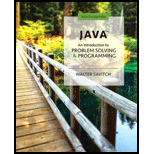
Concept explainers
Create a base class called Vehicle that has the manufacturer's name (type string), number of cylinders in the engine (type int), and owner (type Person given in Listing 8.1). Then create a class called Truck that is derived from Vehicle and has additional properties: the load capacity in tons (type double, since it may contain a fractional part) and towing capacity in tons (type double). Give your classes a reasonable complement of constructors and accessor methods, and an equals method as well. Write a driver program (no pun intended) that tests all your methods.
Deriving a class from Vehicle

Want to see the full answer?
Check out a sample textbook solution
Chapter 8 Solutions
Java: An Introduction to Problem Solving and Programming (8th Edition)
Additional Engineering Textbook Solutions
Starting Out with Java: Early Objects (6th Edition)
Concepts Of Programming Languages
Starting Out with C++ from Control Structures to Objects (9th Edition)
Problem Solving with C++ (9th Edition)
Computer Science: An Overview (12th Edition)
- Implement the Seller class as a derived class of Person class. Create the seller.h and seller.cppfiles for this class. The Seller class contains additional data members that hold a seller’s:o average star rating received from the buyers, ando total number of items sold.These data should be accessible by its derived classes. (should these data be private, public, orprotected?) The following methods are included in the seller class:• A default constructor and a copy constructor• A constructor that receives all of the data for a seller.(for the constructors, make sure to call the base class constructor as appropriate)• Appropriate get and set functions for the new data in this class• print() – overrides the base class print() to print the base class data and print all additionalseller data with appropriate messages. Make sure to call the base class print to print thebase class data.• read() – overrides the base class read() to read the base class data and reads all additionalseller data…arrow_forwardProblem: Create a base class called Vehicle that has the manufacturer’s name (typeString), number of cylinders in the engine (type int), and the owner (type Person). Use thePerson class developed this semester. Create a class automobile that is derived fromVehicle and has additional properties: number of passengers (type int) and weight in tons(type double). Create a class Truck which is derived from Vehicle and has additionalproperties: the load capacity in tons (type double, since it may contain a fractional part)and towing capacity in tons (type double). The classes should have:• Two constructors, a default and an overloaded constructor• All appropriate accessor and mutator methods (getters and setters).• An ‘equals’ method (must conform to the Person example and the Object class ).• A ‘toString’ method• A ‘copy’ constructor• A ‘clone’ method• A ‘finalize’ method Write a driver (client/test) class that tests ALL the methods. Be sure to invoke each of the constructors, and ALL…arrow_forwardDeclare a class named Vehicle as a base class with two data members model (string) and year (int). Then, derive a class called Car as derived class with No (int).arrow_forward
- Person and Customer Classes Write a class named (Person) with data attributes for a person’s name, address, and telephone number. Provide accessors/getters and mutators/setters for each attribute. Write a displayPerson() to print out the attributes of the Person. Next, write a class named (Customer) that is a subclass of the (Person) class. The (Customer) class should have a data attribute for a customer number, and a Boolean data attribute indicating whether the customer wishes to be on a mailing list. Provide accessors/getters and mutators/setters for each attribute. Write a display customer() to print out the attributes of the Customer. Demonstrate an instance of the (Customer) class in a simple program.arrow_forwardCreate a class BeautyProduct with name, color, brand. Provide Constructors, getters, setters and also write toString method. Now create a class Lipstick which extends the class BeautyProduct as Lipstick is-a Beauty Product it has totalVolume, remainingVolume, price, texture (gloss, matt) as private data members. Provide Constructors, getters, setters and an apply() method whenever this method is called a beauty item is applied and its volume decreases by 10.This should also check that volume must not be zero if it then u have to throw exception. Also write toString method which prints all details like name, color, brand, price, texture and volume. Create another class foundation having totalVolume, remainingVolume, price, texture (liquid, cake) and lastingTime (number of hours foundation remain intact on face and does not crease) as private data members. This also extends the base class i.e. BeautyProduct. Provide Constructors, getters, setters and an apply() method whenever this…arrow_forwardCreate a class BeautyProduct with name, color, brand. Provide Constructors, getters, setters and also write toString method. Now create a class Lipstick which extends the class BeautyProduct as Lipstick is-a Beauty Product it has totalVolume, remainingVolume, price, texture (gloss, matt) as private data members. Provide Constructors, getters, setters and an apply() method whenever this method is called a beauty item is applied and its volume decreases by 10.This should also check that volume must not be zero if it then u have to throw exception. Also write toString method which prints all details like name, color, brand, price, texture and volume. Create another class foundation having totalVolume, remainingVolume, price, texture (liquid, cake) and lastingTime (number of hours foundation remain intact on face and does not crease) as private data members. This also extends the base class i.e. BeautyProduct. Provide Constructors, getters, setters and an apply() method whenever this…arrow_forward
- NOTE: READ CAREFULLY Design a class named Person and a subclasses named Employee. Make Teacher a subclass of Employee. A Person has a name, address, and e-mail address. An Employee has an office, salary. A Teacher has office hours and a subject they teach. They also have a tenure status. Define the tenure status as a constant. The tenure status is either Senior or Junior, and is represented as an integer, where Senior is equal to 1, and Junior is equal to 2. Each class should have a Default constructor, and a constructor that accepts all arguments. There should be appropriate calls to the superclass within the constructors. Each class should also have getters and setters for each member variable. Each class should have a toString method. Note: You do NOT need to run this in a test program. Just create the classes as indicated above.arrow_forwardConsider a class "Fan" with two integer data members, "state" (0/1 i.e. on or off) and "speed". 1. Write a parameterized Constructor for this class. 2. Write a member function "changeState( )" which behaves similar to pressing a button i.e. it should change the state of the fan. If it is off (0), calling the function should turn it on (1) and vice versa.. Multi Line Text.arrow_forwardCan a class be a super class and a sub-class at the same time? Give example.arrow_forward
- In the board game Scrabble, each tile contains a letter, which is used to spell words in rows and columns, and a score, which is used to determine the value of words. The point of this exercise is to practice the mechanical part of creating a new class definition: Write a definition for a class named Tile that represents Scrabble tiles. The instance variables should be a character named "letter" and an integer named "value". Write a constructor that takes parameters named letter and value, and initializes the instance variables. Create getters for both of the attributes. (No setters, so that a Tile is immutable.) Implement the .toString() and .equals methods for a Tile. Your completed Tile class should work with this Main program (Links to an external site.) so that it produces sample output like shown at the end of the program. You can Fork the program to make your own version in Replit, where you can add your Tile.java, or you can copy the program to your own Java development…arrow_forwardEvery employee in a firm XYZ has attributes employeeid, name and salary. However the sales employees in firm XYZ has all the attributes of employees and also has an additional attribute named bonus. You are a software developer who has to the implement the relation between employee and sales employee. Write the code for classes employee and employee_sales. Write the constructors for both classes that set the attributes of both classes.arrow_forwardImplement the following using JAVA: a.Design a class named Person and its two subclasses named Student andEmployee. b.Make Faculty and Staff subclasses of Employee. Please note that,● A person has a name, address, phone number, and email address. A student has aclass status (freshman,sophomore, junior, or senior). You can define the status as aconstant.● An employee has office & salary.● A faculty member has office hours and a rank.● A staff member has a title. c. Draw a UML diagram of the system.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
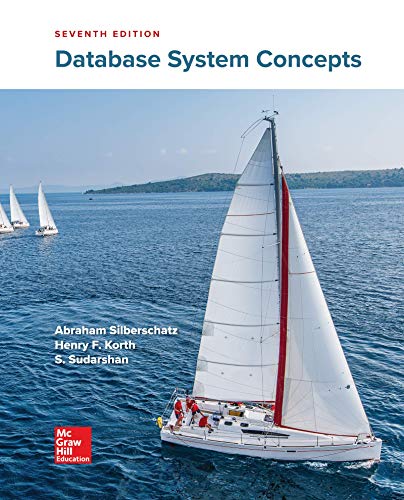
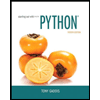
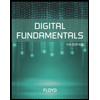
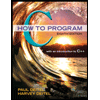
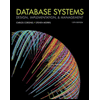
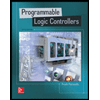