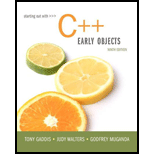
Array:
An array is a data structure which stores multiple values of same types of data; the array values are stored in continuous memory locations.
- Size of an array has been declared inside a square bracket, which is named as size declarator.
- The size declarator must be declared as an integer with a value greater than “0” and the number inside the brackets represents the number of elements that the array can hold.
Syntax:
Syntax to declare an array,
data_type array_name[size_declarator];
Structure array:
- The structure needs an array if the items needs to be grouped together.
- Array is a “derived” data type and it contains similar elements.
- The array index starts with “0”.
- If the size of the array is 4, then the index values are “0”, “1”, “2”, and “3”.
Syntax:
struct_name array_name[size];
Example:
// Structure array declaration
Book name[20];
Explanation:
“Book” is a structure name, “name” is an array name and the value “20” is the “size” of the array.
Array named “forSale” to hold 35 “Car” structure:
The below is the declaration statement of the array named “forSale” to hold “35” cars structure with the first three elements defined:
/* array named forSale to hold 35 car structure with first three values initialized. */
Car forSale[35] = { Car("Ford ", "Taurus ", 2002, 21000),
Car("Honda", "Accord ", 2001, 11000),
Car("Jeep ", "Wrangler", 2004, 24000) };
Explanation:
- “Car” is a structure name, “forSale” is an array name and the value “35” is the size of the array.
- The above array is defined hold first three values that are given.

Want to see the full answer?
Check out a sample textbook solution
Chapter 8 Solutions
Starting Out with C++: Early Objects (9th Edition)
- Write a statement that declares a procedure-level one-dimensional array named intorders. The array should contain 15 elements.arrow_forward(Electrical eng.) Write a program that specifies three one-dimensional arrays named current, resistance, and volts. Each array should be capable of holding 10 elements. Using a for loop, input values for the current and resistance arrays. The entries in the volts array should be the product of the corresponding values in the current and resistance arrays (sovolts[i]=current[i]resistance[i]). After all the data has been entered, display the following output, with the appropriate value under each column heading: CurrentResistance Voltsarrow_forwardWrite a statement that assigns the number of elements in the intOrders array to the intNum variable.arrow_forward
- The variables numberArray1 and numberArray2 reference arrays that have 100 elements each. Write code that copies the values from numberArray1 to numberArray2arrow_forwardAssume that decSales is an array of 20 Decimal values. Write a statement thatresizes the array to 50 elements. If the array has existing values, they should bepreserved.arrow_forwardThe strItems array is declared as follows: Dim strItems(20) As String. The intSub variable keeps track of the array subscripts and is initialized to 0. Which of the following Do clauses will process the loop instructions for each element in the array? a. Do While intSub > 20 b. Do While intSub < 20 c. Do While intSub >= 20 d. Do While intSub <= 20arrow_forward
- Write a piece of code that declares an array variable named data with the elements 7, -1, 13, 24, and 6. Use only one statement to declare and initialize the array.arrow_forwardIn visual basic Write the code that will sequentially search through the array strFirstNames and will print out the word "Found" if the name "Brian" is in the array.arrow_forwardUsing C# in Microsoft Visual Studio Create an application that simulates a game of tic-tac-toe. The application should use a two-dimensional int array to simulate the game board in memory. When the user clicks the New Game button, the application should step through the array, storing a random number in the range of 0 through 1 in each element.The number 0 represents the letter O, and the number 1 represents the letterX. The form should then be updated to display the game board. The application should display a message indicating whether player X won, player Y won, or the game was a tie.arrow_forward
- 3. Declare and initialize an array with the sequence: 300, 200, 100, 400, 500, and 600. Then,whenever the user provides a number, you will return the index of the array element thatstores that number. If the user enters a number that cannot be found in the array, then theprogram should return -1. The program should run in a loop until the user enters the values1234.arrow_forwardTrue or False : Numeric array elements are automatically initialized to –1.arrow_forwardTrue or FalseYou use the == operator to compare two array reference variables and determine whether the arrays are equal.arrow_forward
- Programming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
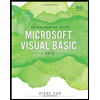
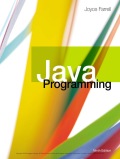
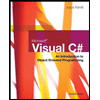
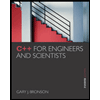
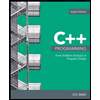