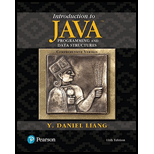
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
11th Edition
ISBN: 9780134670942
Author: Y. Daniel Liang
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 8, Problem 8.9PE
Program Plan Intro
Game: play a tic-tac-toe game
Program Plan:
- Include the necessary package.
- Define the class named “Exercise08_09”.
- Declare the object “obj” for scanner class.
- Define the main method.
- Declare a variable “brd” for grid board.
- Call the method “printBoard()” to print the board on screen.
- Define the “while” loop.
- Call the method “user_move()” which takes board and symbol.
- Call the method “printBoard()”.
- Using “if…else” condition to call the function “check_Won()”.
- If the condition is true, print “X player won” statement on screen and exit the code.
- Otherwise, check the “if” condition and call the function “check_draw()”.
- Print “No winner” on screen and exit the code.
- Otherwise, check the “if” condition and call the function “check_draw()”.
- If the condition is true, print “X player won” statement on screen and exit the code.
- Define the method “user_move()” which takes board and user’s symbol.
- Declare the Boolean variable “done” and assign “false” on screen.
- Define “do…while” loop.
- Inside the loop, prompt the value of variables “row” and “column” from user.
- Using “if…else” condition, initialize the user’s symbol into board and assign “true” to variable “done”.
- Otherwise, print the statement.
- Define the method “printBoard()” which takes the board as input.
- Using “for” loop, print the board with symbols on screen.
- Define the method “check_won()” which takes character symbol and board as arguments.
- Using “for” loop and “if” condition, check the values for row, column, major diagonal, and sub diagonal grid values.
- Define the method “check_draw()” which takes board as input.
- Using “for” loops, check the grid values.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
1. Astronomy Board Game
In an astronomy board game, N planets in an imaginary universe do not follow the normal law of
gravitation. All the planets are positioned in a row.
The planetary system can be in a stable state only if the sum of the mass of all planets at even
positions is equal to the sum of the mass of planets at the odd positions.
Initially, the system is not stable, but a player can destroy one planet to make it stable. Find the
planet that should be destroyed to make the system stable. If no such planet exists, then return
-1. If there are multiple such planets, then destroy the planet with the smallest index and return
the index of the destroyed planet.
Example
Let N=5 and planets = [2,4,6,3,4]. Destroying the fourth planet of mass 3 will result in planets=
[2,4,6,4], and here, the sum of odd positioned planets is (2+6)=8, and the sum of even positioned
planets is (4+4)=8, and both are equal now. Hence, we destroy the fourth planet.
Function Description
Complete the…
Lucky Pairs
Richie and Raechal are participating in a game called "Lucky pairs" at the Annual Game Fair in their Company. As per the rules of the contest, two members form a team and Richie initially has the number A and Raechal has the number B.There are a total of N turns in the game, and Richie and Raechal alternatively take turns. In each turn, the player's number is multiplied by 2. Richie has the first turn. Suppose after the entire N turns, Richie’s number has become C, and Raechal’s number has become D, the final score of the team will be the sum of the scores (C+D) of both the players after N turns. Write a program to facilitate the quiz organizers to find the final scores of the team. Input and Output Format:The only line of input contains 3 integers A, B, and N.Output a single line that contains the integer that gives the final score of the team which will be the sum of the scores of both the players after N turns.Refer sample input and output for formatting specifications.…
The card game “War” is played by the following rules (you may have learned different rules. Use these):
- Each player gets half of the deck of cards (in our case, a set of random integers with value 1-13)
- The game itself is played in a series of “rounds”. In each round: o Both players draw a card, putting it into the “reward pile” (and removing it from their hand). o If one player’s card is higher than another, they gain all cards in the reward pile (and add it to their hand) o If there is a tie, both players add an additional card to the reward pile (which is not compared
- I call this a “penalty card” in my sample run below), and then we begin a new round with a larger reward pile. The next round’s winner will win the entire reward pile (though it is possible to have multiple consecutive ties, leading to a much larger reward pile)
- The game ends when a player needs to draw a card and cannot. That player loses.
Your task is to implement this game with the player’s hands and the…
Chapter 8 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Ch. 8.2 - Declare an array reference variable for a...Ch. 8.2 - Prob. 8.2.2CPCh. 8.2 - What is the output of the following code? int[] []...Ch. 8.2 - Which of the following statements are valid? int...Ch. 8.3 - Show the output of the following code: int[][]...Ch. 8.3 - Show the output of the following code: int[][]...Ch. 8.4 - Show the output of the following code: public...Ch. 8.5 - Prob. 8.5.1CPCh. 8.6 - What happens if the input has only one point?Ch. 8.7 - What happens if the code in line 51 in Listing 8.4...
Ch. 8.8 - Declare an array variable for a three-dimensional...Ch. 8.8 - Assume char[][][] x =new char[12][5][2], how many...Ch. 8.8 - Show the output of the following code: int[][][]...Ch. 8 - (Sum elements column by column) Write a method...Ch. 8 - (Sum the major diagonal in a matrix) Write a...Ch. 8 - (Sort students on grades) Rewrite Listing 8.2,...Ch. 8 - (Compute the weekly hours for each employee)...Ch. 8 - (Algebra: add two matrices) Write a method to add...Ch. 8 - (Algebra: multiply two matrices) Write a method to...Ch. 8 - (Points nearest to each other) Listing 8.3 gives a...Ch. 8 - (All closest pairs of points) Revise Listing 8.3,...Ch. 8 - Prob. 8.9PECh. 8 - (Largest row and column) Write a program that...Ch. 8 - (Game: nine heads and tails) Nine coins are placed...Ch. 8 - (Financial application: compute tax) Rewrite...Ch. 8 - (Locate the largest element) Write the following...Ch. 8 - (Explore matrix) Write a program that prompts the...Ch. 8 - (Geometry: same line ?) Programming Exercise 6.39...Ch. 8 - (Sort two-dimensional array) Write a method to...Ch. 8 - (Financial tsunami) Banks lend money to each...Ch. 8 - (Shuffle rows) Write a method that shuffles the...Ch. 8 - (Pattern recognition: four consecutive equal...Ch. 8 - Prob. 8.20PECh. 8 - (Central city) Given a set of cities, the central...Ch. 8 - (Even number of 1s) Write a program that generates...Ch. 8 - (Game: find the flipped cell) Suppose you are...Ch. 8 - (Check Sudoku solution) Listing 8.4 checks whether...Ch. 8 - Prob. 8.25PECh. 8 - (Row sorting) Implement the following method to...Ch. 8 - (Column sorting) Implement the following method to...Ch. 8 - (Strictly identical arrays) The two-dimensional...Ch. 8 - (Identical arrays) The two-dimensional arrays m1...Ch. 8 - (Algebra: solve linear equations) Write a method...Ch. 8 - (Geometry: intersecting point) Write a method that...Ch. 8 - (Geometry: area of a triangle) Write a method that...Ch. 8 - (Geometry: polygon subareas) A convex four-vertex...Ch. 8 - (Geometry: rightmost lowest point) In...Ch. 8 - (Largest block) Given a square matrix with the...Ch. 8 - (Latin square) A Latin square is an n-by-n array...Ch. 8 - (Guess the capitals) Write a program that...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- def winning_card(cards, trump=None): Playing cards are again represented as tuples of (rank,suit) as in the cardproblems.pylecture example program. In trick taking games such as whist or bridge, four players each play one card from their hand to the trick, committing to their play in clockwise order starting from the player who plays first into the trick. The winner of the trick is determined by the following rules:1. If one or more cards of the trump suit have been played to the trick, the trick is won by the highest ranking trump card, regardless of the other cards played.2. If no trump cards have been played to the trick, the trick is won by the highest card of the suit of the first card played to the trick. Cards of any other suits, regardless of their rank, are powerless to win that trick.3. Ace is the highest card in each suit.Note that the order in which the cards are played to the trick greatly affects the outcome of that trick, since the first card played in the trick…arrow_forwardDescription: Raghu and Sayan both like to eat (a lot) but since they are also looking after their health, they can only eat a limited amount of calories per day. So when Kuldeep invites them to a party, both Raghu and Sayan decide to play a game. The game is simple, both Raghu and Sayan will eat the dishes served at the party till they are full, and the one who eats maximum number of distinct dishes is the winner. However, both of them can only eat a dishes if they can finish it completely i.e. if Raghu can eat only 50 kCal in a day and has already eaten dishes worth 40 kCal, then he can't eat a dish with calorie value greater than 10 kCal. Given that all the dishes served at the party are infinite in number, (Kuldeep doesn't want any of his friends to miss on any dish) represented by their calorie value(in kCal) and the amount of kCal Raghu and Sayan can eat in a day, your job is to find out who'll win, in case of a tie print "Tie" (quotes for clarity). Input: First line contains…arrow_forwardPLEASE CODE IN PYTHON The Penny Pitch game is popular in amusement parks. Pennies are tossed onto a board that has certain areas marked with different prizes. For example: The prizes available on this board are puzzle, game, ball, poster, and doll. At the end of the game, if all of the squares that say BALL are covered by a penny, the player gets the ball. This is also true for the other prizes. The board is made up of 25 squares (5 x 5). Each prize appears on three randomly chosen squares so that 15 squares contain prizes.In Python, create a PennyPitch application that displays a Penny Pitch board (use [ and ] to indicate squares) with prizes randomly placed and then simulates ten pennies being randomly pitched onto the board. After the pennies have been pitched, the application should display a message indicating which prizes have been won, if any.arrow_forward
- Python tic tac toe. Tic tac toe is a very popular game. Only two players can play at a time. Game Rules Traditionally the first player plays with "X". So you can decide who wants to go with "X" and who wants to go with "O". Only one player can play at a time. If any of the players have filled a square then the other player and the same player cannot override that square. There are only two conditions that may match will be a draw or may win. The player that succeeds in placing three respective marks (X or O) in a horizontal, vertical, or diagonal row wins the game. Winning condition Whoever places three respective marks (X or O) horizontally, vertically, or diagonally will be the winner. Submit your code and screenshots of your code in action. Hints : Have a function that draws the board Have a function that checks position if empty or not Have a function that checks player or won or not expected output:arrow_forwardFaceUp card game In this assignment we will implement a made-up card game we'll call FaceUp. When the game starts, you deal five cards face down. Your goal is to achieve as high a score as possible. Your score only includes cards that are face up. Red cards (hearts and diamonds) award positive points, while black cards (clubs and spades) award negative points. Cards 2-10 have points worth their face value. Cards Jack, Queen, and King have value 10, and Ace is 11. The game is played by flipping over cards, either from face-down to face-up or from face-up to face-down. As you play, you are told your total score (ie, total of the face-up cards) and the total score of the cards that are face down. The challenge is that you only get up to a fixed number of flips and then the game is over. Here is an example of the output from playing the game: FACE-DOWN | FACE-DOWN | FACE-DOWN | FACE-DOWN | FACE-DOWN Face up total: 0 Face down total: -5 Number of flips left: 5 Pick a card to flip between 1…arrow_forwardFaceUp card game In this assignment we will implement a made-up card game we'll call FaceUp. When the game starts, you deal five cards face down. Your goal is to achieve as high a score as possible. Your score only includes cards that are face up. Red cards (hearts and diamonds) award positive points, while black cards (clubs and spades) award negative points. Cards 2-10 have points worth their face value. Cards Jack, Queen, and King have value 10, and Ace is 11. The game is played by flipping over cards, either from face-down to face-up or from face-up to face-down. As you play, you are told your total score (ie, total of the face-up cards) and the total score of the cards that are face down. The challenge is that you only get up to a fixed number of flips and then the game is over. Here is an example of the output from playing the game: FACE-DOWN | FACE-DOWN | FACE-DOWN | FACE-DOWN | FACE-DOWN Face up total: 0 Face down total: -5 Number of flips left: 5 Pick a card to flip between 1…arrow_forward
- C Programming Language (Code With C Programming Language) Problem Title : Visible Trees There is a legend about a magical park with N × N trees. The trees are positioned in a square grid with N rows (numbered from 1 to N from north to south) and N columns (numbered from 1 to N from west to east). The height (in metres) of each tree is an integer between 1 and N × N, inclusive. Magically, the height of all trees is unique. Bunga is standing on the northmost point of the park and wants to count the number of visible trees for each Column. Similarly, Lestari is standing on the westmost point of the park and wants to count the number of visible trees for each Row. A tree X is visible if all other trees in front of the tree X are shorter than the tree X. For example, let N = 3 and the height (in metres) of the trees are as follows6 1 87 5 32 9 4 On the first column, Bunga can see two trees, as the tree on the third row is obstructed by the other trees. On the second column, Bunga can see…arrow_forwardJAVA CODE PLEASE THANK YOU Rectangle by CodeChum Admin A rectangle can be formed given two points, the top left point and the bottom right point. Assuming that the top left corner of the console is point (0,0), the bottom right corner of the console is point (MAX, MAX) and given two points (all "x" and "y" coordinates are positive), you should be able to draw the rectangle in the correct location, determine if it is a square or a rectangle, and compute for its area, perimeter and center point. To be able to do this, you should create a class for a point (that has an x-coordinate and a y-coordinate). Also, create another class called Rectangle. The Rectangle should have 2 points, the top left and the bottom right. You should also implement the following methods for the Rectangle: display() - draws the rectangle on the console based on the sample area() - computes and returns the area of a given rectangle perimeter() - computes and returns the perimeter of a given rectangle…arrow_forwardPig is a game that has two players that alternate turns rolling dice. In this case, there will be one human player and one computer player. Each player’s goal is to get 100 points rolled on a normal six-sided die first. Each turn consists of rolling the die repeatedly until you decide to stop or until you roll a 1. For each roll: • If you roll a 2, 3, 4, 5, or 6 –you will add the amount rolled to your score.• If you roll a 1 – your turn ends and you receive zero points for that entire turn (You will keep whatever points you had before your turn started)• If you decide to stop rolling at any point in your turn, your points for that turn are then added to the overall score. The overall score is then safe from future rolls.Use functions to break apart the code into logical portionsarrow_forward
- (PLEASE USE JAVA AND JFRAME GUI) Game rules:The game consists of a two-dimensional field of size m × n (game field). Each element ofthe game field is a button with a number assigned to it.Initialization of the game:• The numbers on the buttons of the game field are set to a random digit between 0and 9• The target value is displayed above the game field• The current sum of the numbers displayed on the buttons of the game field is printedbelow the game field• The number of moves to completion is displayed in the upper right corner above thegame field.Playing:The first move is determined by the player by selecting any button (button A) on thegame field.1. The player is allowed to choose a second button (button B) located in the columnor row of the previously selected button (A).2. Upon selecting the second button (B), the value of button (A) is updated accordingto the following formula: A = (AoperationB)mod10.3. The operation for the basic game will be +.4. Decrement the number of moves…arrow_forwardNim is a two-player game played with several piles of stones. You can use as many piles and as many stones in each pile as you want, but in order to better understand the game, we'll start off with just a few small piles of stones (see figure 1 below). Pile 1 Pile 1 Pile 2 The two players take turns removing stones from the game. On each turn, the player removing stones can only take stones from one pile, but they can remove as many stones from that pile as they want (please note, a player must remove atleast 1 stone from a pile during his/her turn). If they want, they can even remove the entire pile from the game! The winner is the player who removes the final stone (avoid taking the last stone - see figure 2 below). Pile 2 Pile 3 Pile 3 Let's say its Max (player 1) turn to play. Then Max can win by simply removing a stone from Pile 2 or Pile 3 Draw a game tree (upto depth level 2) for the given version of the Nim game. Please consider figure 1 as your initial game configuration/state…arrow_forward: You will build a simplified, one-player version of the classic board game Battleship! In this version of the game, there will be a single ship hidden in a random location on a 5 °ø 5 grid. The player will have 4 guesses at most to try to sink the ship. At each guess, the player names an attacking coordinate, that is (“guessrow”, “guess col”). The game ends in two conditions: (1) the player is out of guesses; (2) the player hits the ship. Examples are given in Figure 1. § The 5 X 5 board is shown every time the player inputs a guess entry. § The ship takes only one entry of the board, and it is randomly given before the player’s guesses. § The player inputs guessing entries in the Python console. § Entries that missed the ship are replaced by “X” on the board. § You must use a loop in your code. § Please submit your code and console screenshots to Blackboard. Code containing syntax error will be graded zero. Hints: 1. Create a variable board and set it equal to an empty list and…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
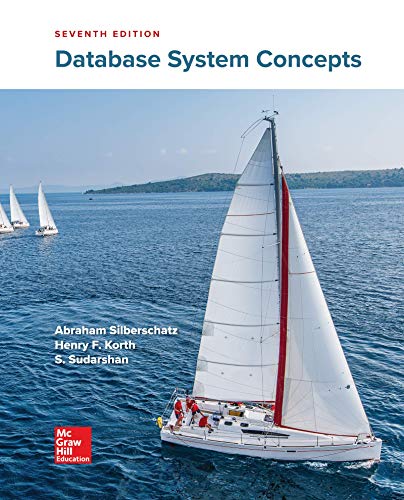
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
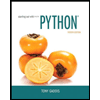
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
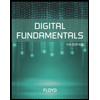
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
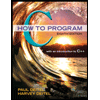
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
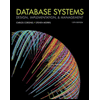
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
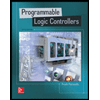
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education