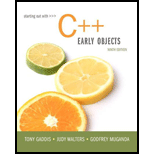
Concept explainers
A)
The Standard Template Library (STL) includes the collection of data types and
- A special data type called vector is offered by STL which is useful for standard arrays.
A vector stores the elements or values in sequence order and the values are stored in continuous memory locations.
- The subscript operator “[]” in the vector helps to read the content of each element.
- If there is a need of vector to be used in the programs, then the following header file must be used:
// Header file for vector
#include<vector>
After including the header file, then it is easy to initialize the vector object.
“#include vector” header file must be included to define the vector in a
Syntax:
Syntax to define a vector is as follows:
//syntax for declaring a vector with size
vector <data_type> name_of_vector(no_of_elements);
In the above line,
- The keyword “vector” represents the vector declaration.
- The “<data_type>” represents the type of data the vector can hold; the data types such as “int”, “float”, “string”, “char”, and “double”.
- The “name_of_vector” represents the name of the vector.
- The “no_of_elements” inside the brackets refers the number of elements that the vector can hold.
B)
Vector:
The Standard Template Library (STL) includes the collection of data types and algorithms which can be used by the programmer in their programs.
- A special data type called vector is offered by STL which is useful for standard arrays.
A vector stores the elements or values in sequence order and the values are stored in continuous memory locations.
- The subscript operator “[]” in the vector helps to read the content of each element.
- If there is a need of vector to be used in the programs, then the following header file must be used:
// Header file for vector
#include<vector>
After including the header file, then it is easy to initialize the vector object.
“#include vector” header file must be included to define the vector in a program.
Syntax:
Syntax to define a vector is as follows:
//syntax for declaring a vector with size
vector <data_type> name_of_vector(no_of_elements);
In the above line,
- The keyword “vector” represents the vector declaration.
- The “<data_type>” represents the type of data the vector can hold; the data types such as “int”, “float”, “string”, “char”, and “double”.
- The “name_of_vector” represents the name of the vector.
- The “no_of_elements” inside the brackets refers the number of elements that the vector can hold.
C)
Vector:
The Standard Template Library (STL) includes the collection of data types and algorithms which can be used by the programmer in their programs.
- A special data type called vector is offered by STL which is useful for standard arrays.
A vector stores the elements or values in sequence order and the values are stored in continuous memory locations.
- The subscript operator “[]” in the vector helps to read the content of each element.
- If there is a need of vector to be used in the programs, then the following header file must be used:
// Header file for vector
#include<vector>
After including the header file, then it is easy to initialize the vector object.
“#include vector” header file must be included to define the vector in a program.
Syntax:
Syntax to define a vector is as follows:
//syntax for declaring a vector with size
vector <data_type> name_of_vector(no_of_elements);
In the above line,
- The keyword “vector” represents the vector declaration.
- The “<data_type>” represents the type of data the vector can hold; the data types such as “int”, “float”, “string”, “char”, and “double”.
- The “name_of_vector” represents the name of the vector.
- The “no_of_elements” inside the brackets refers the number of elements that the vector can hold.
D)
Vector:
The Standard Template Library (STL) includes the collection of data types and algorithms which can be used by the programmer in their programs.
- A special data type called vector is offered by STL which is useful for standard arrays.
A vector stores the elements or values in sequence order and the values are stored in continuous memory locations.
- The subscript operator “[]” in the vector helps to read the content of each element.
- If there is a need of vector to be used in the programs, then the following header file must be used:
// Header file for vector
#include<vector>
After including the header file, then it is easy to initialize the vector object.
“#include vector” header file must be included to define the vector in a program.
Syntax:
Syntax to define a vector is as follows:
//syntax for declaring a vector with size
vector <data_type> name_of_vector(no_of_elements);
In the above line,
- The keyword “vector” represents the vector declaration.
- The “<data_type>” represents the type of data the vector can hold; the data types such as “int”, “float”, “string”, “char”, and “double”.
- The “name_of_vector” represents the name of the vector.
- The “no_of_elements” inside the brackets refers the number of elements that the vector can hold.
E)
Vector:
The Standard Template Library (STL) includes the collection of data types and algorithms which can be used by the programmer in their programs.
- A special data type called vector is offered by STL which is useful for standard arrays.
A vector stores the elements or values in sequence order and the values are stored in continuous memory locations.
- The subscript operator “[]” in the vector helps to read the content of each element.
- If there is a need of vector to be used in the programs, then the following header file must be used:
// Header file for vector
#include<vector>
After including the header file, then it is easy to initialize the vector object.
“#include vector” header file must be included to define the vector in a program.
Syntax:
Syntax to define a vector is as follows:
//syntax for declaring a vector with size
vector <data_type> name_of_vector(no_of_elements);
In the above line,
- The keyword “vector” represents the vector declaration.
- The “<data_type>” represents the type of data the vector can hold; the data types such as “int”, “float”, “string”, “char”, and “double”.
- The “name_of_vector” represents the name of the vector.
- The “no_of_elements” inside the brackets refers the number of elements that the vector can hold.

Want to see the full answer?
Check out a sample textbook solution
Chapter 8 Solutions
Starting Out with C++: Early Objects (9th Edition)
- I need to resolve the following....You are trying to convince your boss that your company needs to invest in a license for MS-Project (project management software from Microsoft) before beginning a systems project. What arguments would you give her?arrow_forwardWhat are the four types of feasibility? what is the issues addressed by each feasibility component.arrow_forwardI would like to get ab example of a situation where Agile Methods might be preferable versus the traditional SDLC? What are the characteristics of this situation that give Agile Methods an advantage?arrow_forward
- What is a functional decomposition diagram? what is a good example of a high level task being broken down into tasks in at least two lower levels (three levels in all).arrow_forwardWhat are the advantages to using a Sytems Analysis and Design model like the SDLC vs. other approaches?arrow_forward3. Problem Description: Define the Circle2D class that contains: Two double data fields named x and y that specify the center of the circle with get methods. • A data field radius with a get method. • A no-arg constructor that creates a default circle with (0, 0) for (x, y) and 1 for radius. • A constructor that creates a circle with the specified x, y, and radius. • A method getArea() that returns the area of the circle. • A method getPerimeter() that returns the perimeter of the circle. • • • A method contains(double x, double y) that returns true if the specified point (x, y) is inside this circle. See Figure (a). A method contains(Circle2D circle) that returns true if the specified circle is inside this circle. See Figure (b). A method overlaps (Circle2D circle) that returns true if the specified circle overlaps with this circle. See the figure below. р O со (a) (b) (c)< Figure (a) A point is inside the circle. (b) A circle is inside another circle. (c) A circle overlaps another…arrow_forward
- 1. Explain in detail with examples each of the following fundamental security design principles: economy of mechanism, fail-safe default, complete mediation, open design, separation of privilege, least privilege, least common mechanism, psychological acceptability, isolation, encapsulation, modularity, layering, and least astonishment.arrow_forwardSecurity in general means the protection of an asset. In the context of computer and network security, explore and explain what assets must be protected within an online university. What the threats are to the security of these assets, and what countermeasures are available to mitigate and protect the organization from such threats. For each of the assets you identify, assign an impact level (low, moderate, or high) for the loss of confidentiality, availability, and integrity. Justify your answers.arrow_forwardPlease include comments and docs comments on the program. The two other classes are Attraction and Entertainment.arrow_forward
- Object-Oriented Programming In this separate files. ent, you'll need to build and run a small Zoo in Lennoxville. All classes must be created in Animal (5) First, start by building a class that describes an Animal at a Zoo. It should have one private instance variable for the name of the animal, and one for its hunger status (fed or hungry). Add methods for setting and getting the hunger satus variable, along with a getter for the name. Consider how these should be named for code clarity. For instance, using a method called hungry () to make the animal hungry could be used as a setter for the hunger field. The same logic could be applied to when it's being fed: public void feed () { this.fed = true; Furthermore, the getter for the fed variable could be named is Fed as it is more descriptive about what it answers when compared to get Fed. Keep this technique in mind for future class designs. Zoo (10) Now we have the animals designed and ready for building a little Zoo! Build a class…arrow_forward1.[30 pts] Answer the following questions: a. [10 pts] Write a Boolean equation in sum-of-products canonical form for the truth table shown below: A B C Y 0 0 0 1 0 0 1 0 0 1 0 0 0 1 1 0 1 0 0 1 1 0 1 0 1 1 0 1 1 1 1 0 a. [10 pts] Minimize the Boolean equation you obtained in (a). b. [10 pts] Implement, using Logisim, the simplified logic circuit. Include an image of the circuit in your report. 2. [20 pts] Student A B will enjoy his picnic on sunny days that have no ants. He will also enjoy his picnic any day he sees a hummingbird, as well as on days where there are ants and ladybugs. a. Write a Boolean equation for his enjoyment (E) in terms of sun (S), ants (A), hummingbirds (H), and ladybugs (L). b. Implement in Logisim, the logic circuit of E function. Use the Circuit Analysis tool in Logisim to view the expression, include an image of the expression generated by Logisim in your report. 3.[20 pts] Find the minimum equivalent circuit for the one shown below (show your work): DAB C…arrow_forwardWhen using functions in python, it allows us tto create procedural abstractioons in our programs. What are 5 major benefits of using a procedural abstraction in python?arrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
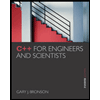
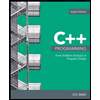
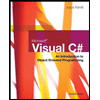
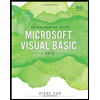
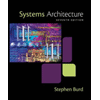