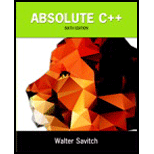
Concept explainers
Define a class for complex numbers. A complex number is a number of the form
where for our purposes, a and b are numbers of type double, and i is a number that represents the quantity
In the interface file, you should define a constant i as follows:
const Complex
This defined constant i will be the same as the i discussed above.

Want to see the full answer?
Check out a sample textbook solution
Chapter 8 Solutions
Absolute C++
Additional Engineering Textbook Solutions
Problem Solving with C++ (10th Edition)
Absolute Java (6th Edition)
Starting Out with C++: Early Objects (9th Edition)
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Objects First with Java: A Practical Introduction Using BlueJ (6th Edition)
Java How To Program (Early Objects)
- Create a Right Triangle class that has two sides. Name your class rightTraingle. Code getter and setters for the base and the height. (Remember class variables are private.) The class should include a two-argument constructor that allows the program to set the base and height. The constructor should verify that all the dimensions are greater than 0. before assigning the values to the private data members. If a side is not greater than zero, set the value to -1. The class also should include two value-returning methods. One value-returning method should calculate the area of a triangle, and the other should calculate the perimeter of a triangle. If either side is -1, these functions return a -1. The formula for calculating the area of a triangle is 1/2 * b*h, where b is the base and h is the height. The formula for calculating the perimeter of a triangle is b+h+sqrt (b*b+h*h). Be sure to include a default constructor that initializes the variables of the base, height to -1. To test…arrow_forwardLanguage is Java Write a Clothing class with the following attributes: color (e.g., "blue", "green", "orange") displayName (e.g., "Doctor Who hoodie", "slacks") price (e.g., 19.99, 7) Include only one constructor. It should have parameters for each of the attributes and set their values. Additionally, include getters and setters for each of the attributes. Add a driver, name it Purchases, and create 2 Clothing objects. Finally, print out some information about both objects (i.e., print the information from some or all of the getters). For example, if you created a Clothing object whose color was blue, whose display name was work trousers, for a price of 27.99, you could use the getters to print something like this:These work trousers are blue and cost $27.99.Don't hardcode the print statement for full credit, you must use the gettersarrow_forward2019 AP® COMPUTER SCIENCE A FREE-RESPONSE QUESTIONS 2. This question involves the implementation of a fitness tracking system that is represented by the StepTracker class. A StepTracker object is created with a parameter that defines the minimum number of steps that must be taken for a day to be considered active. The StepTracker class provides a constructor and the following methods. addDailySteps, which accumulates information about steps, in readings taken once per day activeDays, which returns the number of active days averageSteps, which returns the average number of steps per day, calculated by dividing the total number of steps taken by the number of days tracked The following table contains a sample code execution sequence and the corresponding results. Statements and Expressions Value Returned Comment (blank if no value) StepTracker tr StepTracker(10000); Days with at least 10,000 steps are considered active. Assume that the parameter is positive. new tr.activeDays () ; No…arrow_forward
- In number theory, a value can be categorized as a natural number (a whole number >0, often denoted ℕ), an integer (zero or a positive or negative whole number, including the natural numbers, often denoted ℤ), or a real number (which includes the natural numbers and integers, along with all other positive and negative numbers that are not integers, often denoted ℝ). a) write a definition for a number class that contains: (i) A single field suitable for storing either a natural number, or an integer, or a real number; (ii) Setter and getter methods for manipulating this field; (iii) A constructor that initializes new objects of number to have the value 1 (unity); (iv) a method that determines which kind of number is currently stored (returning 0 if the number is real and an integer and a natural number, 1 if the number is real and integer but not a natural number, and 2 if the number is real but neither an integer nor a natural number) Java code neededarrow_forwardCreate a class BeautyProduct with name, color, brand. Provide Constructors, getters, setters and also write toString method. Now create a class Lipstick which extends the class BeautyProduct as Lipstick is-a Beauty Product it has totalVolume, remainingVolume, price, texture (gloss, matt) as private data members. Provide Constructors, getters, setters and an apply() method whenever this method is called a beauty item is applied and its volume decreases by 10.This should also check that volume must not be zero if it then u have to throw exception. Also write toString method which prints all details like name, color, brand, price, texture and volume. Create another class foundation having totalVolume, remainingVolume, price, texture (liquid, cake) and lastingTime (number of hours foundation remain intact on face and does not crease) as private data members. This also extends the base class i.e. BeautyProduct. Provide Constructors, getters, setters and an apply() method whenever this…arrow_forwardNeed help in Java programming. Write the class definitions for all classes. Be sure to include all properties and two getters and setters for each class. One of the getters and setters should be for a string datatype, and one for either a numeric or Boolean data type. At a minimum the following classes need to be defined: Customer (CustID, LastName, FirstName, Street, City, State, Zip, TaxExempt) Inventory (ItemID, Description, Cost, Selling Price, Quantity On Hand) Order Header (OrderID, CustID, OrderDate, Shipto, Amount Due) Order Detail (OrderID, Line Number, ItemID, Quantity Ordered, Selling Price, Total)arrow_forward
- objective of the project: Implement a class address. An address has a house number street optional apartment number city state postal code. All member variables should be private and the member functions should be public. Implement two constructors: one with an apartment number one without an appartment number. Implement a print function that prints the address with the street on one line and the city, state, and postal code on the next line. Implement a member function comesBefore that tests whether one address comes before another when the addresses are compared by postal code. Returns false if both zipcodes are equal. Use the provided main.cpp to start with. The code creates three instances of the Address class (three objects) to test your class. Each object will utilize a different constructor. You will need to add the class definition and implementation. The comesBefore function assumes one address comes before another based on zip code alone. The test will also return…arrow_forwardwrite a class Point with parametrized constructor. This class have four member variables, a,b,c,d. Write the following member functions a. drawTriangle(int x, int y, int z ) b. drawRectangle(int x, int y, int z, int a). Each function should display the length of lines for each of the shape (triangle, rectangle). For example triangle should calculate length of its three lines by following methods as shown in code. Note: the number of lines depends on the name of shape. void drawTriangle(int x, int y, int z ) { int line 1 = x - y; // convert to positive value if line length is negative int line 2 = y -z; int line 3 = z -x; cout<< The length of each lines is: << //// here display length of each line with proper formatting. } Write similar code for drawRectangle(int x, int y, int z, int a). Wtite main function to call these three functionarrow_forward1) Define a Person class containing two private attributes: last name, first name. Provide this class with a constructor allowing the initialization of its attributes and a method that displays the last name and first name of a person: void display (). 2) Define a Customer class inheriting from the Person class and having a private attribute: numidentity. Provide this class with a constructor allowing you to create a customer from their last name, first name and identity card number and a method: • void display() which displays the last name, first name and ID number of a customer. 3) Define an Owner class inheriting from the Person class and having two private attributes: name of the video club (nomclub), address of the club (adrclub). Provide this class with a constructor allowing to create a landlord from his name, his first name, the name of his video club, the address of his club and a method: • void display() who displays the name, first name, last name and address of his video…arrow_forward
- 1) Define a Person class containing two private attributes: last name, first name. Provide this class with a constructor allowing the initialization of its attributes and a method that displays the last name and first name of a person: void display (). 2) Define a Customer class inheriting from the Person class and having a private attribute: numidentity. Provide this class with a constructor allowing you to create a customer from their last name, first name and identity card number and a method: • void display() which displays the last name, first name and ID number of a customer. 3) Define an Owner class inheriting from the Person class and having two private attributes: name of the video club (nomclub), address of the club (adrclub). Provide this class with a constructor allowing to create a landlord from his name, his first name, the name of his video club, the address of his club and a method: • void display() who displays the name, first name, last name and address of his video…arrow_forwardWrite a class ‘Box’ which has three members height, width and depth; To set values and use these members, write mutator and accessor functions for these members. (Hint: there would be 3 Accessor and 3 Mutator functions).arrow_forwardDefine a new "Exam" class that manages the exam name (string) and its score (integer). For example, an exam can have - "Midterm Exam", 100 - "Final Exam", 50 The class must not provide the default constructor. It must require the exam name and score in order to initialize the Exam object. The class must provide only the following methods (no more and no less): - isPerfect method that returns true if the score is exactly 100 and false otherwise. - isPassing method that returns true if the score is equal or greater than 70 and false otherwise. - toString method must return all the exam information including the result of the exam as a string in the following format: EXAM(<name>) SCORE(<score>) RESULT(Pass/Fail) such asEXAM(Midterm Exam) SCORE(100) RESULT(Pass) EXAM(Final Exam) SCORE(50) RESULT(Fail) "Pass" means the score is greater or equal 70. "Fail" is whenever the score is below 70. - isGreater method that compares with another Exam object and return true if the score…arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
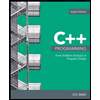