], int size ); // finds and returns the highest value in the array (should be 7)
int sumArray ( int a[ ], int size ); // calculates and returns the sum of all values in the array
float averageVal ( int a[ ], int size ); // calculates and returns the average of all values in the array
int count5 ( int a[ ], int size ); // returns how many times the number 5 appears in the array
int firstMinusLast ( int a[ ], int size ); // returns the difference b/w the First and Last Array Element
void showBeforeIndex( int a [ ], int index); // shows all array values before a specific index
void done…
Chapter 8 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Ch. 8.2 - Declare an array reference variable for a...Ch. 8.2 - Prob. 8.2.2CPCh. 8.2 - What is the output of the following code? int[] []...Ch. 8.2 - Which of the following statements are valid? int...Ch. 8.3 - Show the output of the following code: int[][]...Ch. 8.3 - Show the output of the following code: int[][]...Ch. 8.4 - Show the output of the following code: public...Ch. 8.5 - Prob. 8.5.1CPCh. 8.6 - What happens if the input has only one point?Ch. 8.7 - What happens if the code in line 51 in Listing 8.4...
Ch. 8.8 - Declare an array variable for a three-dimensional...Ch. 8.8 - Assume char[][][] x =new char[12][5][2], how many...Ch. 8.8 - Show the output of the following code: int[][][]...Ch. 8 - (Sum elements column by column) Write a method...Ch. 8 - (Sum the major diagonal in a matrix) Write a...Ch. 8 - (Sort students on grades) Rewrite Listing 8.2,...Ch. 8 - (Compute the weekly hours for each employee)...Ch. 8 - (Algebra: add two matrices) Write a method to add...Ch. 8 - (Algebra: multiply two matrices) Write a method to...Ch. 8 - (Points nearest to each other) Listing 8.3 gives a...Ch. 8 - (All closest pairs of points) Revise Listing 8.3,...Ch. 8 - Prob. 8.9PECh. 8 - (Largest row and column) Write a program that...Ch. 8 - (Game: nine heads and tails) Nine coins are placed...Ch. 8 - (Financial application: compute tax) Rewrite...Ch. 8 - (Locate the largest element) Write the following...Ch. 8 - (Explore matrix) Write a program that prompts the...Ch. 8 - (Geometry: same line ?) Programming Exercise 6.39...Ch. 8 - (Sort two-dimensional array) Write a method to...Ch. 8 - (Financial tsunami) Banks lend money to each...Ch. 8 - (Shuffle rows) Write a method that shuffles the...Ch. 8 - (Pattern recognition: four consecutive equal...Ch. 8 - Prob. 8.20PECh. 8 - (Central city) Given a set of cities, the central...Ch. 8 - (Even number of 1s) Write a program that generates...Ch. 8 - (Game: find the flipped cell) Suppose you are...Ch. 8 - (Check Sudoku solution) Listing 8.4 checks whether...Ch. 8 - Prob. 8.25PECh. 8 - (Row sorting) Implement the following method to...Ch. 8 - (Column sorting) Implement the following method to...Ch. 8 - (Strictly identical arrays) The two-dimensional...Ch. 8 - (Identical arrays) The two-dimensional arrays m1...Ch. 8 - (Algebra: solve linear equations) Write a method...Ch. 8 - (Geometry: intersecting point) Write a method that...Ch. 8 - (Geometry: area of a triangle) Write a method that...Ch. 8 - (Geometry: polygon subareas) A convex four-vertex...Ch. 8 - (Geometry: rightmost lowest point) In...Ch. 8 - (Largest block) Given a square matrix with the...Ch. 8 - (Latin square) A Latin square is an n-by-n array...Ch. 8 - (Guess the capitals) Write a program that...
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
State which values of the control variable x are printed by each of the following for statements:
C How to Program (8th Edition)
Write a loop that asks the user Do you want to repeat the program or quit? (R/Q)". The loop should repeat until...
Starting Out with Python (4th Edition)
Could errors have occurred in a byte from Question 1 without your knowing it? Explain your answer.
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
Modify the Product_T table by adding an attribute QtyOnHand that can be used to track the finished goods invent...
Modern Database Management (12th Edition)
Write a program that reads records of type Pet from a file created by the program described in the previous pro...
Java: An Introduction to Problem Solving and Programming (8th Edition)
Assume the following variable definitions: int a = 5, b = 12; double x = 3.4, z = 9.1; What are the values of t...
Starting Out with C++: Early Objects
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Given the following array declaration: int myArray[10]={1,2,3,4,5,6,7,8,9,10}; How would you reference the 3 in the array?arrow_forwardHow do you initialize an array in C? a. int arr[3] = (1,2,3); b. int arr(3) = {1,2,3}; C. int arr(3) = [1,2,3]; Od. int arr[3] = {1,2,3};arrow_forward#include <stdio.h>int counter=0;int size;void deleteNumber(int array[],int index);void insertNumber(int array[],int index,int value);void insertIntelligently(int array[],int number);void insertByvalue(int array[]);void deleteByrange(int array[]);void search(int array[]);void ascendingOrder(int array[]);void descendingOrder(int array[]);void replace(int array[]);void printArray(int array[]);int main() {int array[size], choice, i;printf("Enter size of the array");scanf("%d", &size);for (i = 0; i < size; i++) {printf("Enter the value for Index %d: ", i);scanf("%d", &array[i]);printf("\n");counter++;printf("%d", counter);}printArray(array);printf("\nCounter is: %d \n", counter);printf("\n");while (choice != 9) {printf("\n\nPress '1' to Insert by Index and Value \nPress '2' to Delete \nPress '3' to Insert by Value\nPress '4' to Delete By Range\nPress '5' to replace a value\nPress '6' to search a number\nPress '7' to print in ascending order\nPress '8' to print in descending…arrow_forward
- #include using namespace std; main() { int num array[] = (1, 2, 3, 4, 5, 6, 7, 8, 9, 10}; for (int n-1; n<-5;++n) { cout <« n<< * "; } return 0; Out put : 1 23 4 567 89 10 O Out put : 3 4 56789 10 O Out put : 2 3 45 67 89 10 11 O Out put : 1 2 3 456 Out put : 1 2 345 Not listed Oarrow_forwardint X[10]={2,0,6,11,4,5,9,11,-2,-1); From the code above, what is the value of X[8] ?arrow_forward4. Given an array of ints, return the number of times that two 6's are next to each other in the array.int n[] = {2, 6, 71, 6, 6, 6, 34, 6, 6, 89}; C++arrow_forward
- Assume that the following program segment is executed. What is the last element of testArray2 after the execution of the statement sortArray1(testArray2);? *arrow_forward1. Given the following array declaration: int A [3 ][2 ]={{13, -23}, {3, 80} ,{91,55} }; declare a pointer that points to A[2][0]? Answer:arrow_forwardFor an array declartion of int number [25];, what will be the ending index? 0 1 24 25arrow_forward
- Do not use AI.arrow_forwardDeclaration int a[4][3]; declares an array that contains this many total elements:arrow_forwardJAVA PROGRAM: Monkey Business A local zoo wants to keep track of how many pounds of food each of its three monkeys eats each day during a typical week. Write a program that stores this information in a two-dimensional 3 × 5 array, where each row represents a different monkey and each column represents a different day of the week. The program should first have the user input the data for each monkey, or use constant values rather than asking user for input. Then it should create a report that includes the following information: Display 3X5 array first. Average amount of food eaten per day by the whole family of The least amount of food eaten during the week by any one The greatest amount of food eaten during the week by any one Input Validation: Do not accept negative numbers for pounds of food eaten.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
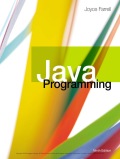
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
9.1: What is an Array? - Processing Tutorial; Author: The Coding Train;https://www.youtube.com/watch?v=NptnmWvkbTw;License: Standard Youtube License